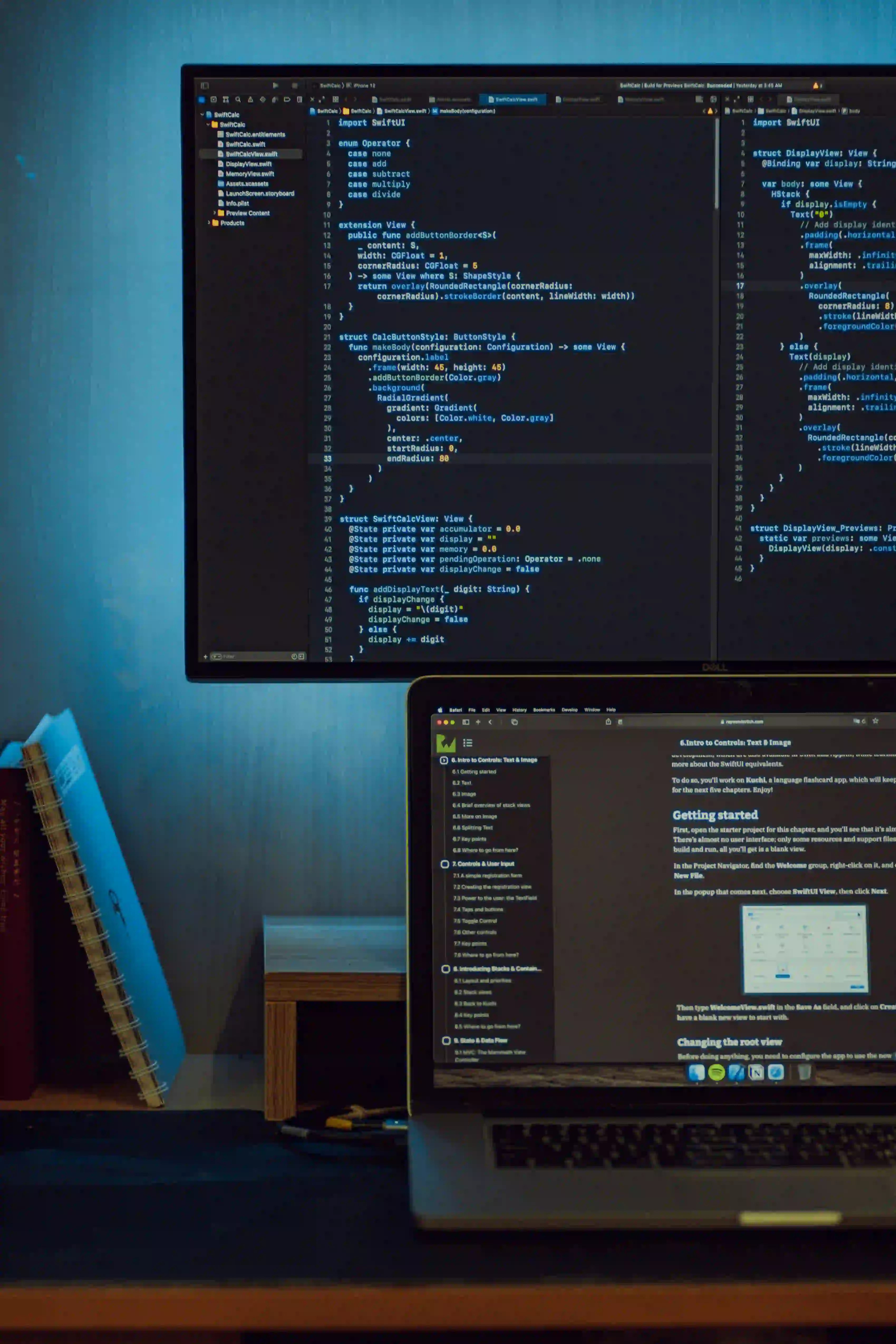
Unpacking the Mystery: Mastering Tuple Destructuring in Reactor
If you're delving into reactive programming with Java, you must be familiar with Project Reactor. One of the core concepts in Reactor is tuple destructuring, which can be a powerful tool in your reactive programming arsenal. In this post, we'll dive deep into tuple destructuring in Reactor, exploring its importance, how it works, and how you can leverage it effectively in your projects.
