Easy Ways to Test Java 8 Functions
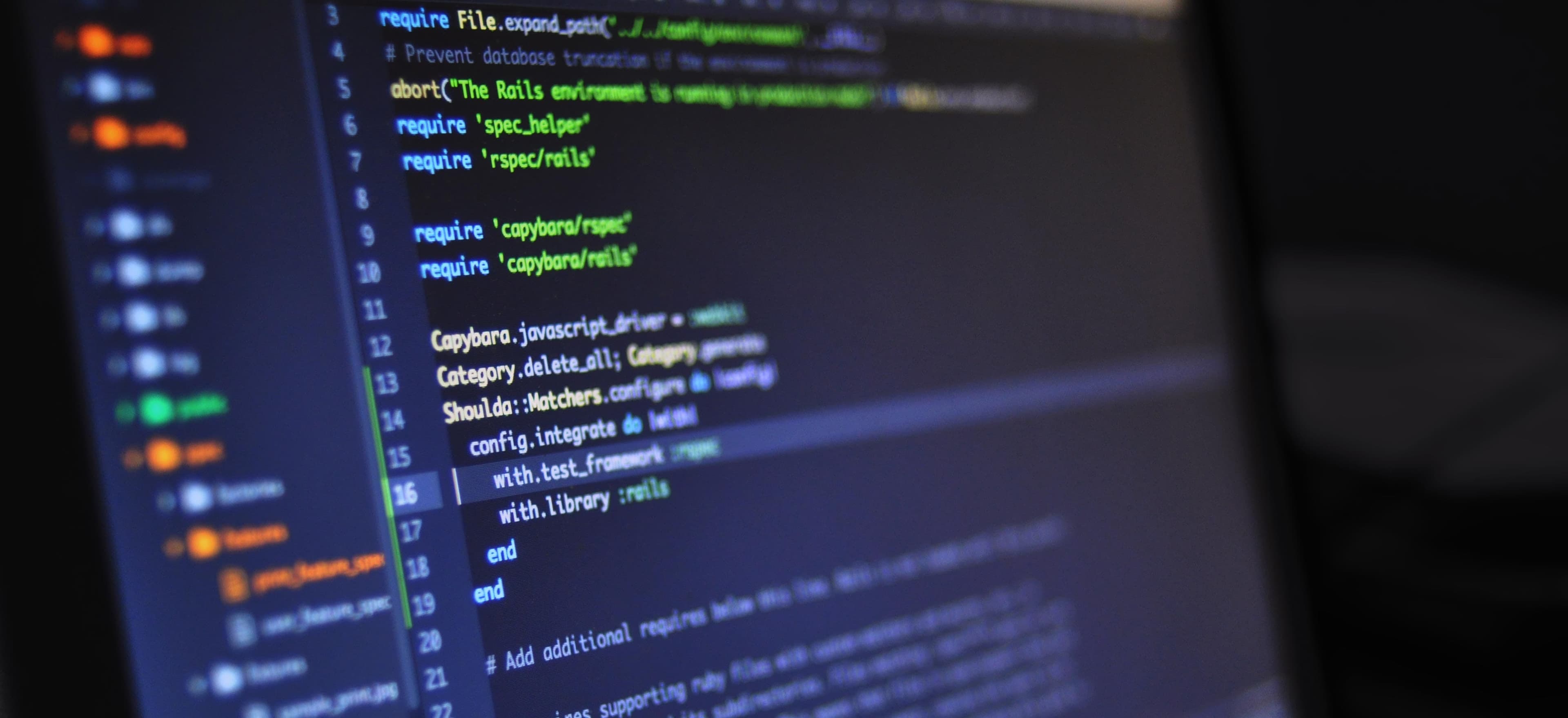
- Published on
Testing Java 8 Functions: A Comprehensive Guide
When it comes to Java programming, testing functions is a crucial aspect of ensuring the reliability and robustness of the code. With the advent of Java 8, which introduced functional programming features such as lambda expressions and the java.util.function
package, testing functions has become more streamlined and efficient.
In this article, we will delve into the various strategies and best practices for testing Java 8 functions. From unit testing simple functions to testing complex functional interfaces, we will explore easy and effective ways to ensure the correctness of your Java 8 functions.
Setting Up the Environment
Before we dive into testing Java 8 functions, we need to ensure that our development environment is properly set up. For the purpose of this article, we will assume that you have a basic understanding of Java development and are familiar with a build tool such as Maven or Gradle.
Firstly, make sure to have Java 8 or later installed on your machine. Additionally, integrate a testing framework such as JUnit or TestNG into your project. These frameworks will provide the necessary tools for writing and executing tests for your Java 8 functions.
Unit Testing Simple Functions
Let’s start by considering a simple scenario where we have a basic function implemented using a lambda expression. Our goal is to test this function to ensure it behaves as expected.
import java.util.function.Function;
public class SimpleFunctionExample {
public static void main(String[] args) {
// Simple function that doubles the input
Function<Integer, Integer> doubleFunction = (number) -> number * 2;
// Test the function
System.out.println(doubleFunction.apply(5)); // Output: 10
}
}
In this scenario, we have a simple Function
that doubles the input integer. To test this function, we can create a unit test using JUnit.
import org.junit.jupiter.api.Test;
import java.util.function.Function;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class SimpleFunctionExampleTest {
@Test
void testDoubleFunction() {
Function<Integer, Integer> doubleFunction = (number) -> number * 2;
assertEquals(Integer.valueOf(10), doubleFunction.apply(5));
}
}
In the test case above, we assert that the function correctly doubles the input integer. This is a straightforward example of unit testing a simple Java 8 function using JUnit.
