Polyglot Pitfalls: Navigating Language Complexity in Apps
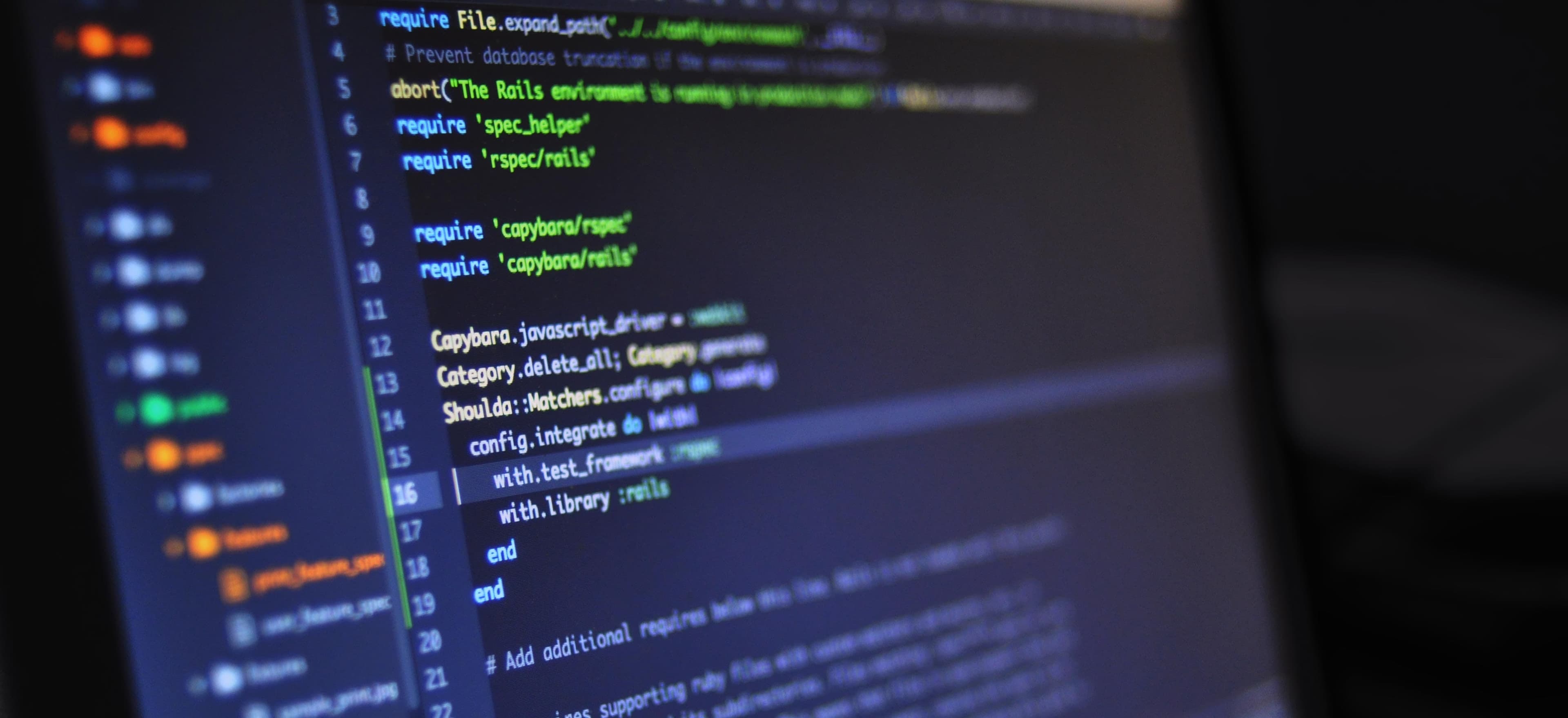
- Published on
Polyglot Pitfalls: Navigating Language Complexity in Apps
In the world of software development, choosing the right programming language for a project is crucial. Each language has its own set of strengths and weaknesses, and developers must carefully consider these factors to ensure the success of their applications. Java, as one of the most widely used programming languages, offers a powerful and versatile platform for building a wide range of applications. This blog post will explore the complexities and challenges of using Java in app development, and provide insights into how developers can navigate these pitfalls to create robust and efficient applications.
