Streamlining Log Analysis: Overcoming Data Overload
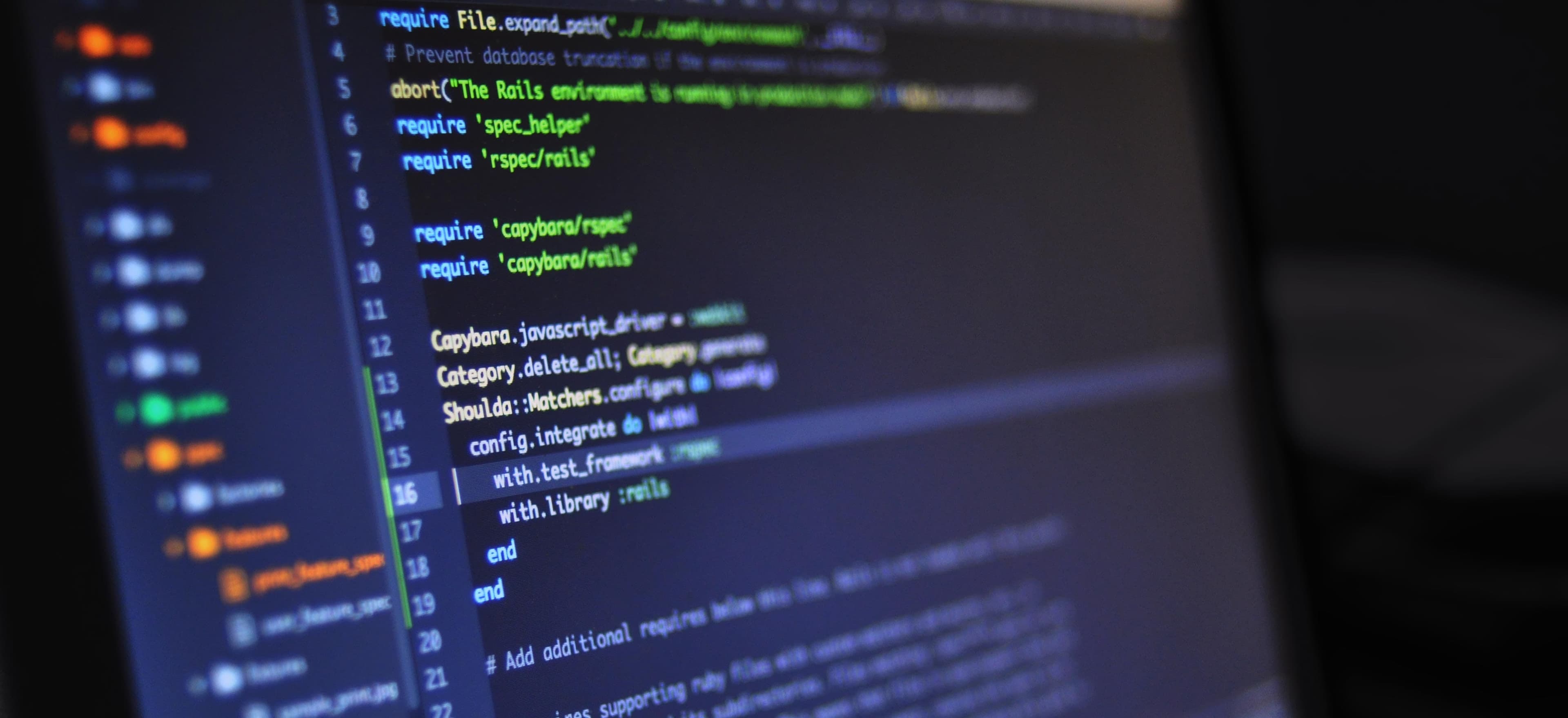
- Published on
Streamlining Log Analysis: Overcoming Data Overload
In the world of software development and operations, log analysis plays a crucial role in understanding the behavior and performance of applications and infrastructure. However, as systems grow more complex, the volume of log data can easily become overwhelming. In this article, we'll discuss how Java developers can streamline log analysis to gain actionable insights from the deluge of log information.
