Boost Your Biz: Ace Integration Tests in Enterprise Systems
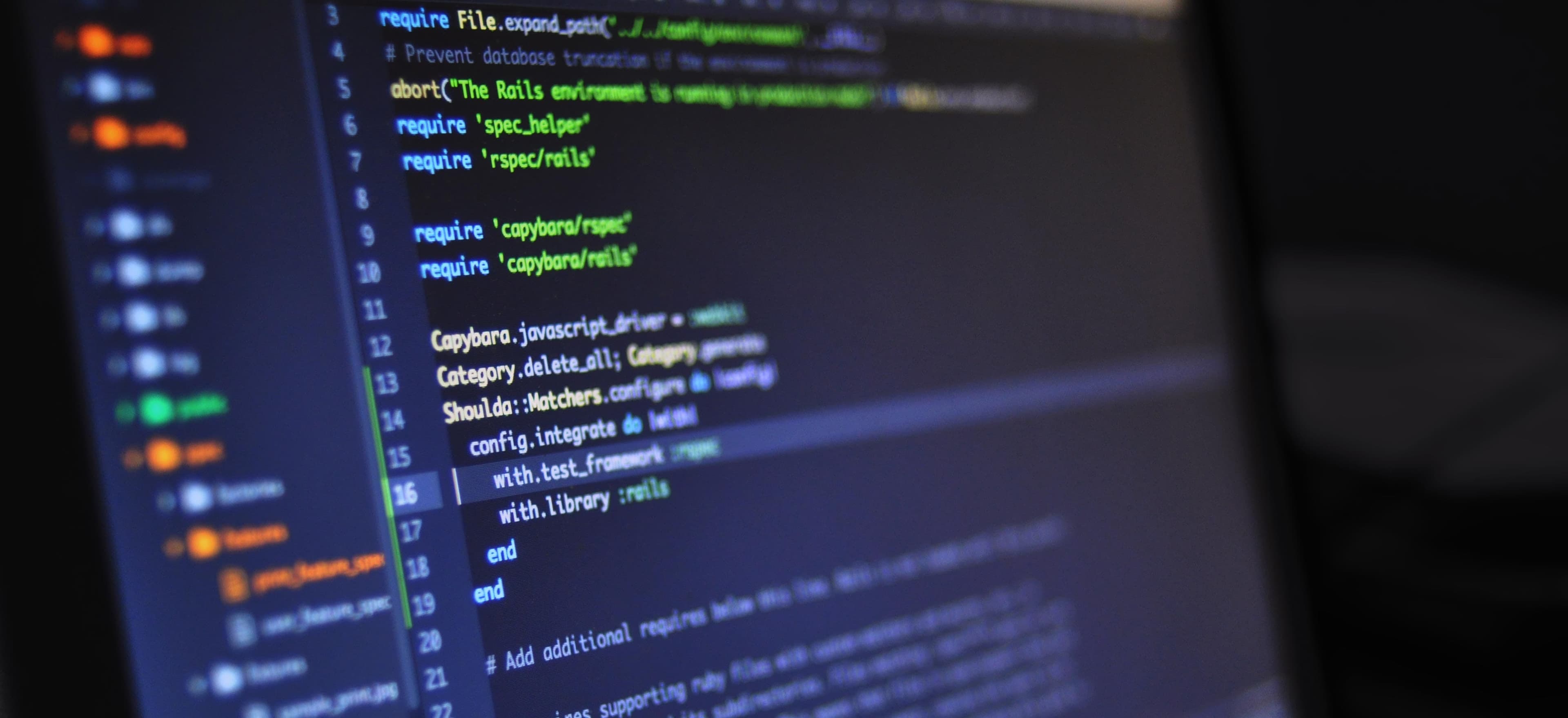
- Published on
Boost Your Biz: Ace Integration Tests in Enterprise Systems
Integration testing is an indispensable component of any enterprise system's testing strategy. It ensures that all individual modules work together seamlessly as a unified system. In Java development, integration testing plays a pivotal role in maintaining the integrity and reliability of enterprise systems.
In this article, we'll dive deep into integration testing in Java enterprise systems. We'll explore essential concepts, best practices, and demonstrate how to write effective integration tests to ensure your business-critical applications perform flawlessly.
