Mastering AJAX: Avoiding Common Beginner Pitfalls
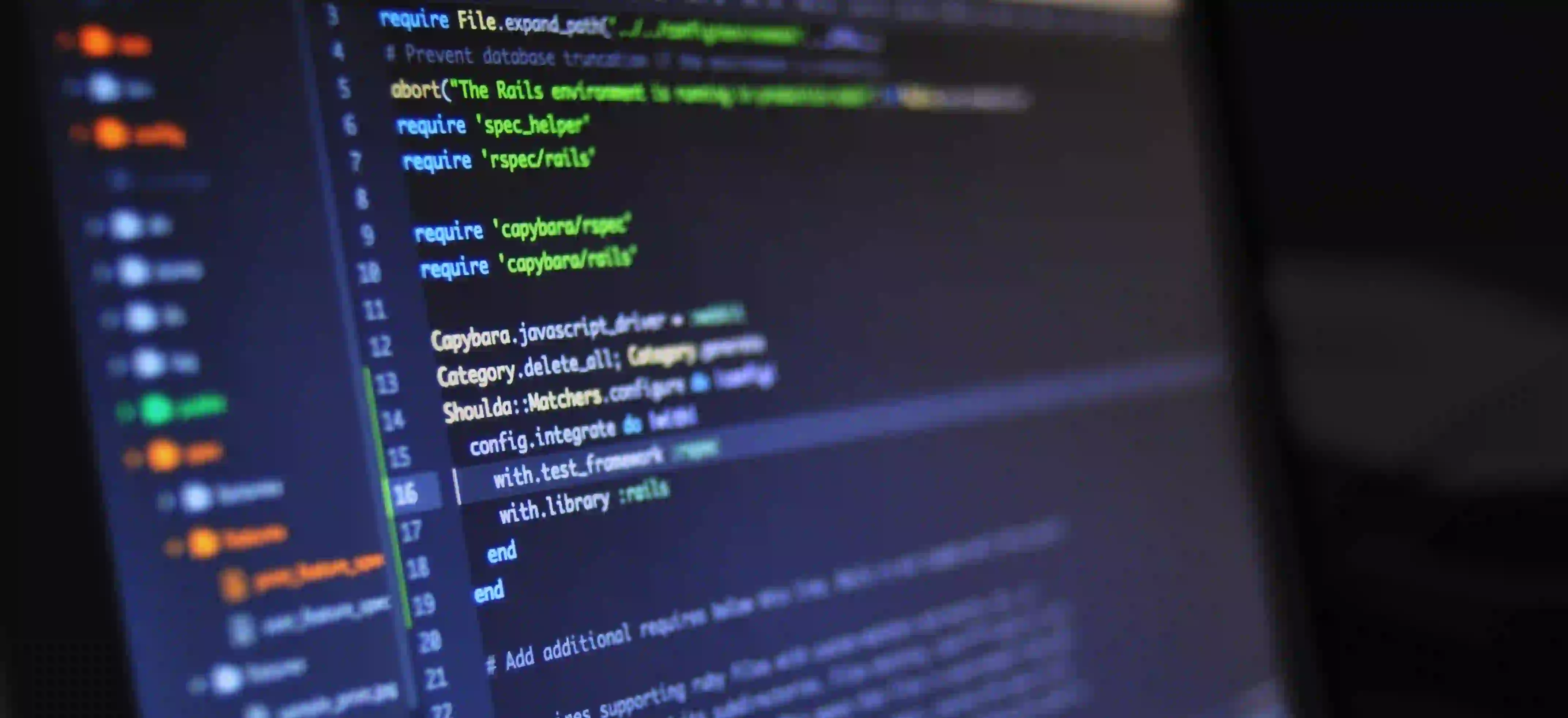
Mastering AJAX: Avoiding Common Beginner Pitfalls
AJAX is a powerful technology that allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. As a Java developer, it is essential to understand AJAX and how to use it effectively to create dynamic and responsive web applications. However, mastering AJAX can be challenging, especially for beginners. In this post, we will discuss some common pitfalls that beginners may encounter when working with AJAX in Java, and how to avoid them.
Understanding the Basics of AJAX
Before delving into the common pitfalls of AJAX, it is important to have a solid understanding of the basics. AJAX, which stands for Asynchronous JavaScript and XML, is not a new technology, but rather a combination of existing technologies, including HTML, CSS, JavaScript, XML, and the XMLHttpRequest object.
