Handling Complex Cases with javax.ws.rs.core.Context
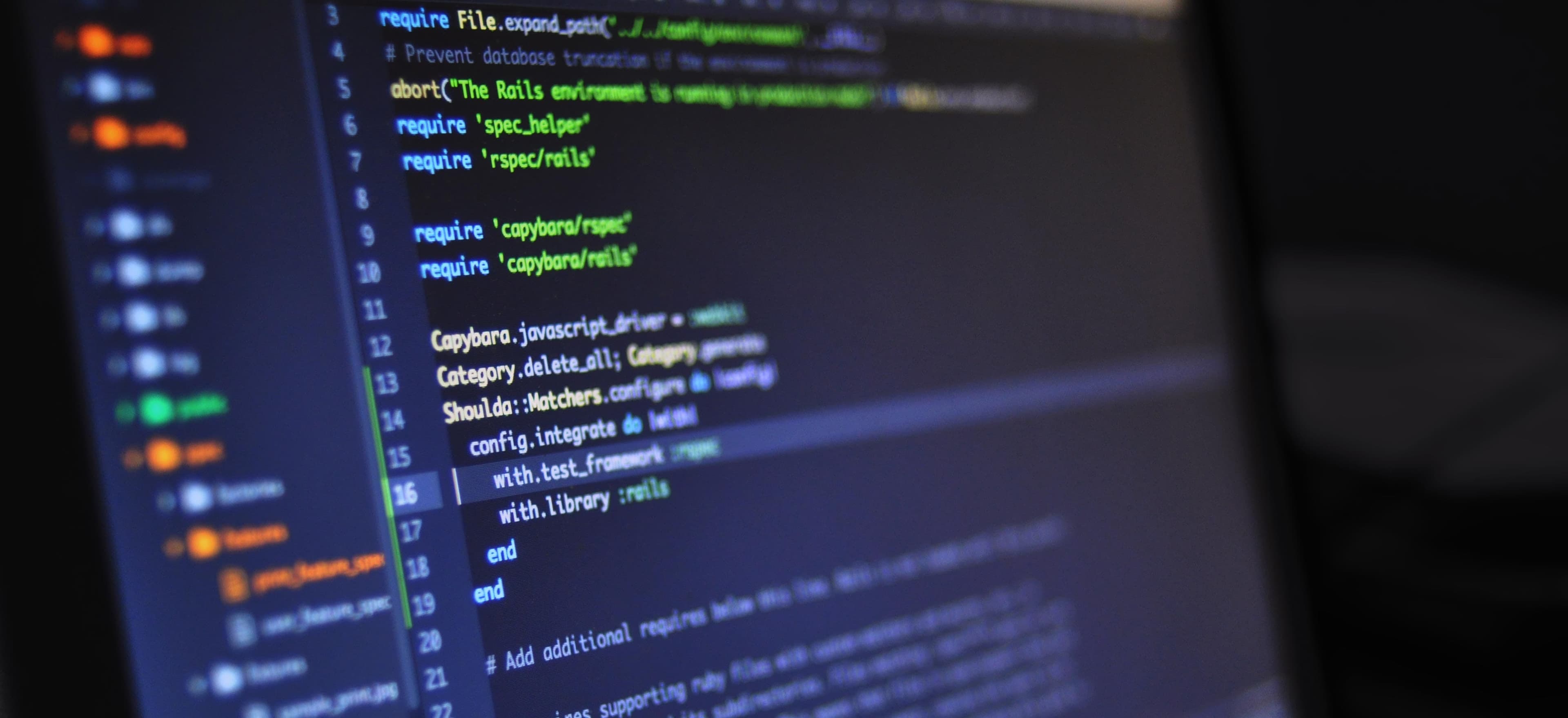
- Published on
Handling Complex Cases with javax.ws.rs.core.Context
In the world of Java web development, the javax.ws.rs
package plays a significant role in creating RESTful web services. It provides the necessary classes and interfaces for building APIs that abide by the principles of Representational State Transfer (REST). One of the key components of javax.ws.rs
is the javax.ws.rs.core.Context
annotation, which allows developers to inject various contextual elements into their resource classes, providers, or client filters.
Understanding javax.ws.rs.core.Context
The javax.ws.rs.core.Context
annotation is used to inject contextual objects into JAX-RS components. These contextual objects can include things like HttpServletRequest
, HttpServletResponse
, UriInfo
, SecurityContext
, and more. By using the @Context
annotation, you can access these objects within your resource methods without the need for manual instantiation or management.
Practical Use Cases
Let's delve into some practical scenarios where the @Context
annotation can be incredibly useful.
1. Accessing the Request and Response Objects
@GET
@Path("/example")
public Response exampleMethod(@Context HttpServletRequest request, @Context HttpServletResponse response) {
// Use the request and response objects here
// ...
return Response.ok("Success").build();
}
In the above example, HttpServletRequest
and HttpServletResponse
objects are injected using the @Context
annotation. This allows you to access and manipulate the request and response objects within your JAX-RS resource method.
2. Obtaining URI Information
@GET
@Path("/resource/{id}")
public Response getResource(@PathParam("id") int resourceId, @Context UriInfo uriInfo) {
// Use the uriInfo object to retrieve URI-related information
// ...
return Response.ok("Resource Retrieved").build();
}
In this scenario, UriInfo
is injected using the @Context
annotation, enabling you to obtain URI-specific details such as query parameters, path parameters, and more.
3. Managing Security Context
@GET
@Path("/secure")
@RolesAllowed("ADMIN")
public Response getSecureResource(@Context SecurityContext securityContext) {
// Check user roles using the securityContext object
// ...
return Response.ok("Authenticated").build();
}
Here, the SecurityContext
object is injected via the @Context
annotation, allowing you to access security-related information such as the authenticated user, their roles, and other security details.
Best Practices
While using @Context
can greatly simplify access to contextual objects, it's important to bear in mind some best practices to ensure clean and maintainable code.
1. Use @Context
Sparingly
While the @Context
annotation can be handy, overusing it can lead to tightly coupled and less testable code. Consider using constructor injection or method parameters for dependencies that are essential for testing and mocking.
2. Document Your Dependencies
It's crucial to document the dependencies of your resource methods. Clearly specify which contextual objects are being injected using the @Context
annotation, as this information is vital for fellow developers and maintainers.
3. Maintain Cohesion
Ensure that the contextual objects injected via @Context
are directly related to the functionality being implemented within the resource method. Mixing unrelated contextual objects can lead to confusion and reduced code clarity.
Wrapping Up
The javax.ws.rs.core.Context
annotation is a powerful tool for simplifying the access to contextual objects within JAX-RS components. By leveraging this annotation effectively, you can streamline your code and improve the maintainability of your RESTful web services.
In conclusion, although the @Context
annotation provides a convenient way to access contextual objects, it should be used judiciously and accompanied by clear documentation to promote code transparency and maintainability.
For more in-depth understanding, detailed documentation for javax.ws.rs.core.Context
can be found in the official Java EE documentation.
So, make the most of @Context
and simplify your code for a better development experience!
Checkout our other articles