Mastering Repeatable Reads in Hibernate Applications
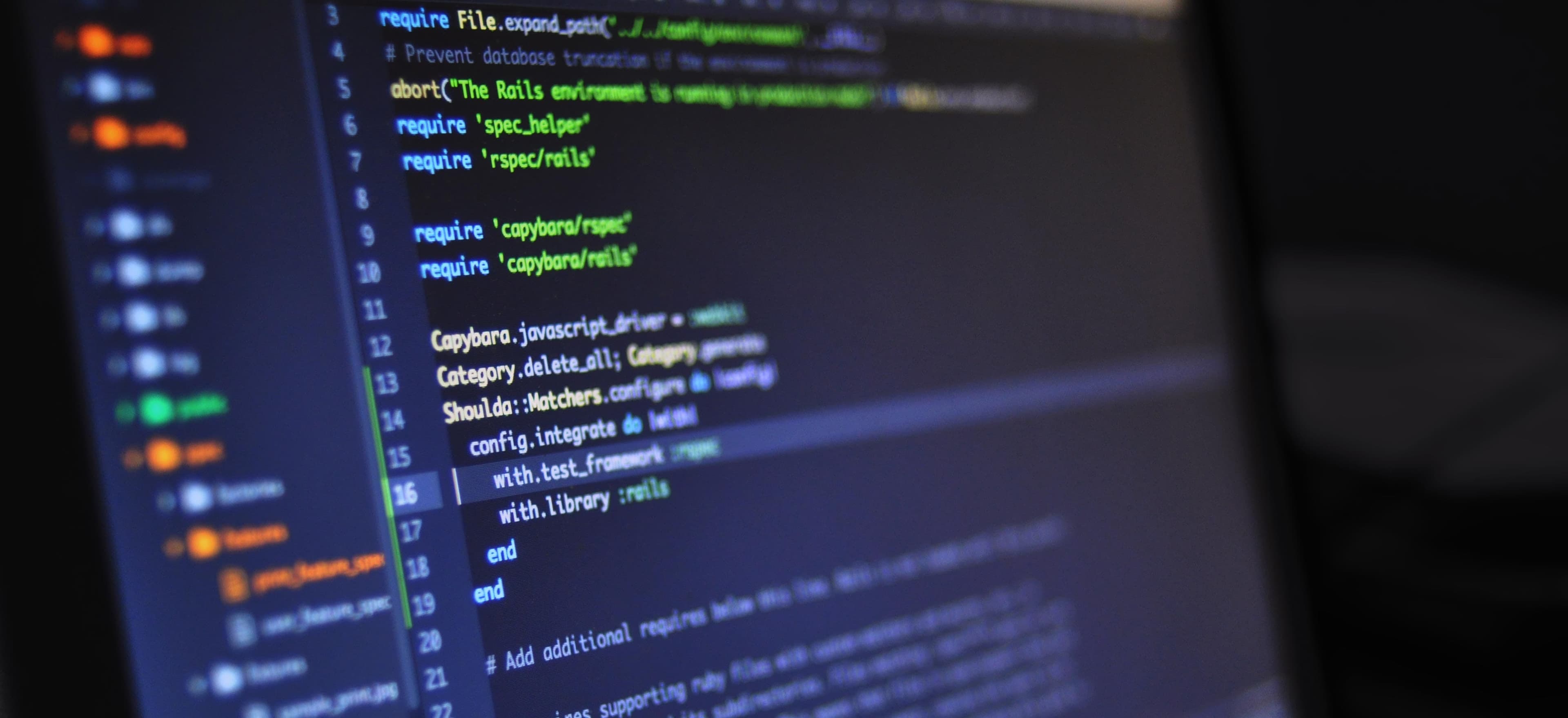
- Published on
Mastering Repeatable Reads in Hibernate Applications
When working on Hibernate applications, it's crucial to understand the concept of repeatable reads and how Hibernate handles this isolation level in database transactions. In this blog post, we will explore the significance of repeatable reads, their implementation in Hibernate, and how you can master this aspect to ensure data consistency and integrity in your applications.
