Boosting Productivity: Choosing Between Resource & Flow Efficiency
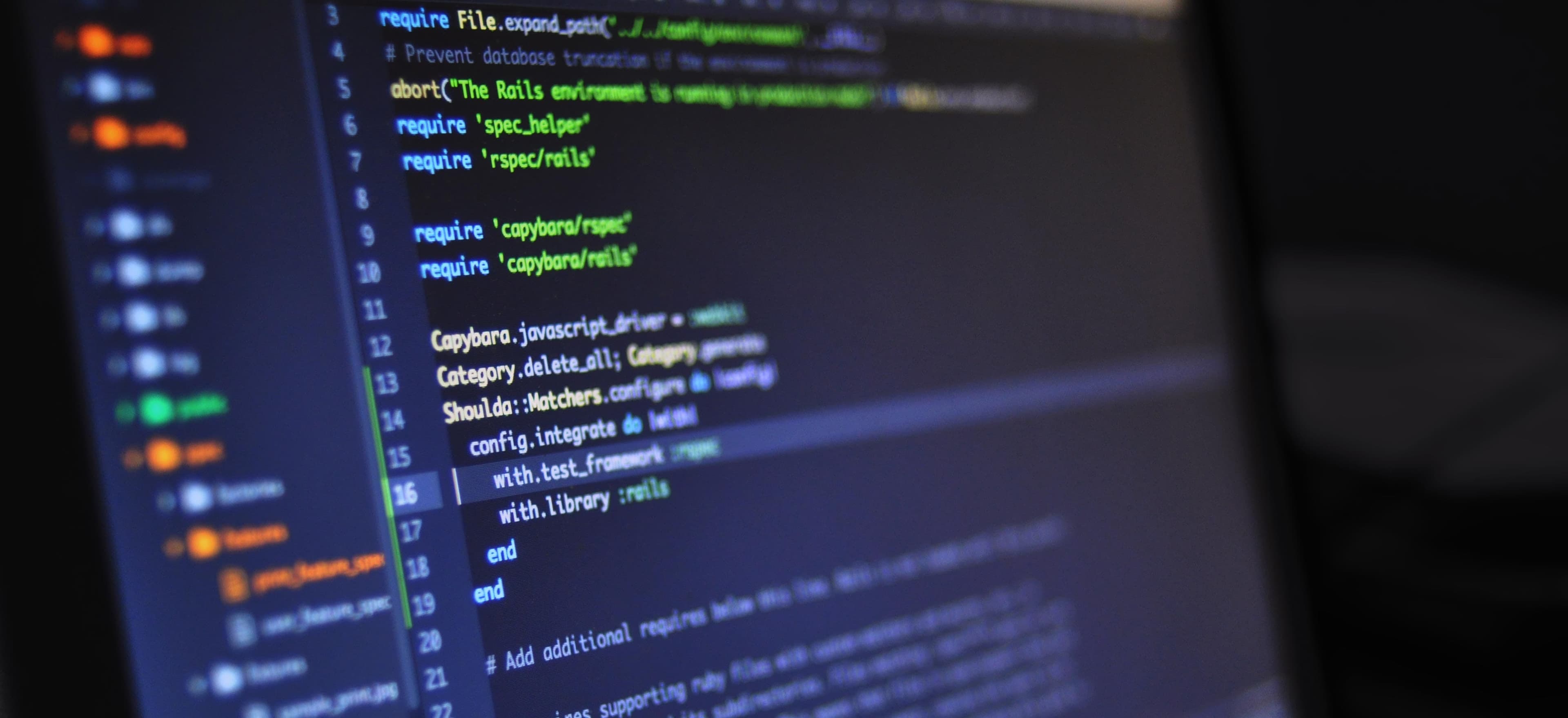
- Published on
Boosting Productivity: Choosing Between Resource & Flow Efficiency
In the realm of software development, Java has long reigned as a prominent language due to its versatility, reliability, and performance. However, developing software efficiently remains a perpetual challenge. One of the crucial decisions in structuring your Java applications lies in choosing between resource efficiency and flow efficiency. But before delving into the choice, let's understand the essence of these concepts.
Resource Efficiency
Resource efficiency in Java involves optimizing the usage of system resources such as memory, CPU, and I/O operations. While it's essential to economize these resources, striving for excessive resource efficiency can lead to convoluted and hard-to-maintain code. It’s imperative to strike a balance and prevent premature optimization, which can lead to code that's challenging to comprehend and modify.
Flow Efficiency
Flow efficiency, on the other hand, focuses on streamlining the flow of work through the system. In the context of Java development, this entails emphasizing readability, maintainability, and the seamless progression of the program logic. Prioritizing flow efficiency allows for agile development, easier debugging, and enhanced collaboration among the development team.
Now that we have a clear understanding of the two paradigms, let's explore how to navigate this decision-making process effectively.
The Dilemma: Resource vs. Flow Efficiency
When faced with the decision to prioritize either resource or flow efficiency, it’s crucial to consider the specific requirements of the project. While resource efficiency can lead to optimized performance, it should not come at the cost of creating complex and convoluted code that hampers maintenance and future development.
Conversely, solely focusing on flow efficiency might result in suboptimal resource utilization, impacting the performance of the application. Finding the equilibrium between the two is pivotal for delivering high-quality, efficient, and maintainable software.
Choosing Resource Efficiency in Java
Selecting resource efficiency in Java can be advantageous for projects with stringent performance requirements, especially with resource-constrained environments. Utilizing resource-efficient data structures and algorithms is crucial in scenarios where the application needs to maximize throughput or manage large-scale data processing.
// Example of resource-efficient algorithm: Binary Search
public int binarySearch(int[] arr, int key) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == key) {
return mid;
}
if (arr[mid] < key) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
The binary search algorithm is a prime illustration of resource efficiency, particularly in comparison to linear search. It drastically reduces the number of iterations required to find an element within a sorted array, thereby conserving computational resources.
Embracing Flow Efficiency in Java
In contrast, prioritizing flow efficiency in Java can significantly enhance the maintainability and readability of the codebase. Employing design patterns, adhering to coding best practices, and fostering a comprehensive testing culture are pivotal components of this approach. By emphasizing a clear and coherent code structure, developers can efficiently manage and evolve the codebase.
// Example of flow-efficient code: Utilizing design patterns
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a circle");
}
}
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Drawing a square");
}
}
Implementing the Factory Method pattern as shown above not only enhances flow efficiency but also fosters extensibility and maintainability. By encapsulating the object creation logic and promoting the use of interfaces, the code becomes more adaptable to future changes and additions.
Striking a Balance: The Best of Both Worlds
Striving for an optimal balance between resource and flow efficiency is imperative in Java development. Leveraging profiling tools to identify performance bottleneers, coupling them with an efficient algorithm and iteratively refining the code contributes to a harmonious coexistence of resource and flow efficiency.
Closing Remarks
In the realm of Java software development, the dichotomy between resource and flow efficiency presents a perennial challenge. While resource efficiency seeks to optimize system resources, flow efficiency stresses the seamless progression and maintainability of the code. Striking a balance between the two is essential, as an excessive focus on either can compromise the overall quality of the software.
In conclusion, understanding the inherent trade-offs between resource and flow efficiency paves the way for making informed decisions that align with the distinct requirements of each project. By embracing the best of both paradigms, Java developers can navigate the complexity of software development while delivering robust, efficient, and maintainable applications.