Understanding the Challenge
Logs are a treasure trove of information, containing valuable insights into system behavior, errors, and performance metrics. However, as the scale of systems and applications grows, so does the volume of log data. Manually sifting through this data to identify issues and trends becomes impractical, if not impossible.
Enter Log Analysis with Java
Java, with its robust ecosystem and powerful libraries, offers several tools and techniques to tackle log analysis challenges. From parsing and filtering to advanced analytics, Java empowers developers to extract meaningful information from log data efficiently.
Leveraging Log4j for Efficient Logging
Log4j is a popular logging framework in the Java ecosystem, known for its flexibility and performance. By utilizing Log4j, developers can configure different log levels, appenders, and layouts to tailor the logging output according to the specific needs of their application.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class MyClass {
private static final Logger logger = LogManager.getLogger(MyClass.class);
public void performTask() {
// Perform the task
logger.debug("Task performed successfully");
}
}
In the above example, the Logger
instance from Log4j is used to log a debug message when a task is performed. This allows developers to categorize log messages based on their severity and control the verbosity of the log output.
Parsing and Structuring Log Data
One of the key challenges in log analysis is parsing unstructured log data into a structured format that allows for easier querying and analysis. Libraries such as Logstash and Jackson can be employed to parse and structure log data in Java.
import com.fasterxml.jackson.databind.ObjectMapper;
public class LogParser {
private ObjectMapper objectMapper = new ObjectMapper();
public LogEntry parseLog(String logLine) {
try {
return objectMapper.readValue(logLine, LogEntry.class);
} catch (IOException e) {
// Handle parsing errors
}
}
}
In the above code snippet, the Jackson library is utilized to parse log data into a LogEntry
object, enabling easier manipulation and querying of log information.
Using Elasticsearch for Log Storage and Analysis
Log storage and analysis are pivotal components of effective log management. Elasticsearch, with its scalable and distributed nature, coupled with the power of the Lucene query language, provides an ideal platform for storing and analyzing log data at scale.
By integrating Java applications with Elasticsearch, developers can index log data efficiently and execute complex queries to extract valuable insights from the logs.
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.common.xcontent.XContentType;
public class ElasticsearchService {
private RestHighLevelClient client;
public void indexLog(String index, String log) {
IndexRequest request = new IndexRequest(index)
.source(log, XContentType.JSON);
try {
client.index(request);
} catch (IOException e) {
// Handle indexing errors
}
}
}
The above code exemplifies how a Java application can utilize the Elasticsearch high-level client to index log data in a specified index within an Elasticsearch cluster, paving the way for subsequent analysis and visualization.
Tackling Log Analysis Challenges with Machine Learning
As log data continues to grow in volume and complexity, traditional analysis and monitoring approaches might fall short in uncovering hidden patterns and anomalies. This is where machine learning techniques, particularly anomaly detection algorithms, can be applied to identify irregular patterns within log data.
Apache Flink and Apache Spark are prominent Java-based frameworks for real-time stream processing and machine learning, providing the capability to analyze log streams and detect anomalies in real-time.
By integrating machine learning algorithms into log analysis pipelines, Java developers can build intelligent systems that autonomously detect and respond to aberrations within log data, enhancing the overall robustness and reliability of their applications and infrastructure.
Bringing It All Together
In the age of big data and distributed systems, efficient log analysis is not just a necessity but a strategic advantage. With a myriad of tools, libraries, and frameworks at their disposal, Java developers can build scalable and intelligent log analysis pipelines that uncover valuable insights from the vast sea of log data. By leveraging the power of Java and its ecosystem, developers can conquer the challenges posed by data overload and extract actionable intelligence from their log data, paving the way for more reliable and performant systems.
Log analysis in Java is not merely a technical endeavor, it is a means to unlock the true potential of data-driven decision making in the realm of software development and operations. With the right tools and techniques, Java developers can harness the power of log data to drive continuous improvement and innovation within their organizations.
Stay tuned for more insightful articles on Java development and stay ahead in the ever-evolving world of technology.