Taming Complexity: Reactive Architecture in Functional Programming
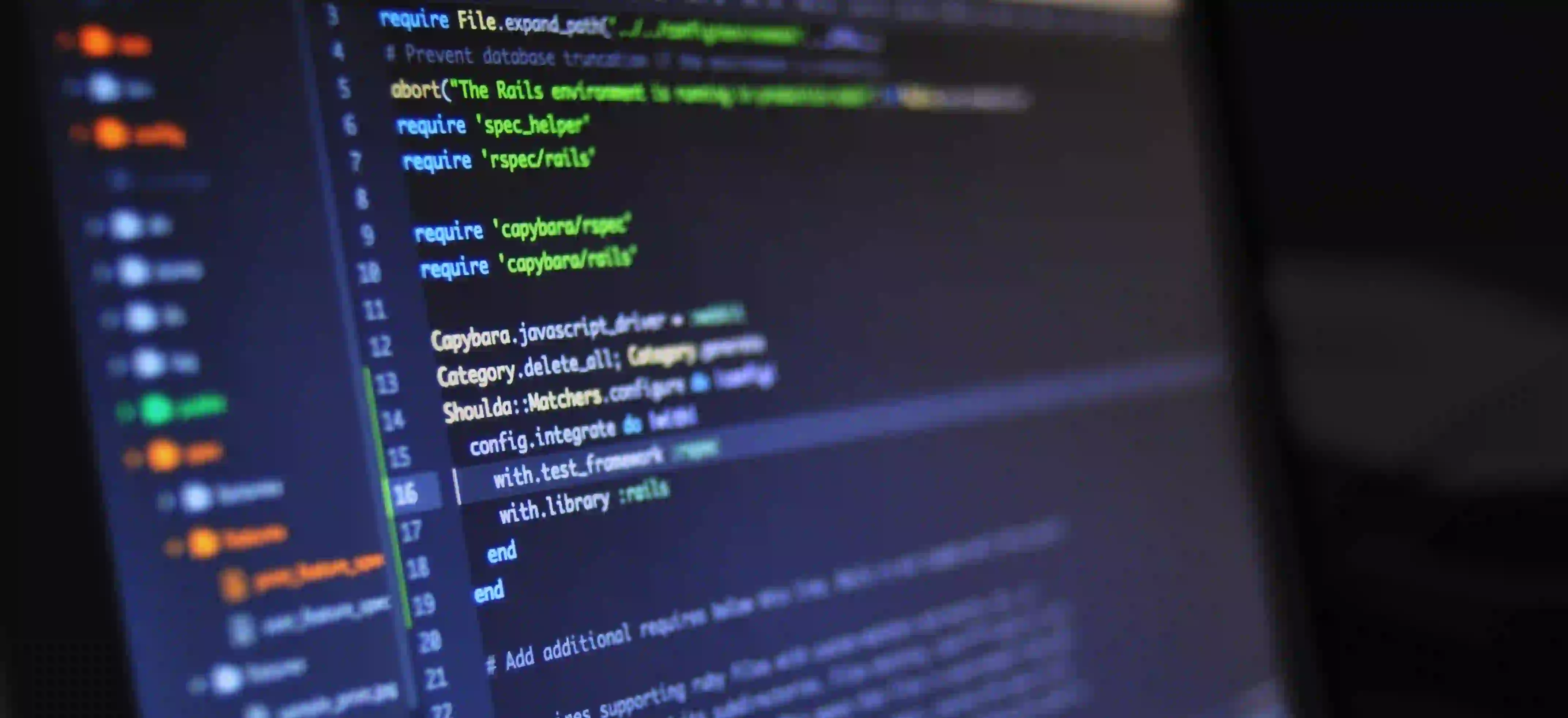
Taming Complexity: Reactive Architecture in Functional Programming
In the world of software development, managing complexity is a critical challenge. As systems grow in size and scope, the need to maintain, extend, and reason about the code becomes increasingly difficult. In this regard, reactive architecture offers a compelling approach to handle the intricacies of modern, distributed systems. When combined with the principles of functional programming, it provides a potent framework for dealing with complexity while retaining scalability, resilience, and responsiveness.
Understanding Reactive Architecture
Reactive architecture is centered around building systems that are responsive, resilient, elastic, and message-driven. This approach is particularly well-suited for modern, distributed systems that handle a high volume of concurrent users and data. At its core, reactive architecture embraces the principles of event-driven and asynchronous processing, enabling systems to react to changes and events in a timely manner.
The Reactive Manifesto
The Reactive Manifesto, a foundational document in the realm of reactive systems, outlines the key principles that define reactive architecture. It emphasizes the following four traits:
-
Responsive: Systems should respond promptly in the face of user interactions and handle high workloads without sacrificing responsiveness.
-
Resilient: Systems should remain responsive in the presence of failures, gracefully recovering from any faults.
-
Elastic: Systems should be able to scale and allocate resources as demand fluctuates.
-
Message-Driven: Communication within systems should be asynchronous, relying on message-passing to decouple components.
Leveraging Functional Programming in Reactive Architecture
Functional programming complements reactive architecture exceptionally well. By emphasizing immutability, pure functions, and declarative style, functional programming fosters code that is easier to reason about, test, and maintain. Additionally, functional programming languages such as Scala and Clojure provide robust support for asynchronous and concurrent programming, aligning with the requirements of reactive systems.
Immutability and Pure Functions
In functional programming, immutability is a core tenet. By ensuring that data remains unchanged once created, immutability simplifies reasoning about code and eliminates a wide array of potential bugs stemming from mutable state. Pure functions, which produce the same output for a given input and have no side effects, further contribute to code predictability and testability.
// Immutable data class in Java
public final class ImmutableData {
private final int id;
private final String name;
public ImmutableData(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
// No setters, ensuring immutability
}
Declarative Style and Asynchronous Operations
Functional programming encourages a declarative, expressive style that focuses on what needs to be done rather than how to do it. This aligns seamlessly with the nature of asynchronous operations in reactive architecture, where the order of execution may not be predetermined. Leveraging constructs such as Java's CompletableFuture or Scala's Future, functional programming enables the concise expression of asynchronous, non-blocking computations.
// Example of asynchronous operation using CompletableFuture in Java
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> "Hello")
.thenApplyAsync(result -> result + " World")
.thenApplyAsync(result -> result.toUpperCase());
future.thenAcceptAsync(System.out::println);
Integration with Reactive Libraries and Frameworks
Functional programming languages often feature robust libraries and frameworks that support reactive programming paradigms. For instance, Akka, a popular toolkit for building highly concurrent, distributed, and resilient message-driven applications in Scala, aligns closely with the principles of reactive architecture. The Actor model, a fundamental concept in Akka, embodies the message-driven nature of reactive systems, facilitating the construction of responsive and resilient applications.
Bringing It All Together
In the realm of modern software development, the challenge of managing complexity looms large. Reactive architecture, with its emphasis on responsiveness, resilience, elasticity, and message-driven communication, offers a compelling framework to address this challenge. When integrated with the principles of functional programming, which promote immutability, pure functions, and declarative style, it becomes a potent combination for taming complexity in distributed systems. By leveraging the strengths of both reactive architecture and functional programming, developers can build systems that exhibit high levels of responsiveness, scalability, and maintainability, even in the face of considerable complexity and change.
As complexity in software systems continues to increase, the marriage of reactive architecture and functional programming stands as a promising path forward, enabling developers to navigate and conquer the challenges of modern, distributed computing.
For further reading on reactive architecture and functional programming, Reactive Manifesto and Functional Programming in Java are valuable resources to delve deeper into these topics.