Boosting Site Performance: Elasticsearch Full-Text Search Secrets
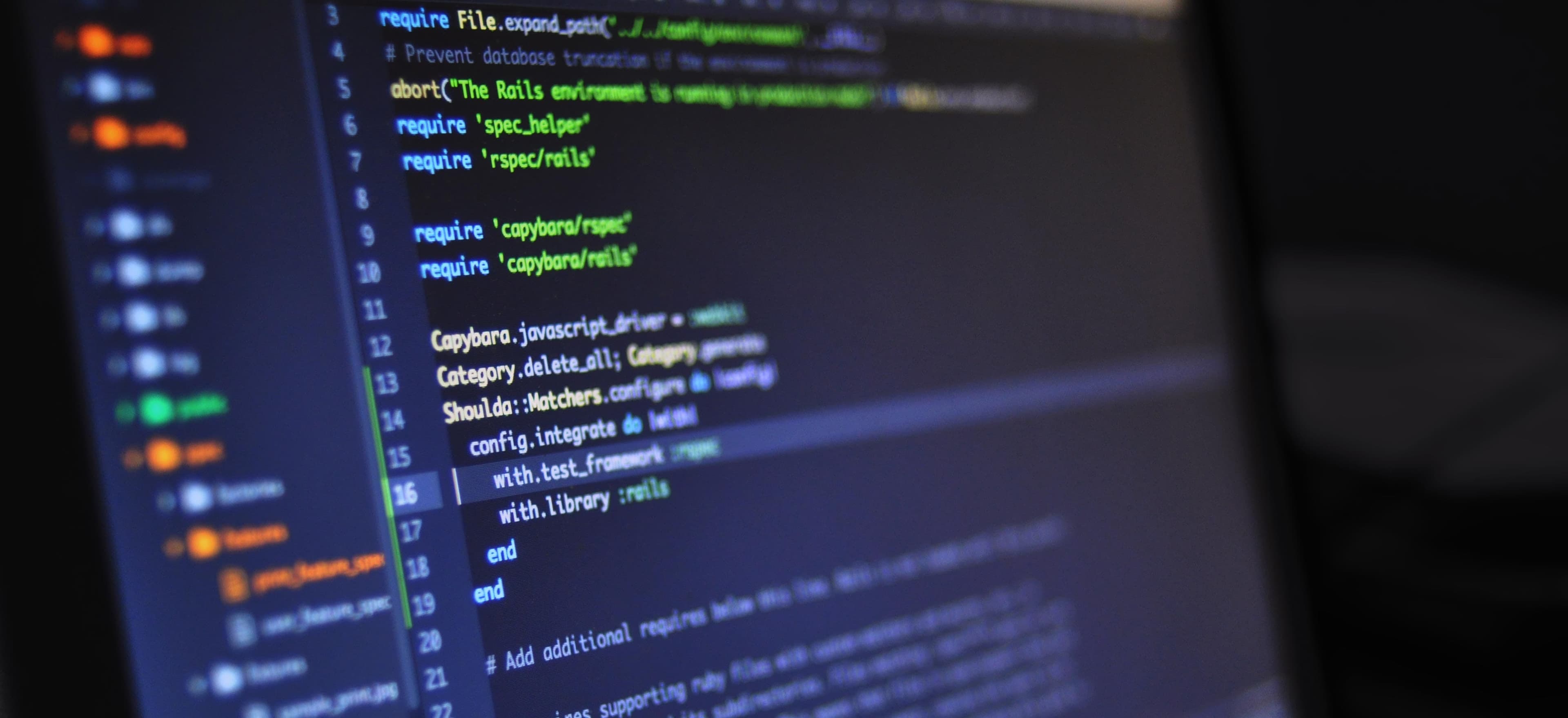
- Published on
Boosting Site Performance: Elasticsearch Full-Text Search Secrets
In the age of information overload, efficient search capabilities are crucial for any successful website. Users expect fast and accurate search results, and poor performance can lead to frustration and lost opportunities. This is where Elasticsearch, a highly scalable open-source full-text search engine, comes into play. With its powerful indexing and search capabilities, Elasticsearch has gained popularity for its ability to deliver lightning-fast search results.
Why Elasticsearch?
Elasticsearch's distributed nature and real-time search capabilities make it a natural choice for applications dealing with large volumes of data. Its ability to efficiently handle unstructured data, such as logs or documents, also makes it a popular choice for building search functionality into a website.