At its core, AJAX allows web pages to make asynchronous requests to the server, enabling them to update content without having to reload the entire page. This results in a more seamless and responsive user experience.
In Java, AJAX is commonly used in conjunction with frameworks like Spring MVC or Servlets to build dynamic web applications. Understanding how to integrate AJAX with Java backends is crucial for building modern web applications.
Common AJAX Pitfalls for Java Developers
1. Ignoring Asynchronous Behavior
One of the most common pitfalls for beginners working with AJAX is ignoring its asynchronous nature. AJAX requests are asynchronous by default, meaning that the rest of the code will continue to execute while the request is being processed. This can lead to unexpected behavior if not handled correctly.
2. Not Handling Errors Properly
Another common mistake is not handling errors properly when making AJAX requests. Without proper error handling, the user may not be informed of issues such as network errors or server-side failures, leading to a poor user experience.
3. Overlooking Cross-Origin Resource Sharing (CORS) Restrictions
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to prevent requests from different origins. Java developers often overlook CORS restrictions when making AJAX requests to a different domain, leading to blocked requests and unexpected behavior.
4. Handling Cross-Site Request Forgery (CSRF) Vulnerabilities
Security is a critical consideration when working with AJAX in Java. Failing to handle Cross-Site Request Forgery (CSRF) vulnerabilities can leave web applications susceptible to attacks. It's essential to implement proper CSRF protection when using AJAX to make requests to the server.
Avoiding Common Pitfalls
Now that we have identified some common pitfalls, let's discuss how to avoid them when working with AJAX in Java.
1. Embracing Asynchronous Behavior with Promises
To avoid the pitfalls of ignoring the asynchronous nature of AJAX, it is crucial to embrace it fully. Instead of relying on traditional callback functions, consider using Promises or async/await to handle asynchronous requests in a more structured and readable manner.
function fetchData() {
return new Promise((resolve, reject) => {
// Perform AJAX request
// Resolve the promise with the data
// Reject the promise if an error occurs
});
}
fetchData()
.then(data => {
// Handle the retrieved data
})
.catch(error => {
// Handle errors
});
Using Promises allows for more predictable code execution and better error handling, leading to more maintainable and reliable code.
2. Implementing Proper Error Handling
Proper error handling is crucial for providing a seamless user experience. When making AJAX requests in Java, ensure that you handle both client-side and server-side errors gracefully. Utilize the .fail() method in jQuery's AJAX function or catch() when using Promises to handle errors.
$.ajax({
url: "example.com/api/data",
method: "GET"
}).done(function(response) {
// Handle successful response
}).fail(function(xhr, status, error) {
// Handle errors
});
By handling errors properly, you can provide meaningful feedback to the user and improve the overall robustness of your web application.
3. Managing CORS Restrictions
When dealing with CORS restrictions in AJAX requests, it is essential to configure the server to include the appropriate CORS headers, allowing requests from different origins. In a Java backend, you can use libraries like Spring CORS to configure CORS policies and ensure that your AJAX requests are not blocked by the browser.
4. Mitigating CSRF Vulnerabilities
To mitigate CSRF vulnerabilities when using AJAX in Java, it is crucial to implement proper CSRF protection mechanisms. Utilize tokens or cookies to validate the authenticity of the requests and prevent unauthorized access to sensitive operations.
Frameworks like Spring Security provide built-in support for CSRF protection, making it easier to secure your AJAX requests against CSRF attacks.
Final Thoughts
In conclusion, mastering AJAX in Java requires a solid understanding of its asynchronous nature, error handling, and security considerations. By avoiding common pitfalls such as ignoring asynchronous behavior, not handling errors properly, overlooking CORS restrictions, and failing to manage CSRF vulnerabilities, you can build more robust and secure web applications.
It's essential to embrace asynchronous behavior with Promises, implement proper error handling, manage CORS restrictions, and mitigate CSRF vulnerabilities to create responsive and secure web applications using AJAX in Java.
By following best practices and staying informed about the latest advancements in AJAX and Java web development, you can elevate your skills and create exceptional web applications that deliver a seamless user experience.
For further reading on AJAX best practices in Java, you can check out the MDN Web Docs on AJAX and Spring Framework Documentation.