Mastering Scala: Unraveling Futures & Combinators Magic!
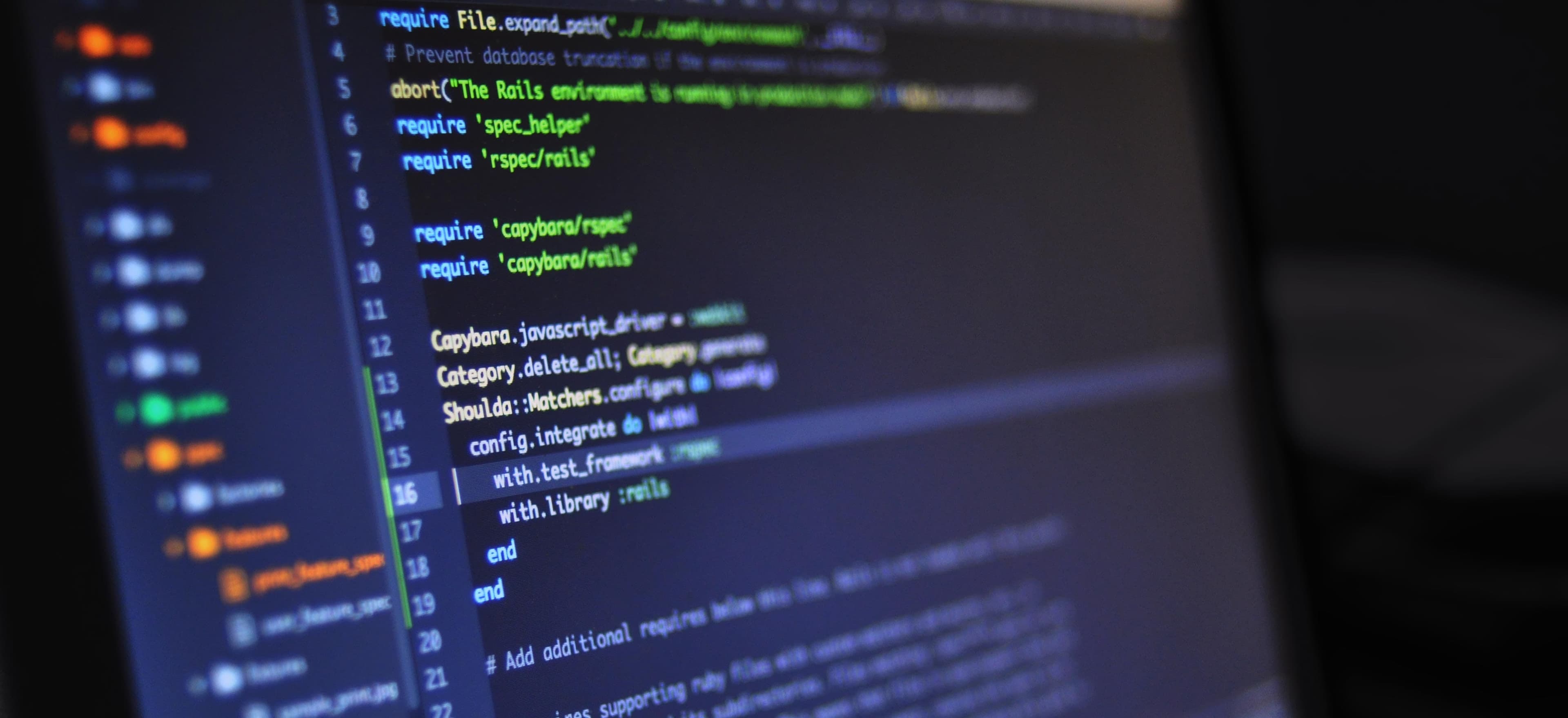
- Published on
Mastering Scala: Unraveling Futures & Combinators Magic!
Scala, a modern, multi-paradigm programming language, has gained immense popularity due to its concise syntax, functional programming capabilities, and compatibility with Java. In this article, we will dive deep into the world of Futures and Combinators in Scala, unraveling the magic behind these powerful abstractions.
Understanding Futures
In Scala, a Future
is a placeholder for a value that may be available at some point in the future. It represents a computation that may not have completed yet, allowing us to perform asynchronous and non-blocking operations. Let's consider a simple example to illustrate the concept of Future
:
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val futureResult: Future[Int] = Future {
// Simulating a time-consuming computation
Thread.sleep(1000)
42
}
In the above code snippet, we create a Future
that will eventually hold an Int
value. The computation inside the Future
simulates a time-consuming task by pausing execution for 1 second using Thread.sleep(1000)
and then producing the value 42
. By utilizing Future
, we can initiate asynchronous computations without blocking the main thread.
