Mastering Mockito: Solving JAXB Deep Stubs Dilemma
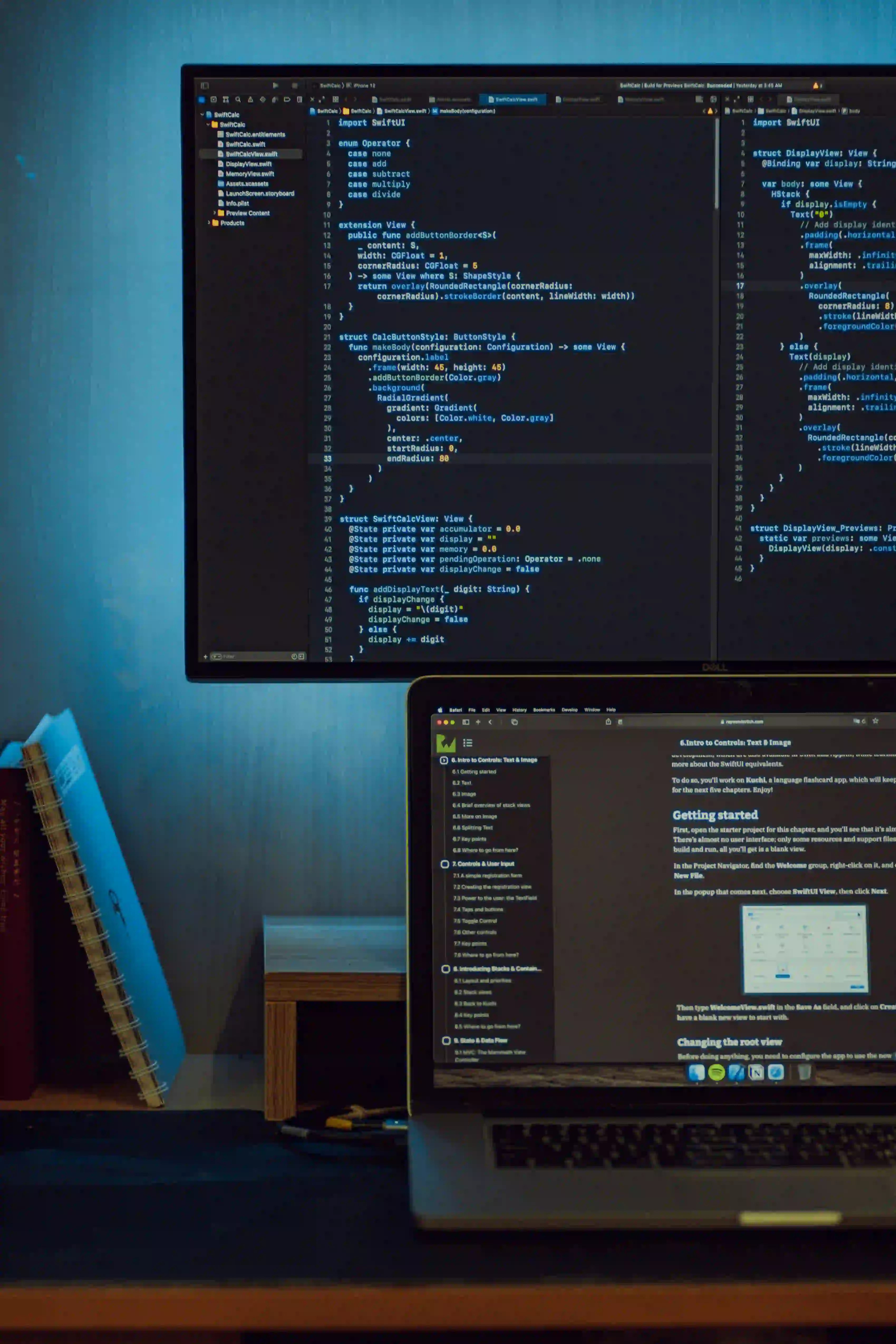
Mastering Mockito: Solving JAXB Deep Stubs Dilemma
Mockito is a powerful and widely used mocking framework for Java. It allows developers to effectively test code by creating mock objects, stubbing method calls, and verifying interactions between objects. However, Mockito has a limitation when it comes to working with deep object hierarchies, especially for classes generated from JAXB (Java Architecture for XML Binding) or similar libraries. In this article, we will explore this issue and provide a solution using Mockito deep stubs.