Understanding Integration Testing
What is Integration Testing?
Integration testing is the process of testing the interactions between different parts of a system to ensure they work together as expected. In an enterprise system, this involves testing the communication and data flow between various components, such as databases, APIs, and external services.
Benefits of Integration Testing in Enterprise Systems
- Detects defects early in the development lifecycle
- Validates the behavior of interconnected components
- Ensures the system works as a cohesive unit
- Provides confidence in the system's overall functionality
Integration Testing in Java: Best Practices
1. Use a Reliable Testing Framework
Java offers a plethora of testing frameworks, such as JUnit and TestNG, that provide robust support for writing integration tests. Choose a framework that best aligns with your project's requirements and has good community support.
2. Isolate External Dependencies
When writing integration tests, it's crucial to isolate external dependencies, such as databases and third-party services, to maintain test stability and repeatability. Utilize tools like Docker to spin up containers for dependent services or employ mocking libraries such as Mockito to simulate external behavior.
3. Leverage Test Data Management
Managing test data is critical for integration testing. Incorporate tools like Flyway or Liquibase to version control your database schema and seed test data. This ensures consistent and predictable test environments.
4. Implement Test Environment Configuration
Configuration management is vital for creating consistent test environments. Use tools like Spring Profiles to manage different environment configurations, allowing seamless integration testing across multiple environments.
Writing Effective Integration Tests in Java
Now, let's delve into the implementation of effective integration tests in Java using JUnit, a widely adopted testing framework.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
public class IntegrationTestExample {
@Test
void givenValidData_whenAPICallMade_thenRetrieveExpectedResult() {
// Arrange
// Set up test data or mock external dependencies
// Act
// Trigger the API call or service invocation
// Assert
// Validate the expected outcome
}
}
In the above example, we define an integration test using JUnit. The test method encompasses three key phases:
- Arrange: Set up the preconditions for the test. This may involve initializing test data or mocking external dependencies.
- Act: Invoke the API call or service that is being tested.
- Assert: Verify the expected outcome of the test.
By following this structure, integration tests become organized, self-documenting, and easier to maintain.
Dealing with External Dependencies
In enterprise systems, integration tests often involve interacting with external components like databases and web services. It's imperative to handle these dependencies effectively to ensure test reliability.
Let's take a look at an example of how you can mock a database interaction using Mockito in an integration test.
import static org.mockito.Mockito.*;
public class DatabaseIntegrationTest {
@Test
void givenDataInDB_whenRetrieved_thenNotNull() {
// Arrange
DataSource dataSource = mock(DataSource.class);
Connection connection = mock(Connection.class);
when(dataSource.getConnection()).thenReturn(connection);
// Act
// Perform database interaction
// Assert
// Validate the expected result
}
}
In the above snippet, we use Mockito to mock the behavior of the database interaction, allowing us to focus solely on the functionality being tested without relying on a live database.
Ensuring Test Data Consistency
Managing test data consistency is crucial for reliable integration testing. Tools like Flyway and Liquibase assist in versioning and maintaining the integrity of the test data and schema.
Here's an example of using Flyway to initialize test data in an integration test:
import org.flywaydb.core.Flyway;
public class TestDataInitializationTest {
@Before
public void setupTestData() {
Flyway flyway = Flyway.configure().dataSource(url, user, password).load();
flyway.migrate();
}
@Test
void givenTestEnvironment_whenDataInitialized_thenVerifyConsistency() {
// Perform assertions to verify data consistency
}
}
In this example, we use Flyway to migrate the test database to a consistent state before running the integration test, ensuring the reliability of the test data.
My Closing Thoughts on the Matter
Effective integration testing is paramount in ensuring the robustness and reliability of enterprise systems. By following best practices, leveraging reliable testing frameworks, and effectively managing external dependencies and test data, you can build a comprehensive integration testing strategy for your Java enterprise applications.
Integration testing in Java is a combination of meticulous planning, strategic implementation, and leveraging the right tools to achieve comprehensive test coverage. By mastering the art of integration testing, you can ensure that your business-critical applications operate seamlessly, fostering trust and confidence among users and stakeholders alike.
Incorporate these best practices into your development workflows, and see your enterprise systems thrive with resilient, well-tested components that work harmoniously together. Happy testing!
Checkout our other articles