How to Spot and Manage Inactive Okta Users Effectively
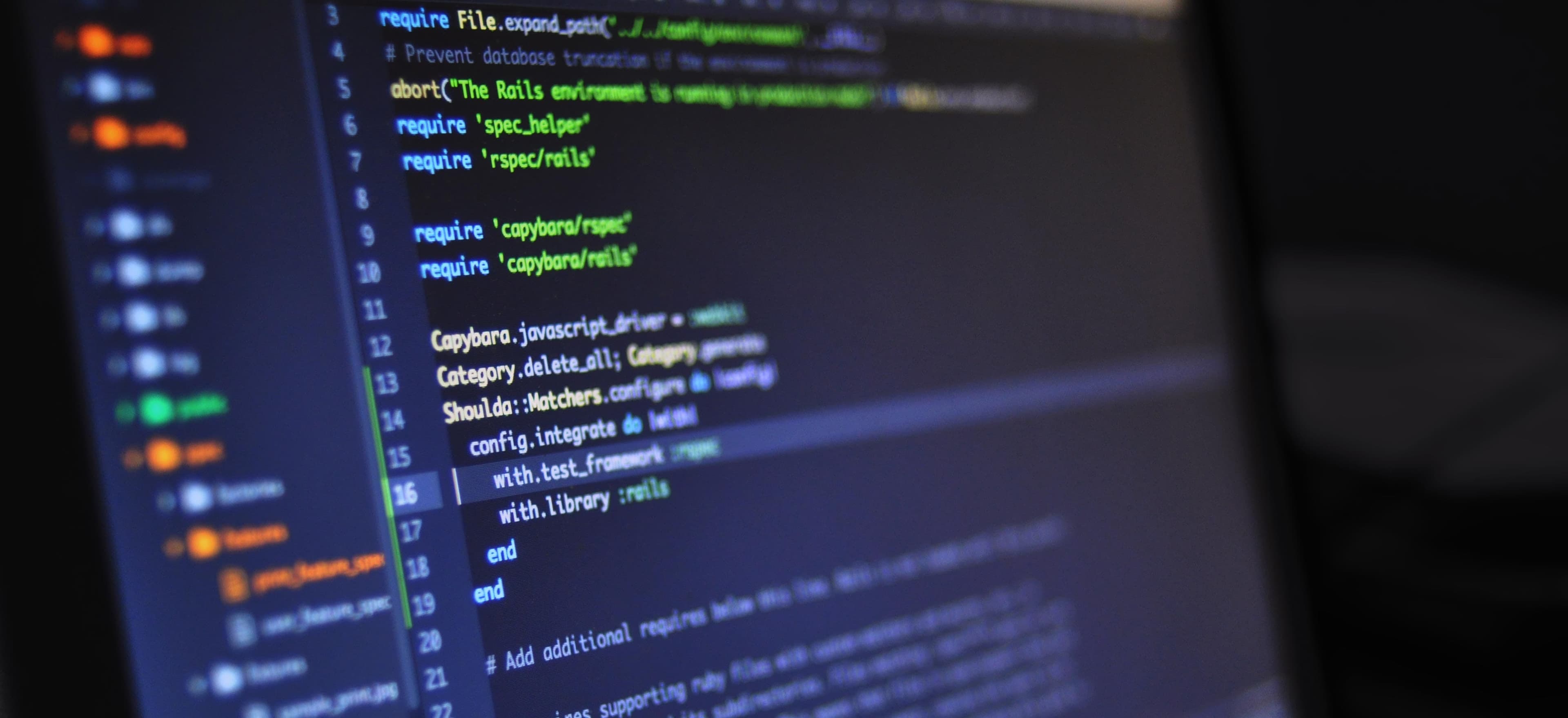
- Published on
Identifying and Managing Inactive Okta Users in Your Java Application
In any Java application that uses Okta for user management, it is crucial to keep track of user activity and manage inactive users effectively. Inactive users not only pose security risks but also clutter up your user database, making it difficult to identify active users. In this article, we will discuss how to identify and manage inactive Okta users in your Java application.
Identifying Inactive Users
The first step in managing inactive Okta users is to identify them. Okta provides APIs that allow you to retrieve user information, including the last login timestamp. By checking this timestamp, you can determine which users have been inactive for a specified period.
Let's take a look at a Java code snippet that retrieves Okta user information and identifies inactive users:
// Use Okta Java SDK to authenticate and retrieve user information
User user = oktaClient.getUser(userId);
// Get the last login timestamp of the user
Instant lastLogin = user.getLastLogin();
Instant now = Instant.now();
// Calculate the time difference to identify inactive users
Duration inactiveDuration = Duration.between(lastLogin, now);
// Define a threshold for inactive users (e.g., 90 days)
Duration threshold = Duration.ofDays(90);
if (inactiveDuration.compareTo(threshold) > 0) {
// The user is inactive
// Add the user to the list of inactive users or take appropriate action
}
In this code snippet, we use the Okta Java SDK to retrieve user information and calculate the duration of inactivity. We then compare this duration to a predefined threshold to identify inactive users.
Managing Inactive Users
Once you have identified the inactive users in your Okta system, you need to take appropriate action to manage them. This could involve notifying the users, disabling their accounts, or even deleting them from your user database, depending on your organization's policies.
Here's an example of how you can disable an inactive Okta user account using the Okta Java SDK:
// Use Okta Java SDK to disable the inactive user account
user.deactivate(); // or user.suspend()
// Save the changes
user.update();
In this code snippet, we use the deactivate
method provided by the Okta Java SDK to disable the inactive user account. We then call the update
method to save the changes to the user account.
Automating User Management
To effectively manage inactive Okta users, it is essential to automate the process. You can schedule a background job in your Java application to periodically check for inactive users and take the necessary actions.
Here's a simplified example using Spring's @Scheduled
annotation to create a scheduled task for managing inactive users:
@Component
public class UserManagementTask {
@Scheduled(cron = "0 0 0 * * *") // Run daily at midnight
public void manageInactiveUsers() {
// Logic to identify and manage inactive users
}
}
In this example, we create a Spring component with a method annotated with @Scheduled
to run daily at midnight. Inside the method, you can implement the logic to identify and manage inactive Okta users.
The Bottom Line
In conclusion, identifying and managing inactive Okta users in your Java application is essential for maintaining security and keeping your user database clean. By using the Okta Java SDK and implementing automation, you can effectively handle inactive users and ensure that your application remains secure and efficient.
To learn more about Okta user management in Java applications, you can refer to the Okta Java SDK documentation.
I hope this article helps you understand the importance of managing inactive Okta users and provides you with the necessary guidance to implement effective user management in your Java application.
Checkout our other articles