Java in 2020: Mastering the Move to Modular Projects
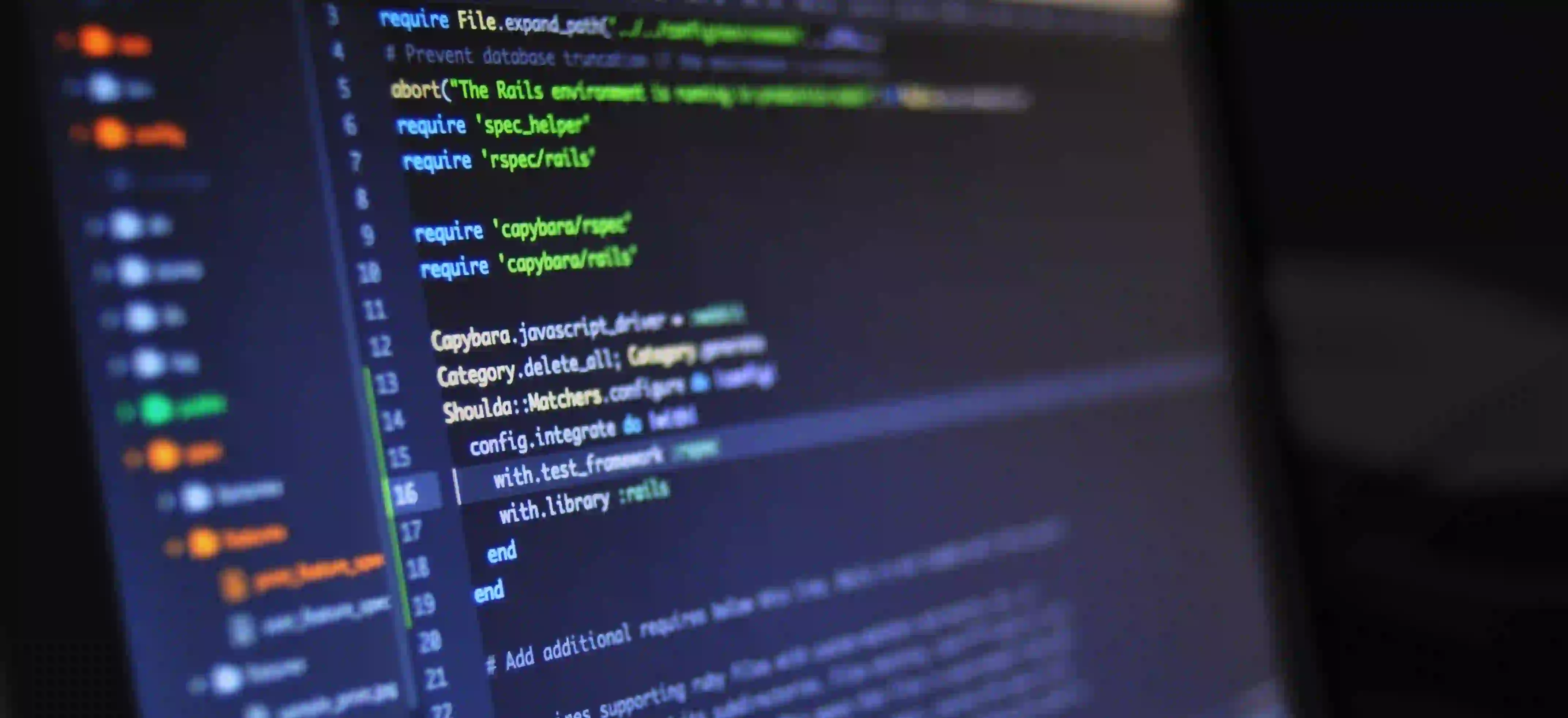
Java in 2020: Mastering the Move to Modular Projects
In the world of Java development, 2020 has brought about significant changes, with modularity being one of the key areas of focus. As a Java developer, it's crucial to stay ahead of the curve and master the move to modular projects. In this blog post, we will delve into the importance of modularity, explore how to create modular projects in Java, and discuss the best practices to optimize your modular Java projects.
The Importance of Modularity in Java
Modularity is all about breaking down a system into smaller, manageable components with well-defined interfaces. In the context of Java, modularity brings a host of benefits including better maintainability, reusability, and encapsulation. With the release of Java 9, the introduction of the Java Platform Module System (JPMS) has made modularity an integral part of Java development.
Creating a Modular Project in Java
Let's dive into creating a modular project in Java. We'll start by creating a simple modular project using Java 9 and then gradually build upon it to understand the key concepts and best practices.
Setting Up the Project Structure
First, let's define a simple modular project structure:
project
ā module-info.java
ā
āāāā com
āāā module1
ā Main.java
ā
āāā module2
Helper.java
In the above structure, the module-info.java
file is key. It defines the module and its dependencies.
Creating module-info.java
module module1 {
exports com.module1;
}
In this module descriptor file, we declare a module named module1
and specify that the package com.module1
is to be exported. This is the essence of modularity ā explicitly defining what parts of the module are accessible to other modules.
Writing the Main Class
package com.module1;
public class Main {
public static void main(String[] args) {
System.out.println("Hello, modular world!");
}
}
Here, we have a simple Main
class within the com.module1
package.
Writing the Helper Class in module2
package com.module2;
public class Helper {
public void help() {
System.out.println("I am here to help!");
}
}
We have created a Helper
class within the com.module2
package to demonstrate inter-module interaction.
Why Modularity is Essential
Modularity is essential for managing the complexity of large-scale applications. It promotes separation of concerns, which in turn enhances maintainability and reusability. By clearly defining module boundaries and dependencies, modularity also facilitates encapsulation, allowing for better control and understanding of the application's architecture.
Best Practices for Modular Java Projects
Now that we have a basic understanding of creating a modular project in Java, let's discuss some best practices to optimize modular Java projects.
Define Clear Module Boundaries
When designing a modular project, it's crucial to define clear and coherent module boundaries. Proper division of modules based on functionality helps in maintaining a clean and organized codebase.
Minimize Module Dependencies
Minimizing module dependencies reduces coupling and simplifies the management of project dependencies. It's important to keep dependencies to a minimum to ensure that modules remain focused and independent.
Encapsulate Internal Implementations
Encapsulation is key to modularity. Hide the internal implementation details of a module by only exposing necessary interfaces. This ensures that changes within a module do not impact other modules.
Use Service Provider Interfaces (SPI)
SPI is a design pattern that allows a module to define services and let other modules implement them. This promotes extensibility and loose coupling, making the application more adaptable to changes.
Leverage Java 9+ Features
With Java 9 and above, several new features have been introduced to support modularity. Utilize features such as jlink
for creating custom runtime images, jdeps
for analyzing dependencies, and jmod
for creating JMOD files.
Closing Remarks
In conclusion, modularity has become an essential aspect of Java development in 2020 and mastering the move to modular projects is crucial for every Java developer. By understanding the importance of modularity, walking through the process of creating a modular project, and adopting best practices, you can optimize your Java projects to be more maintainable, scalable, and adaptable. Stay ahead of the curve and embrace modularity to elevate your Java development skills to the next level.
2020 is the year of modularity in the Java ecosystem. Are you ready to take the leap into modular projects?