Securing the Future: Navigating Big Data Vulnerabilities
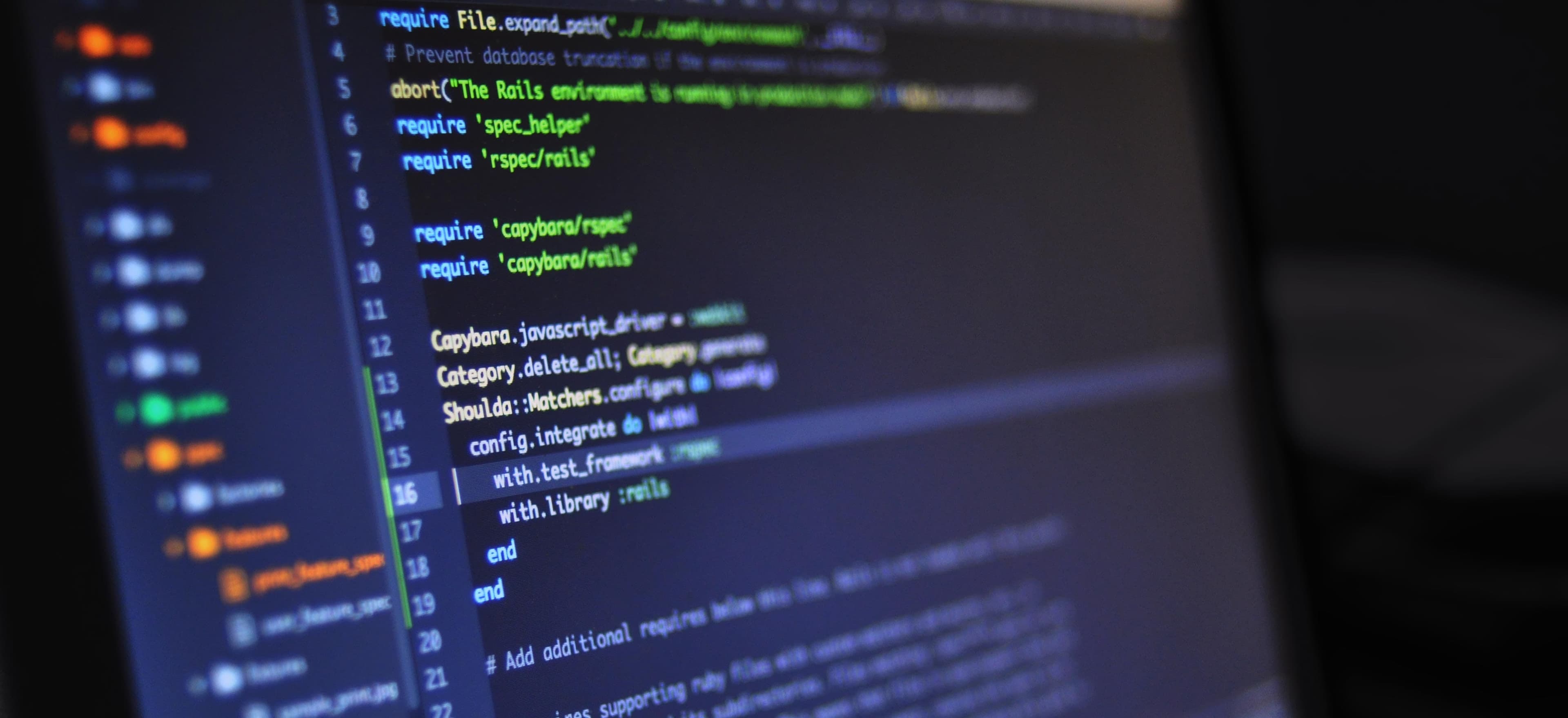
- Published on
Securing the Future: Navigating Big Data Vulnerabilities
In an era where data is the new fuel powering our world, it's imperative to acknowledge the significance of securing it. With the exponential growth of big data, the need to address vulnerabilities becomes all the more crucial. As a Java developer, understanding and effectively implementing security measures is paramount. In this article, we'll delve into the realm of big data vulnerabilities and how Java can be used to fortify our defenses.
