24/7 Mocking Challenge: Avoiding Test Data Nightmares
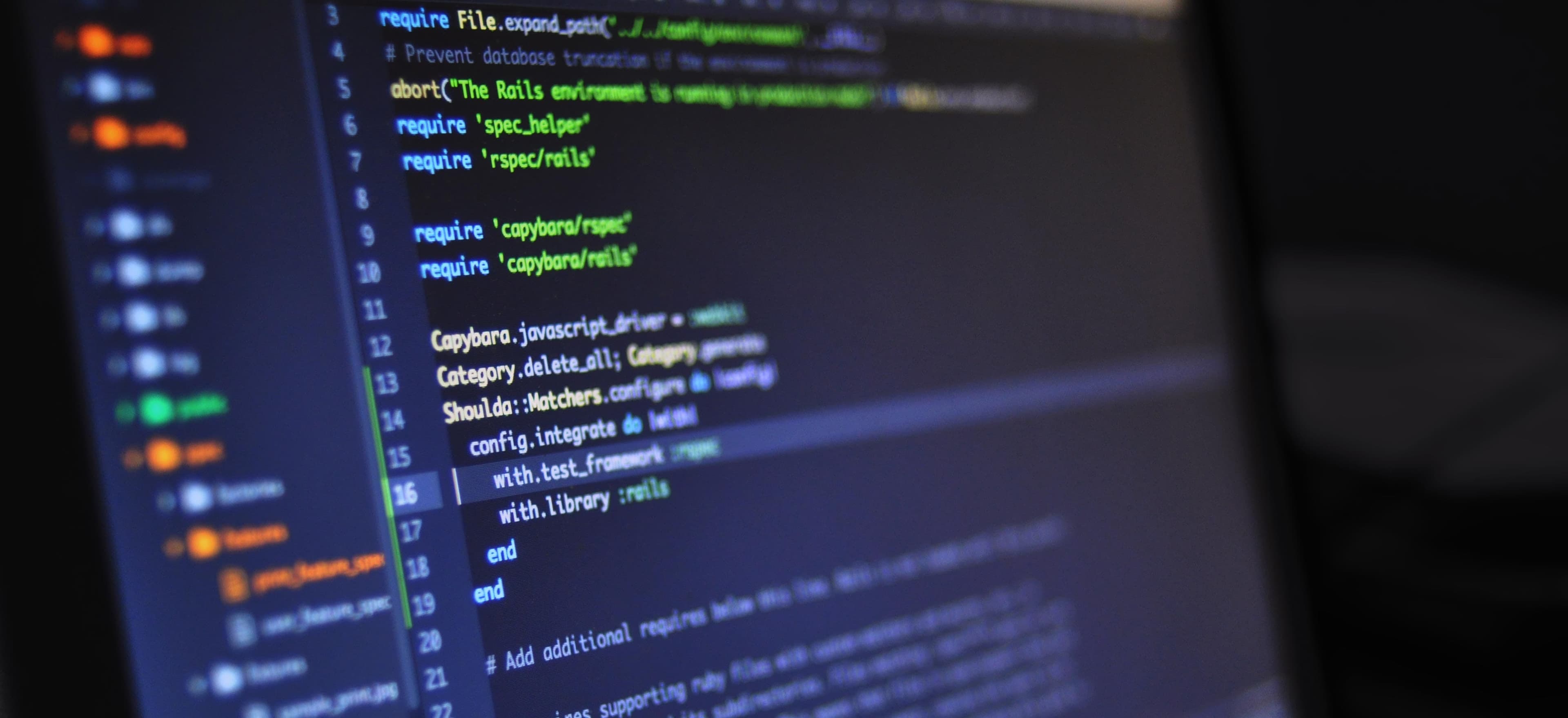
- Published on
The 24/7 Mocking Challenge: Avoiding Test Data Nightmares
Testing Java code is an essential part of the software development process. However, creating efficient and reliable tests often requires a lot of effort, especially when it comes to managing test data. This is where mocking comes into play. In this blog post, we will explore the concept of mocking in Java, its significance in testing, and how it can help you avoid test data nightmares.