Testing Functional Interfaces
When working with functional interfaces such as Predicate
, Consumer
, Supplier
, or UnaryOperator
, it is essential to ensure that their behavior aligns with the intended functionality. Let’s consider testing a Predicate
that checks whether a given string is of even length.
import java.util.function.Predicate;
public class PredicateExample {
public static void main(String[] args) {
// Predicate to check even length of a string
Predicate<String> isEvenLength = s -> s.length() % 2 == 0;
// Test the predicate
System.out.println(isEvenLength.test("java")); // Output: true
System.out.println(isEvenLength.test("testing")); // Output: false
}
}
To test this Predicate
, we can create a unit test as follows:
import org.junit.jupiter.api.Test;
import java.util.function.Predicate;
import static org.junit.jupiter.api.Assertions.assertTrue;
import static org.junit.jupiter.api.Assertions.assertFalse;
public class PredicateExampleTest {
@Test
void testIsEvenLength() {
Predicate<String> isEvenLength = s -> s.length() % 2 == 0;
assertTrue(isEvenLength.test("java"));
assertFalse(isEvenLength.test("testing"));
}
}
In the test case above, we verify that the isEvenLength
predicate correctly identifies strings with even length. By testing these functional interfaces, we can gain confidence in their reliability and suitability for our applications.
Integration Testing with Functional Interfaces
In certain cases, functional interfaces are utilized within the context of larger components or systems. To test the integration of functional interfaces with other components, we can employ integration testing techniques.
Consider a scenario where a Function
is used to transform a list of integers. We want to ensure that the transformation produces the expected results within the broader context of the application.
import java.util.List;
import java.util.Arrays;
import java.util.function.Function;
public class FunctionIntegrationExample {
public static void main(String[] args) {
// Function to square the input integer
Function<Integer, Integer> squareFunction = (number) -> number * number;
// Transform the list of integers
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.replaceAll(squareFunction::apply);
// Output the transformed list
System.out.println(numbers); // Output: [1, 4, 9, 16, 25]
}
}
In an integration test, we can verify that the transformation function works correctly within the given context.
import org.junit.jupiter.api.Test;
import java.util.List;
import java.util.Arrays;
import java.util.function.Function;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class FunctionIntegrationExampleTest {
@Test
void testSquareFunctionIntegration() {
Function<Integer, Integer> squareFunction = (number) -> number * number;
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.replaceAll(squareFunction::apply);
assertEquals(Arrays.asList(1, 4, 9, 16, 25), numbers);
}
}
Integration testing ensures that the function interacts correctly within the context of the broader application, providing confidence in its functionality and integration.
Mocking Functional Interfaces
In some cases, functional interfaces may depend on external resources or services, making it challenging to test their behavior in isolation. This is where mocking frameworks, such as Mockito, come into play.
Imagine a scenario where a Consumer
is responsible for writing data to a file. To test the behavior of the Consumer
, we can mock the file writing operation using Mockito.
import java.util.function.Consumer;
import java.io.FileWriter;
import java.io.IOException;
public class FileConsumerExample {
private FileWriter fileWriter;
public FileConsumerExample(FileWriter fileWriter) {
this.fileWriter = fileWriter;
}
public void writeData(String data) {
Consumer<String> fileWriterConsumer = fileWriter::write;
fileWriterConsumer.accept(data);
}
}
In the corresponding test case, we utilize Mockito to mock the file writing operation and verify that the Consumer
behaves as expected.
import org.junit.jupiter.api.Test;
import java.io.FileWriter;
import java.io.IOException;
import java.util.function.Consumer;
import static org.mockito.Mockito.*;
public class FileConsumerExampleTest {
@Test
void testWriteData() throws IOException {
FileWriter fileWriter = mock(FileWriter.class);
FileConsumerExample fileConsumer = new FileConsumerExample(fileWriter);
String testData = "Sample data";
fileConsumer.writeData(testData);
verify(fileWriter, times(1)).write(testData);
}
}
Mocking allows us to isolate the behavior of the functional interface and focus on its specific functionality, enabling thorough testing in scenarios where external dependencies are involved.
Closing the Chapter
Testing Java 8 functions, whether simple or complex, is essential for guaranteeing the reliability and performance of your code. By employing diverse testing techniques such as unit testing, integration testing, and mocking, you can ensure that your Java 8 functions behave as expected in a variety of scenarios.
To further enhance your understanding of testing Java 8 functions, consider delving into in-depth resources such as Oracle's official documentation and practical tutorials from well-established platforms like Baeldung for comprehensive insights and best practices.
In conclusion, with a robust testing strategy in place, you can confidently develop and maintain Java 8 functions that meet the highest standards of quality and reliability.
Checkout our other articles