Understanding the JAXB Deep Stubs Dilemma
When working with JAXB-generated classes, which often represent complex XML structures, developers encounter challenges when using Mockito to stub methods deep within the object hierarchy. This is due to the default behavior of Mockito, which only allows stubbing and verification of methods directly invoked on mock objects.
Consider the following scenario:
public class OrderService {
private OrderRepository orderRepository;
public void processOrder(Order order) {
// Business logic
orderRepository.saveOrder(order);
}
}
In this example, if Order
is a JAXB-generated class with deep object hierarchy, stubbing method calls on it using Mockito's default behavior becomes cumbersome.
Introducing Deep Stubs
To address this issue, Mockito provides the concept of deep stubs. Deep stubs allow developers to stub method calls on nested objects within a mock object's hierarchy. This feature is particularly useful when working with JAXB-generated classes, as it enables seamless mocking and stubbing of methods deep within the object structure.
Let's take a look at how we can utilize deep stubs to overcome the JAXB deep stubs dilemma.
Utilizing Deep Stubs in Mockito
To enable deep stubbing in Mockito, we can use the then
method from the AdditionalAnswers
class. This allows us to stub the method calls on nested objects within the mock object hierarchy.
Here's an example demonstrating the usage of deep stubs with JAXB-generated classes:
@Test
public void testProcessOrder() {
OrderRepository orderRepository = mock(OrderRepository.class, RETURNS_DEEP_STUBS);
OrderService orderService = new OrderService(orderRepository);
Order order = new Order();
// Set up order object
// Stubbing deep method call on the mocked OrderRepository
when(orderRepository.saveOrder(any(Order.class)).then(invocation -> {
// Custom behavior
return true;
});
orderService.processOrder(order);
// Verify the method call
verify(orderRepository).saveOrder(order);
}
In this example, we create a mock OrderRepository
with deep stubs enabled using RETURNS_DEEP_STUBS
. We then stub the saveOrder
method call on the nested Order
object within the OrderRepository
mock.
By using the then
method, we provide custom behavior for the stubbed method call, allowing us to test the processOrder
method in the OrderService
class effectively.
Benefits of Using Deep Stubs
Utilizing deep stubs in Mockito offers several benefits, especially when dealing with complex object hierarchies such as those generated by JAXB.
- Simplified Mocking: Deep stubs simplify the process of mocking and stubbing methods on deeply nested objects, reducing the complexity of test setup.
- Improved Test Readability: By allowing seamless interaction with nested objects, deep stubs improve the readability of tests, making it easier to understand the mock object's behavior.
- Efficient Testing of Complex Logic: Deep stubs enable developers to effectively test methods that interact with complex object hierarchies, such as those found in JAXB-generated classes.
Bringing It All Together
In conclusion, the use of deep stubs in Mockito provides an effective solution to the JAXB deep stubs dilemma. By enabling seamless mocking and stubbing of methods within deeply nested object hierarchies, developers can efficiently test code that interacts with complex JAXB-generated classes.
By leveraging the then
method from the AdditionalAnswers
class and enabling deep stubs using RETURNS_DEEP_STUBS
, developers can overcome the limitations of Mockito when working with JAXB-generated classes.
Mastering Mockito and understanding how to effectively utilize features like deep stubs is essential for writing robust and comprehensive unit tests for Java applications.
To further explore Mockito and deep stubbing, check out the official Mockito documentation and the Mockito GitHub repository for detailed information and examples.
Happy testing!