Why use Futures?
Futures are instrumental in building responsive and scalable applications, especially when dealing with I/O operations, network requests, or other blocking tasks. By leveraging Futures, we can execute multiple tasks concurrently, effectively utilizing system resources and improving overall system responsiveness.
Transforming Future Results with Combinators
Scala provides a rich set of combinators to manipulate and compose Future
results easily. Combinators are higher-order functions that enable us to perform operations on Future
instances in a declarative and concise manner.
Mapping Future Results
The map
combinator allows us to transform the result of a Future
using a provided function. Consider the following example:
val transformedFuture: Future[String] = futureResult.map(result => s"The answer is $result")
In this code snippet, the map
combinator is used to create a new Future
that holds a String
value constructed from the original Future
's result.
Chaining Future Computations
The flatMap
combinator is particularly useful for chaining dependent asynchronous computations. It enables us to sequence multiple Future
operations, where the result of one Future
determines the computation of the next. Let's take a look at an example:
val nextFuture: Future[Int] = futureResult.flatMap(result => Future(result * 2))
Here, we use flatMap
to create a new Future
that performs a computation based on the result of the original Future
.
Handling Multiple Futures
In scenarios where we need to aggregate results from multiple Future
instances, Scala provides the for
comprehension, which allows for concise and readable composition of asynchronous operations. Consider the following example:
val futureResult1: Future[Int] = Future { 10 }
val futureResult2: Future[Int] = Future { 20 }
val aggregatedResult: Future[Int] = for {
result1 <- futureResult1
result2 <- futureResult2
} yield result1 + result2
The for
comprehension elegantly combines the results of futureResult1
and futureResult2
into a new Future
, representing the sum of the two values.
Why use Combinators?
Combinators empower developers to express complex asynchronous workflows in a clear and concise manner. By leveraging combinators, we can avoid callback hell and nested structures, leading to more readable and maintainable code. Additionally, combinators facilitate error handling and composition of asynchronous operations, enhancing the overall robustness of the application.
Error Handling in Futures
Handling errors is a crucial aspect of asynchronous programming. Scala's Future
provides built-in mechanisms for error handling, ensuring that exceptions and failures are properly managed.
Handling Completion, Success, and Failure
We can use the onComplete
method to specify actions to be taken when a Future
completes, either successfully or with an error. For example:
futureResult.onComplete {
case Success(result) => println(s"The result is $result")
case Failure(exception) => println(s"An error occurred: ${exception.getMessage}")
}
The onComplete
method allows us to define separate behavior for successful completion and failure, enabling robust error management.
Dealing with Asynchronous Errors
When working with multiple concurrent Future
instances, Scala provides the Future.sequence
method to handle collections of Future
results. This method collects results from all the provided Future
instances, effectively aggregating them while managing errors. Consider the following example:
val futures: List[Future[Int]] = List(Future { 1 }, Future { 2 }, Future { throw new RuntimeException("Error!") })
val aggregatedResults: Future[List[Int]] = Future.sequence(futures)
In this example, Future.sequence
ensures that all the results are collected, even if some of the individual Future
instances encounter errors. This aids in consolidating the handling of asynchronous errors within a collection of Future
instances.
Why Focus on Error Handling?
By emphasizing robust error handling, developers can ensure the reliability and resilience of asynchronous operations. Proper error management in asynchronous code is essential for delivering stable and fault-tolerant applications, especially in systems handling concurrent and distributed operations.
The Last Word
In this exploration of Scala Futures and Combinators, we have uncovered the power and flexibility of asynchronous programming in Scala. By harnessing the potential of Future
and its combinators, developers can create responsive, scalable, and resilient applications capable of managing asynchronous operations effortlessly. The seamless integration of error handling further solidifies Scala's position as a preferred language for building high-performance, concurrent systems.
Mastering Scala's Futures and Combinators unlocks a realm of possibilities for developers, allowing them to tackle complex asynchronous workflows with elegance and precision. Asynchronous programming is an integral part of modern application development, and Scala equips developers with the tools to excel in this domain.
Embrace the magic of Futures and Combinators in Scala, and elevate your proficiency in building sophisticated, concurrent applications!
Whether you are an experienced Scala developer or looking to venture into the world of asynchronous programming, mastering Futures and Combinators in Scala will undoubtedly broaden your horizons and elevate your skill set.
Make the most of Scala’s asynchronous potential by mastering Futures and Combinators! Harness the power of concurrent programming and create robust, responsive applications with Scala. Dive into the world of Futures and Combinators to unlock a new realm of possibilities!
Checkout our other articles