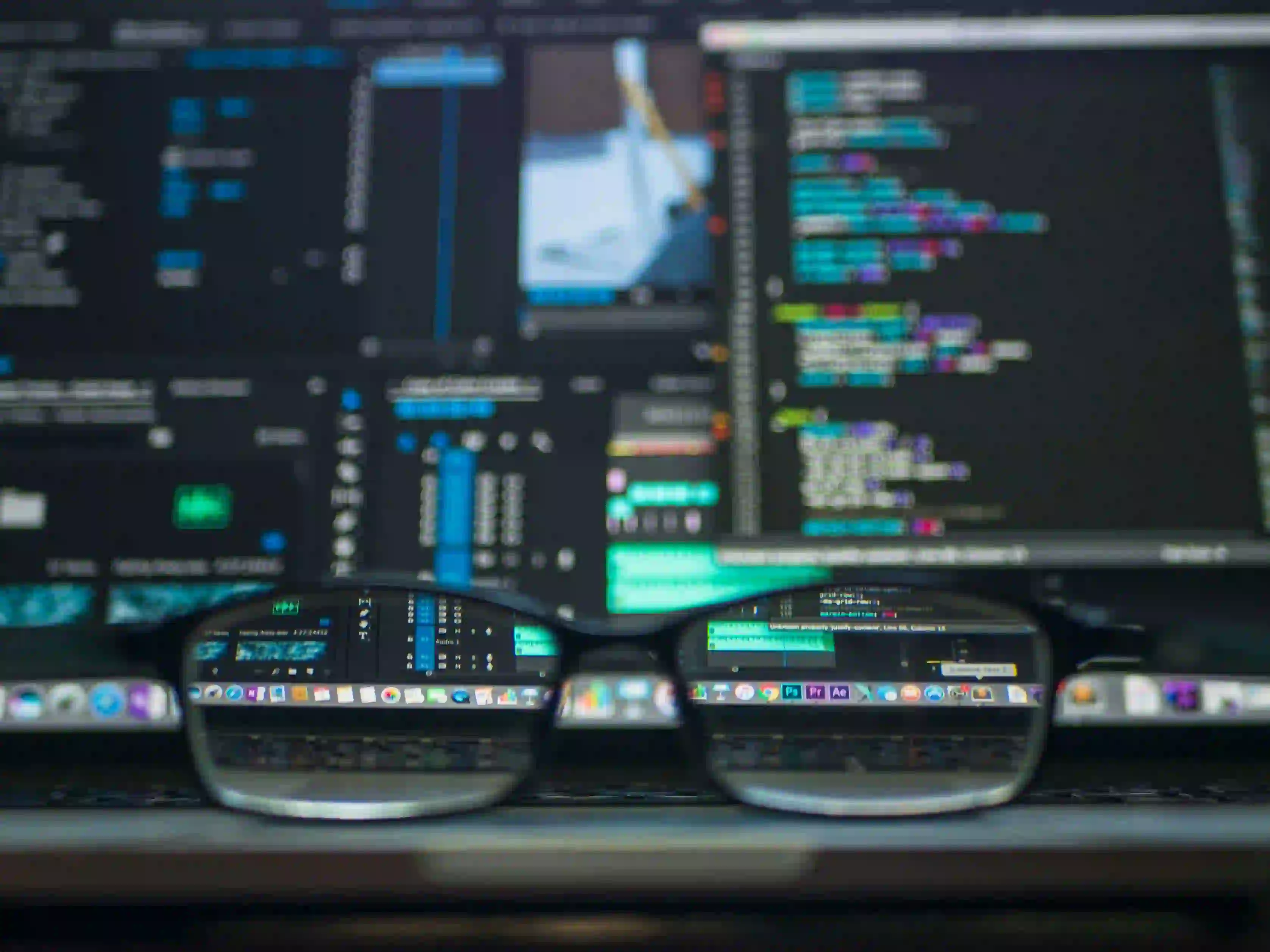
Mastering Spring Boot: Solving Complex Profile Management
Managing user profiles is a critical aspect of many applications. With Spring Boot, you can effectively handle complex profile management while leveraging its powerful features. In this post, we'll explore how to accomplish this, covering everything from basic CRUD operations to managing different user roles and permissions.
Understanding the Requirements
Before we dive into the implementation, let's define the requirements for our profile management system. We'll consider scenarios such as creating, reading, updating, and deleting user profiles, as well as managing user roles and permissions. Additionally, we'll need to secure the endpoints using Spring Security.
Setting Up the Project
To get started, we need to set up a new Spring Boot project. You can use Spring Initializr or your preferred IDE to generate a new project with the necessary dependencies such as Spring Web, Spring Data JPA, Spring Security, and H2 Database (for simplicity).
@SpringBootApplication
public class ProfileManagementApplication {
public static void main(String[] args) {
SpringApplication.run(ProfileManagementApplication.class, args);
}
}
Defining the User Entity
We'll begin by creating the User
entity, which will represent the user profiles in our system. We'll also define an enumeration for user roles.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
@Enumerated(EnumType.STRING)
private UserRole role;
// Getters and setters
// Constructors
}
public enum UserRole {
USER, ADMIN
}
Implementing the Repository
Next, we'll create a repository interface for the User
entity to perform CRUD operations.
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByUsername(String username);
}
Building the Service Layer
We'll then create a service layer to encapsulate the business logic for managing user profiles.
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User createUser(String username, String password, UserRole role) {
User user = new User();
user.setUsername(username);
user.setPassword(password);
user.setRole(role);
return userRepository.save(user);
}
// Other methods for updating, deleting, and retrieving user profiles
}
Securing the Endpoints
To secure the profile management endpoints, we'll configure Spring Security to restrict access based on user roles.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
// Configure user authentication (e.g., using in-memory authentication or UserDetailsService)
}
Testing the Endpoints
Finally, we'll create integration tests to ensure that our profile management endpoints function as expected.
@RunWith(SpringRunner.class)
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class ProfileManagementIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void givenAdminUser_whenGetAllUsers_thenSuccess() {
ResponseEntity<String> response = restTemplate
.withBasicAuth("admin", "password")
.getForEntity("/admin/users", String.class);
assertEquals(HttpStatus.OK, response.getStatusCode());
}
// Other test cases for creating, updating, and deleting user profiles
}
Key Takeaways
In this post, we've demonstrated how to effectively manage complex user profiles using Spring Boot. By leveraging its features such as Spring Data JPA for data access, Spring Security for endpoint security, and testing support, you can build a robust profile management system for your applications.
Whether you're developing a simple user management system or a sophisticated role-based access control system, Spring Boot provides the necessary tools to streamline the development process and ensure the security and integrity of your user profiles.
Start mastering Spring Boot for complex profile management today and unleash the full potential of your applications.