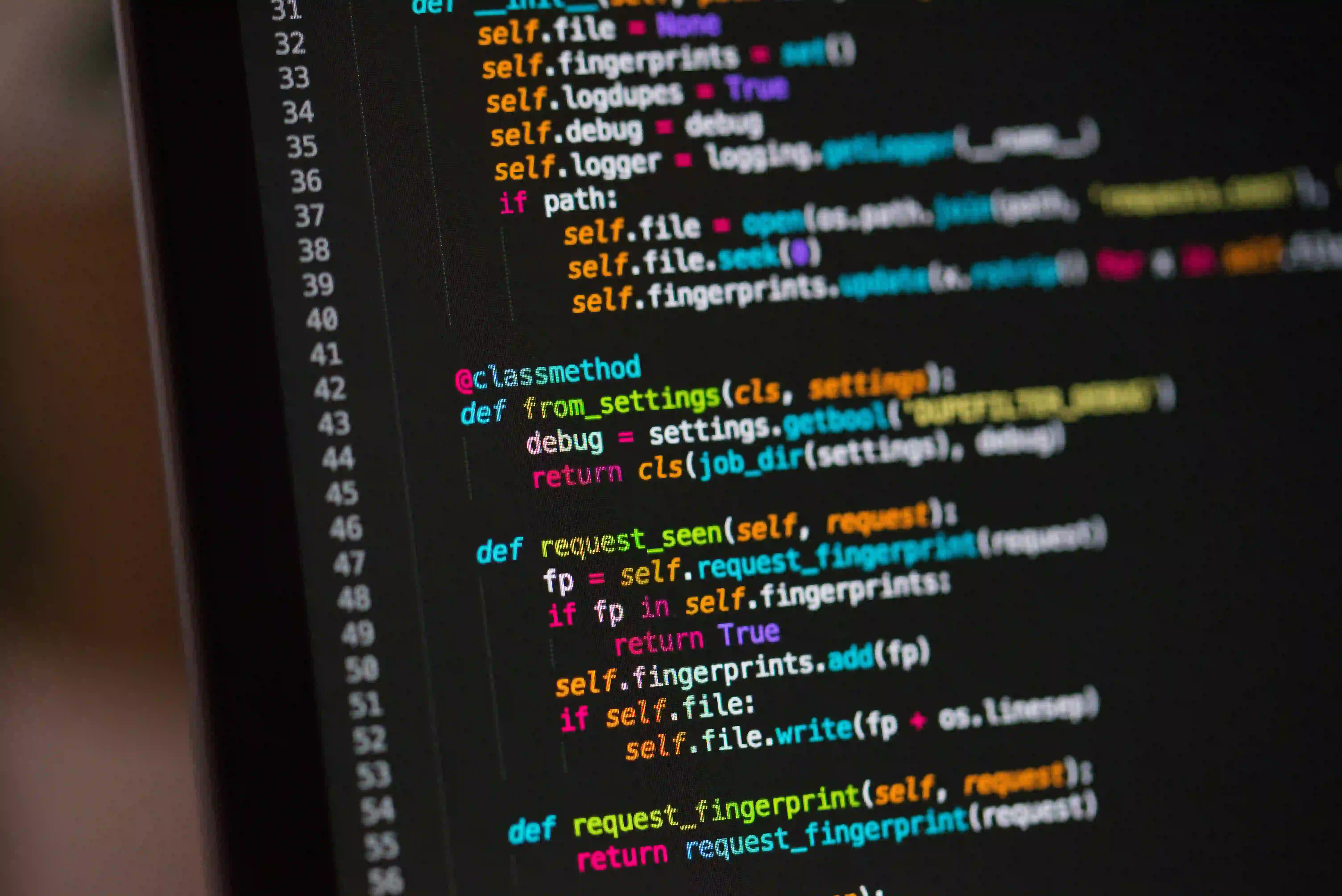
Mastering Unit Testing with Mocking in Spring Security
Unit testing in Java is crucial for ensuring the reliability and maintainability of your codebase. However, when it comes to testing components that involve Spring Security, things can get a bit tricky. In this blog post, we will delve into the intricacies of effectively mocking Spring Security components in unit tests, enabling you to write comprehensive, robust tests for your security-related code.
Understanding the Challenge
Spring Security is a powerful and complex framework that provides various features such as authentication, authorization, and session management. When writing unit tests for classes that interact with Spring Security, we often encounter challenges due to its tight integration with the application context. Additionally, performing actual security operations in unit tests is impractical and can lead to slow and unreliable test suites.