Understanding the Complexity of Java
Java is renowned for its performance, portability, and security features, making it an attractive choice for developing enterprise-level applications, mobile apps, and web services. However, its complexity lies in its extensive standard libraries, diverse frameworks, and the need for proper memory management. While these aspects contribute to Java's robustness, they also present challenges for developers, especially when it comes to optimizing performance, managing memory efficiently, and ensuring scalability.
Performance Optimization in Java
When it comes to performance optimization in Java, developers often face the challenge of balancing speed and resource efficiency. Java's automatic memory management through garbage collection can impact performance, leading to occasional pauses and unpredictable latency. To address this, developers can implement various strategies such as object pooling, caching, and optimizing data structures to minimize memory overhead and improve overall performance.
// Example of object pooling in Java
public class ObjectPool<T> {
private List<T> pool = new ArrayList<>();
public T borrowObject() {
if (pool.isEmpty()) {
return createObject();
}
return pool.remove(0);
}
public void returnObject(T object) {
pool.add(object);
}
private T createObject() {
// Logic to create and return a new object
}
}
In the code snippet above, object pooling is demonstrated as a technique to efficiently manage and reuse instances, thus reducing the overhead of object creation and memory allocation.
Memory Management in Java
Java's automatic memory management alleviates the burden of manual memory allocation and deallocation, but it also introduces the challenge of optimizing memory usage. Memory leaks and inefficient resource utilization can negatively impact the performance and scalability of Java applications. To mitigate these issues, developers can leverage tools like Java Memory Model and profilers to identify memory leaks and inefficient memory usage patterns, allowing for proactive optimization and fine-tuning of the application's memory footprint.
Navigating Language Complexity
While Java presents challenges in terms of performance optimization and memory management, developers can navigate these complexities by adopting best practices, utilizing modern tools and frameworks, and staying informed about the latest advancements in Java development. Embracing a proactive and strategic approach is essential for harnessing the full potential of Java and delivering high-quality, reliable applications.
Best Practices for Java Development
Adhering to best practices is fundamental for overcoming the challenges associated with Java development. Writing clean, modular, and maintainable code, following design patterns, and practicing defensive programming are essential to mitigate potential pitfalls. Additionally, staying updated with the latest Java features, enhancements, and best practices can empower developers to make informed decisions and optimize their applications effectively.
Modern Tools and Frameworks
The Java ecosystem offers a plethora of modern tools and frameworks that simplify and streamline the development process. Frameworks like Spring and Hibernate provide robust solutions for building enterprise-level applications, while tools like JUnit and Mockito facilitate efficient testing and mocking. Embracing these tools and frameworks not only enhances productivity but also enables developers to address performance and memory management challenges effectively.
Evolving with Java
As Java continues to evolve with new releases and updates, developers must stay abreast of the latest trends and advancements in the Java ecosystem. Features such as Project Loom, which aims to introduce lightweight concurrency models, and enhanced memory management techniques in newer Java versions, underscore the importance of adapting to the evolving landscape of Java development. By embracing these advancements and leveraging modern Java features, developers can effectively navigate the intricacies of Java and elevate the performance and efficiency of their applications.
The Last Word
Java's complexity in performance optimization and memory management presents challenges for developers, but with a strategic mindset, adherence to best practices, and a proactive approach to utilizing modern tools and frameworks, these challenges can be effectively mitigated. Navigating the complexities of Java requires a holistic understanding of its capabilities and limitations, along with a continuous commitment to staying informed and evolving with the Java ecosystem.
In summary, Java's complexity should not deter developers, but rather inspire them to leverage its robustness and versatility to build powerful and efficient applications that meet the demands of modern software development.
By equipping themselves with the right knowledge, skills, and tools, developers can navigate the polyglot pitfalls of Java and unlock its full potential in app development.
References:
- Java Memory Management Best Practices
- Java Performance Optimization Techniques
- Project Loom: Lightweight Concurrency for Java