What is Mocking?
Mocking is a technique used in unit testing to isolate a section of code under test and validate its behavior. This is achieved by creating objects that simulate the behavior of real objects, thus allowing the tested code to run in an isolated environment.
In Java, popular mocking frameworks like Mockito and EasyMock provide the necessary tools to create these mock objects and define their behavior, enabling developers to focus on testing the specific functionality of their code.
The Significance of Mocking in Testing
Mocking plays a crucial role in testing for several reasons:
-
Isolation: Mocking allows developers to test individual components of a system in isolation, ensuring that the behavior of one component does not interfere with others. This isolation helps in identifying and fixing bugs more effectively.
-
Controlled Environment: With mocking, developers can set up a controlled environment for the code under test, ensuring that external dependencies do not impact the test results. This is particularly important when testing complex systems involving external resources like databases, web services, or third-party APIs.
-
Predictable Behavior: By defining the behavior of mock objects, developers can create predictable test scenarios, leading to more reliable tests. This predictability is essential for ensuring that the code behaves as expected under various conditions.
Implementing Mocking with Mockito
Let's delve into an example to understand how mocking, using the Mockito framework, can alleviate test data nightmares.
Consider a scenario where we have a UserService
class that interacts with a UserRepository
to perform operations such as fetching user details. We want to test the UserService
class without involving the actual database interactions.
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public String getUserDetails(String userId) {
// Business logic for fetching user details
User user = userRepository.getUserById(userId);
return "Details for user " + user.getName();
}
}
In a traditional approach, testing UserService
would involve setting up a test database, populating it with test data, and ensuring that the test data remains consistent across different test runs. This not only introduces complexity but also slows down the testing process.
However, with Mockito, we can create a mock UserRepository
and define its behavior for the test scenario. Here's how we can achieve this:
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUserDetails() {
UserRepository mockUserRepository = mock(UserRepository.class);
UserService userService = new UserService(mockUserRepository);
// Define the behavior of the mock repository
User testUser = new User("123", "John Doe");
when(mockUserRepository.getUserById("123")).thenReturn(testUser);
// Perform the test
String userDetails = userService.getUserDetails("123");
// Validate the result
assertEquals("Details for user John Doe", userDetails);
}
}
In this example, we created a mock UserRepository
using Mockito's mock
method. We then defined the behavior of the mock repository using the when
and thenReturn
methods to simulate the getUserById
call. This approach allows us to test the UserService
class without the need for a real database, making the test data management significantly easier and more efficient.
Best Practices for Effective Mocking
While mocking can greatly simplify the testing process, it's important to adhere to best practices to ensure the effectiveness of your tests:
-
Focus on Behavior, Not Implementation: When using mocking frameworks, focus on testing the behavior of the code rather than its implementation details. This ensures that tests remain resilient to refactoring and changes in the internal implementation.
-
Keep Mocking Minimal: Avoid over-mocking by only mocking the necessary dependencies for the test scenario. Over-mocking can lead to brittle tests that are tightly coupled to the implementation details, increasing maintenance overhead.
-
Use Mocking with Integration Tests: While mocking is valuable for unit tests, it should be complemented with integration tests that validate the interaction of real components, ensuring the overall system behavior.
-
Update Mocks When Behaviors Change: As the codebase evolves, ensure that the behaviors defined in mock objects are updated to reflect the changes. Outdated behaviors can lead to misleading test results.
Bringing It All Together
In the world of Java testing, mocking plays a pivotal role in simplifying test data management and ensuring the reliability of tests. By using mocking frameworks like Mockito, developers can create isolated and predictable test environments, ultimately avoiding test data nightmares and enabling faster, more effective testing.
Mocking is a powerful concept that, when used effectively, empowers developers to write robust and maintainable tests, leading to higher quality software. Embrace the art of mocking, and say goodbye to test data nightmares! Happy mocking!
To explore more about Mockito and advanced mocking techniques, check out the official documentation at Mockito Official Documentation.
Do you have experiences or insights to share about mocking in Java testing? We'd love to hear your thoughts in the comments below!
Checkout our other articles