Understanding Big Data Vulnerabilities
Big data vulnerabilities encompass a broad spectrum of potential security risks. These vulnerabilities can include unauthorized access to sensitive information, data breaches, insider threats, and the exploitation of software flaws. As the volume, variety, and velocity of big data increase, so too do the avenues for exploitation. Therefore, it's essential for developers to adopt a proactive approach to security.
Common Vulnerabilities
-
Injection Attacks: As with traditional databases, big data systems are susceptible to SQL injection attacks. Unsanitized user inputs can lead to malicious code execution, compromising the integrity of the data.
-
Inadequate Authentication and Authorization: Weak authentication mechanisms can expose big data systems to unauthorized access and potential data manipulation. Without robust authorization controls, sensitive data is at risk.
-
Data Privacy and Protection: With vast amounts of personal and sensitive information being stored within big data systems, ensuring compliance with privacy regulations and safeguarding data from unauthorized access are critical concerns.
Leveraging Java for Security
Java, renowned for its robust security features, is a powerful tool for addressing big data vulnerabilities. Through its extensive libraries, APIs, and security frameworks, Java enables developers to implement stringent security measures.
Secure Coding Practices
Adhering to secure coding practices is fundamental in mitigating big data vulnerabilities. Utilizing techniques such as input validation, proper error handling, and secure authentication mechanisms can significantly enhance the security posture of a Java application.
Let's take a look at an example of input validation in Java:
public class InputValidationExample {
public boolean isValidInput(String input) {
// Validate the input to prevent potential injection attacks
// Implement validation logic here
}
}
In the above code snippet, the isValidInput
method demonstrates a basic input validation check to mitigate the risk of injection attacks. By validating user input, developers can preemptively thwart potential security threats.
Encryption and Decryption
Data encryption is an indispensable component of data security. Java provides robust support for encryption and decryption through libraries like Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE). By incorporating encryption algorithms and secure key management, developers can safeguard sensitive data from unauthorized access.
Let's consider a simple example of data encryption in Java:
public class DataEncryptionExample {
public byte[] encryptData(String data, Key encryptionKey) {
// Implement data encryption using encryptionKey
// Return the encrypted data
}
public String decryptData(byte[] encryptedData, Key decryptionKey) {
// Implement data decryption using decryptionKey
// Return the decrypted data
}
}
In the above code snippet, the encryptData
and decryptData
methods showcase the encryption and decryption of data using cryptographic keys in Java. By leveraging encryption, developers can bolster the confidentiality and integrity of data stored in big data systems.
Role-Based Access Control
Implementing role-based access control (RBAC) mechanisms is pivotal in regulating access to big data systems. Java provides robust support for RBAC through frameworks like Spring Security, enabling developers to enforce fine-grained access policies based on user roles and permissions.
Here's a simplified illustration of RBAC implementation in Java using Spring Security:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("ADMIN", "USER")
.and()
.formLogin();
}
}
In the above code snippet, the SecurityConfig
class demonstrates the configuration of role-based access control using Spring Security. By defining access restrictions based on user roles, Java empowers developers to enforce comprehensive access policies within big data applications.
The Road Ahead: Embracing a Security-Centric Mindset
As the big data landscape continues to evolve, the importance of prioritizing security cannot be overstated. Java serves as an indispensable ally in fortifying big data systems against vulnerabilities. By leveraging Java's robust security features, developers can proactively address potential risks and uphold the integrity of data.
In conclusion, the fusion of Java and stringent security practices is pivotal in navigating big data vulnerabilities and shaping a more secure future. Embracing a security-centric mindset from the outset of development is not just a best practice—it's an imperative for safeguarding the invaluable asset that is big data.
As we traverse the ever-expanding terrain of big data, let's fortify our foundations with the resilient shield that Java provides, ensuring that our data remains secure and our future remains unassailable.
Remember, the path to a secure future begins with a single line of code.
For more information on big data security, refer to OWASP's Top Ten Big Data Security Risks and Oracle's Java Security Overview.
Keep coding securely!