Leveraging Mocking for Unit Testing
To overcome these challenges, we can utilize mocking frameworks such as Mockito to create mock objects that simulate the behavior of Spring Security components. By doing so, we can isolate the code under test and verify its interactions with Spring Security in a controlled environment.
Let's consider a scenario where we have a service class responsible for user authentication. This class interacts with the AuthenticationManager
provided by Spring Security to authenticate user credentials. Our goal is to write unit tests for this service without the need for a complete Spring application context and an actual authentication process.
@Service
public class AuthenticationService {
@Autowired
private AuthenticationManager authenticationManager;
public boolean authenticate(String username, String password) {
// Authenticate user using authenticationManager
// ...
}
}
Creating Mock Objects with Mockito
To begin, we need to create a mock AuthenticationManager
object using Mockito. This can be achieved by annotating a field with @Mock
and initializing the mocks before each test method using MockitoAnnotations.initMocks(this)
.
@RunWith(MockitoJUnitRunner.class)
public class AuthenticationServiceTest {
@Mock
private AuthenticationManager authenticationManager;
@InjectMocks
private AuthenticationService authenticationService;
@Before
public void init() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testAuthenticationSuccess() {
// Define test scenario for successful authentication
// ...
}
@Test
public void testAuthenticationFailure() {
// Define test scenario for failed authentication
// ...
}
}
Implementing Test Scenarios
With the mock AuthenticationManager
in place, we can now define various test scenarios to validate the behavior of our AuthenticationService
. For example, we can simulate successful and failed authentication attempts by configuring the mock object to return appropriate results.
@Test
public void testAuthenticationSuccess() {
// Define test scenario for successful authentication
String username = "user1";
String password = "pass123";
// Configure mock to return successful authentication
when(authenticationManager.authenticate(any(Authentication.class)))
.thenReturn(new UsernamePasswordAuthenticationToken(username, password));
assertTrue(authenticationService.authenticate(username, password));
}
@Test
public void testAuthenticationFailure() {
// Define test scenario for failed authentication
String username = "user2";
String password = "invalidpass";
// Configure mock to throw BadCredentialsException
when(authenticationManager.authenticate(any(Authentication.class)))
.thenThrow(new BadCredentialsException("Invalid credentials"));
assertFalse(authenticationService.authenticate(username, password));
}
Verifying Interactions and Assertions
After defining the test scenarios, we can verify the interactions between the AuthenticationService
and the mock AuthenticationManager
using Mockito's verification and assertion methods. For instance, we can use verify
to ensure that the authenticate
method of the mock was invoked with the expected parameters.
@Test
public void testAuthenticationSuccess() {
// ... (scenario setup)
assertTrue(authenticationService.authenticate(username, password));
// Verify that authenticate method was called with the provided credentials
verify(authenticationManager).authenticate(any(Authentication.class));
}
Enhancing Test Coverage with Integration Testing
While unit testing with mock objects is essential for validating individual components, it's equally important to perform integration testing to assess the behavior of the entire security configuration. Spring Security provides testing support through the @WithMockUser
annotation, enabling you to simulate user authentication in integration tests.
@WebMvcTest(SecurityController.class)
class SecurityControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
@WithMockUser(username = "user1", password = "pass123", roles = "USER")
public void testSecuredEndpointAccess() {
// Test secured endpoint access with authenticated user
// ...
}
@Test
public void testUnsecuredEndpointAccess() {
// Test access to unsecured endpoint without authentication
// ...
}
}
To Wrap Things Up
In conclusion, mastering unit testing with mocking in Spring Security is essential for achieving comprehensive test coverage and ensuring the robustness of security-related components in your applications. By utilizing mocking frameworks like Mockito, you can effectively isolate and test the behavior of classes that interact with Spring Security, while also leveraging integration testing to validate the overall security configuration.
Embracing a test-driven approach and incorporating mocking techniques into your testing strategy will lead to more resilient and maintainable code, ultimately enhancing the security and reliability of your Java applications.
By mastering the art of mocking in Spring Security, you are equipping yourself to write resilient and comprehensive tests, ensuring that your code is reliable and secure.
Remember, solid and complete testing is the foundation of successful and robust software development.
We hope this guide has been illuminating, but if you need further guidance, you can always refer to the official Spring Security documentation or reach out to the Java community.