Getting Started with Elasticsearch and Java
To start utilizing Elasticsearch in a Java application, we first need to include the relevant dependencies in our project's pom.xml
file if using Maven:
<dependency>
<groupId>org.elasticsearch</groupId>
<artifactId>elasticsearch</artifactId>
<version>7.15.1</version> <!-- Replace with the latest version -->
</dependency>
Now, let's delve into some best practices and a few "secrets" to enhance our Elasticsearch full-text search performance in a Java environment.
1. Indexing Data
Indexing is the process of structuring and organizing data to facilitate efficient retrieval. When working with Elasticsearch, thoughtful indexing is crucial for optimizing search performance.
Why Is Proper Indexing Important?
Adequate indexing:
- Reduces the search scope, leading to faster search.
- Enables relevancy calculations and scoring for search results.
- Facilitates efficient data retrieval.
Example Code for Indexing
Let's take a look at how we can index a sample document using the Elasticsearch Java High Level REST Client:
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(new HttpHost("localhost", 9200, "http")));
IndexRequest request = new IndexRequest("posts");
request.id("1");
String jsonString = "{"title": "Elasticsearch Performance Tuning", "content": "Learn how to optimize Elasticsearch performance."}";
request.source(jsonString, XContentType.JSON);
IndexResponse indexResponse = client.index(request, RequestOptions.DEFAULT);
In this example, we create an index request for a document with a title and content, then execute the request using the High Level REST Client.
2. Query Performance Optimization
Efficient querying is vital for maximizing search performance. Utilizing the appropriate query types and understanding how they affect search performance is key to optimizing Elasticsearch for full-text search.
Choose the Right Query Type
Elasticsearch provides various query types, each suited for different use cases. For full-text search, the match
and multi_match
queries are commonly used.
Why Use match
and multi_match
?
match
: This query is suitable for analyzing full-text fields and supports fuzziness, stemming, and relevance tuning.multi_match
: When searching across multiple fields, this query type enables simultaneous search across different fields, thus enhancing search performance.
Example Code for Querying
Let's consider an example of how to utilize the multi_match
query using the Elasticsearch Java High Level REST Client:
SearchRequest searchRequest = new SearchRequest("posts");
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
searchSourceBuilder.query(QueryBuilders.multiMatchQuery("Elasticsearch Performance", "title", "content"));
searchRequest.source(searchSourceBuilder);
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
In this example, we construct a multi_match
query to search for the term "Elasticsearch Performance" within the title and content fields of the indexed documents.
3. Scaling for Performance
As the volume of data grows, scaling Elasticsearch becomes crucial for maintaining optimal search performance.
Why Scale Elasticsearch?
- Ensures High Availability: Distributing data across multiple nodes mitigates the risk of downtime and data loss.
- Improves Throughput: Distributing indexing and query load across multiple nodes enhances overall search performance.
- Handles Increased Data Volume: Scaling the cluster allows for seamless expansion to accommodate growing data.
Example Code for Cluster Scaling
Scaling a cluster involves adding more nodes to distribute the data and query load. Using the Java High Level REST Client, we can add a new data node to the cluster as follows:
ClusterUpdateSettingsRequest request = new ClusterUpdateSettingsRequest()
.transientSettings(Collections.singletonMap("cluster.routing.allocation.enable", "all"));
client.cluster().putSettings(request, RequestOptions.DEFAULT);
In this snippet, we update the cluster settings to enable allocation across all nodes, allowing the cluster to distribute the data and query load efficiently.
Closing Remarks
Optimizing Elasticsearch for full-text search in a Java environment involves a combination of proper indexing, efficient querying, and strategic cluster scaling. By adhering to these best practices and "secrets" of Elasticsearch, you can significantly enhance the search performance of your application.
In summary, Elasticsearch empowers Java developers to build robust search functionalities, and with the right techniques, it can deliver exceptional performance, making it an invaluable asset for any data-intensive application.
By integrating Elasticsearch, you can deliver a seamless and efficient search experience, ultimately enhancing user satisfaction and the overall success of your application.
Now that we’ve uncovered some essential tips and tricks, it’s time to put them into practice and witness the performance enhancements firsthand. Happy optimizing!
Remember, the world of Elasticsearch is vast and continually evolving, so staying updated with the latest advancements and best practices is key to unlocking the full potential of this powerful search engine. Happy searching!