Understanding Repeatable Reads
In database transactions, the isolation level defines the degree to which one transaction must be isolated from other transactions. Repeatable read is one such isolation level which ensures that during the course of a transaction, if a row is read multiple times, the result will remain consistent and unchanged.
This means that if you retrieve a set of records in a transaction, you can be confident that those records will not be modified or deleted by other transactions until your transaction is complete. This is important in scenarios where data consistency is critical, and it prevents issues such as dirty reads and non-repeatable reads.
Implementing Repeatable Reads in Hibernate
Hibernate provides support for managing transaction isolation levels, including repeatable reads. When working with Hibernate, you can control the isolation level at different levels, such as at the session level or even at the transaction level.
Setting Isolation Level at Session Level
Session session = sessionFactory.openSession();
session.setFlushMode(FlushMode.COMMIT);
session.setDefaultReadOnly(true);
session.setTransactionIsolation(Connection.TRANSACTION_REPEATABLE_READ);
In the above code snippet, we create a Hibernate session and set the default isolation level to TRANSACTION_REPEATABLE_READ
. Additionally, we set the flush mode to COMMIT
and mark the session as read-only by default. These settings help in enforcing the repeatable read semantics at the session level.
Controlling Isolation Level at Transaction Level
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
transaction.setIsolationLevel(Connection.TRANSACTION_REPEATABLE_READ);
In this code snippet, we start a Hibernate transaction and explicitly set the isolation level to TRANSACTION_REPEATABLE_READ
. This ensures that the specific transaction adheres to the repeatable read semantics, providing consistency for the duration of the transaction.
Mastering Repeatable Reads Best Practices
To master repeatable reads effectively in your Hibernate applications, it's essential to follow best practices that ensure optimal usage and understanding of this isolation level.
Proper Transaction Boundaries
One key practice is to define precise transaction boundaries in your application code. By clearly demarcating the start and end of transactions, you can control the scope of repeatable reads and ensure that the data remains consistent within each transaction.
Utilizing Optimistic Locking
Hibernate provides support for optimistic locking, which can be beneficial in conjunction with repeatable reads. By employing versioned entities and optimistic locking strategies, you can mitigate concurrency issues and maintain data consistency, especially in scenarios where repeatable reads are critical.
Testing and Validation
Thoroughly testing the behavior of repeatable reads in your Hibernate application is imperative. Write comprehensive unit tests that cover various transactional scenarios, including concurrent access, to validate that the repeatable read semantics are upheld as expected.
Final Considerations
Mastering repeatable reads in Hibernate applications is crucial for ensuring data consistency and integrity within transactions. By understanding the concept of repeatable reads, implementing it effectively in Hibernate, and adhering to best practices, you can harness the power of this isolation level to build robust and reliable database transactions.
Understanding how Hibernate manages database transactions and isolation levels can greatly impact the overall performance and reliability of your application. By mastering repeatable reads and incorporating them into your Hibernate applications, you can elevate the data integrity and consistency of your system to new heights.
Incorporate these best practices and code snippets into your Hibernate applications to ensure that repeatable reads are managed effectively, providing a solid foundation for transactional operations within your database.
Remember, mastering repeatable reads is not just about writing the code but also about understanding the underlying principles and implications of transaction isolation levels in Hibernate. Stay attentive to the needs of your application and leverage the power of repeatable reads to create resilient and dependable database interactions.
To delve deeper into the world of Hibernate and transaction management, check out the official Hibernate documentation.
Now, armed with a deeper understanding of repeatable reads in Hibernate applications, you can confidently navigate the complexities of transaction isolation levels and elevate the robustness of your database interactions.
Start mastering repeatable reads in Hibernate today!
Checkout our other articles