Understanding Tuples
Before we explore tuple destructuring, let's first understand what tuples are. A tuple is a fixed-size, immutable collection of heterogeneous elements. In the context of Project Reactor, a tuple typically represents a fixed number of values emitted by a Flux or Mono.
In Reactor, tuples are commonly represented using the Tuple
class, which provides T1
, T2
, T3
, and so on, up to T8
, representing the different elements of the tuple.
Tuple Destructuring: Unveiling Its Power
Tuple destructuring allows you to unpack the elements of a tuple and work with them individually. This technique brings clarity and expressiveness to your code, making it easier to handle multiple values emitted from reactive streams.
Syntax of Tuple Destructuring
In Java, tuple destructuring can be achieved using the subscribe
method of Flux or Mono. Here's a simple example:
Flux<Tuple2<String, Integer>> tupleFlux = Flux.just(Tuples.of("A", 1), Tuples.of("B", 2));
tupleFlux.subscribe(t -> {
String letter = t.getT1();
int number = t.getT2();
System.out.println("Letter: " + letter + ", Number: " + number);
});
In this example, the subscribe
method allows us to destructure the tuple and retrieve the individual elements T1
and T2
for further processing.
Why Tuple Destructuring?
Tuple destructuring offers several benefits:
-
Clarity: Destructuring tuples provides a clear and concise way of handling multiple values, improving code readability.
-
Type Safety: By accessing tuple elements using explicit methods like
getT1()
,getT2()
, etc., tuple destructuring ensures type safety at compile time. -
Simplicity: It simplifies the handling of multiple values by directly accessing the individual elements.
Real-World Scenarios: Leveraging Tuple Destructuring
Now that we understand the basics of tuple destructuring, let's explore some real-world scenarios where it can be incredibly useful.
Database Operations
When working with reactive database access in Java, tuple destructuring simplifies handling the results of queries that return multiple values. For example, when retrieving a user's details, you can easily destructure the tuple to access the user's name, email, and other attributes.
REST API Responses
In a reactive web application, when consuming a REST API that returns tuples of data, tuple destructuring makes it straightforward to unpack the response and process the individual pieces of information.
Error Handling
Tuple destructuring also plays a vital role in error handling. When dealing with reactive flows, errors may be represented as tuples, containing an error code and a descriptive message. You can use tuple destructuring to extract and handle the error information seamlessly.
Tuple Destructuring in Reactor: Best Practices
As with any programming technique, there are best practices to consider when working with tuple destructuring in Reactor.
Use Proper Naming
When deconstructing tuples, use clear and concise variable names that reflect the meaning of the deconstructed values. This promotes readability and maintains code clarity.
Handle null Values
Be mindful of null values when working with tuple destructuring. Always check for null values and handle them appropriately to avoid potential NullPointerExceptions.
Consider Pattern Matching
With the introduction of pattern matching in Java 16, you can consider using pattern matching to destructure tuples more elegantly in the future.
Lessons Learned
In the realm of reactive programming with Project Reactor, tuple destructuring emerges as a valuable tool for handling multiple values emitted by reactive streams. By using tuple destructuring, you can simplify code, improve readability, and enhance the expressiveness of your reactive applications.
Understanding the syntax and benefits of tuple destructuring, along with best practices, equips you to wield this technique effectively in your projects. As you continue your journey in reactive programming, mastering tuple destructuring will undoubtedly amplify your ability to craft robust and elegant solutions in Java.
So, roll up your sleeves and unlock the potential of tuple destructuring in Reactor to propel your reactive Java applications to new heights!
For more in-depth insights into Project Reactor and reactive programming with Java, check out the official Project Reactor documentation and explore the endless possibilities of reactive programming in Java.