Unraveling ThreadLocal: Common Java Concurrency Pitfalls
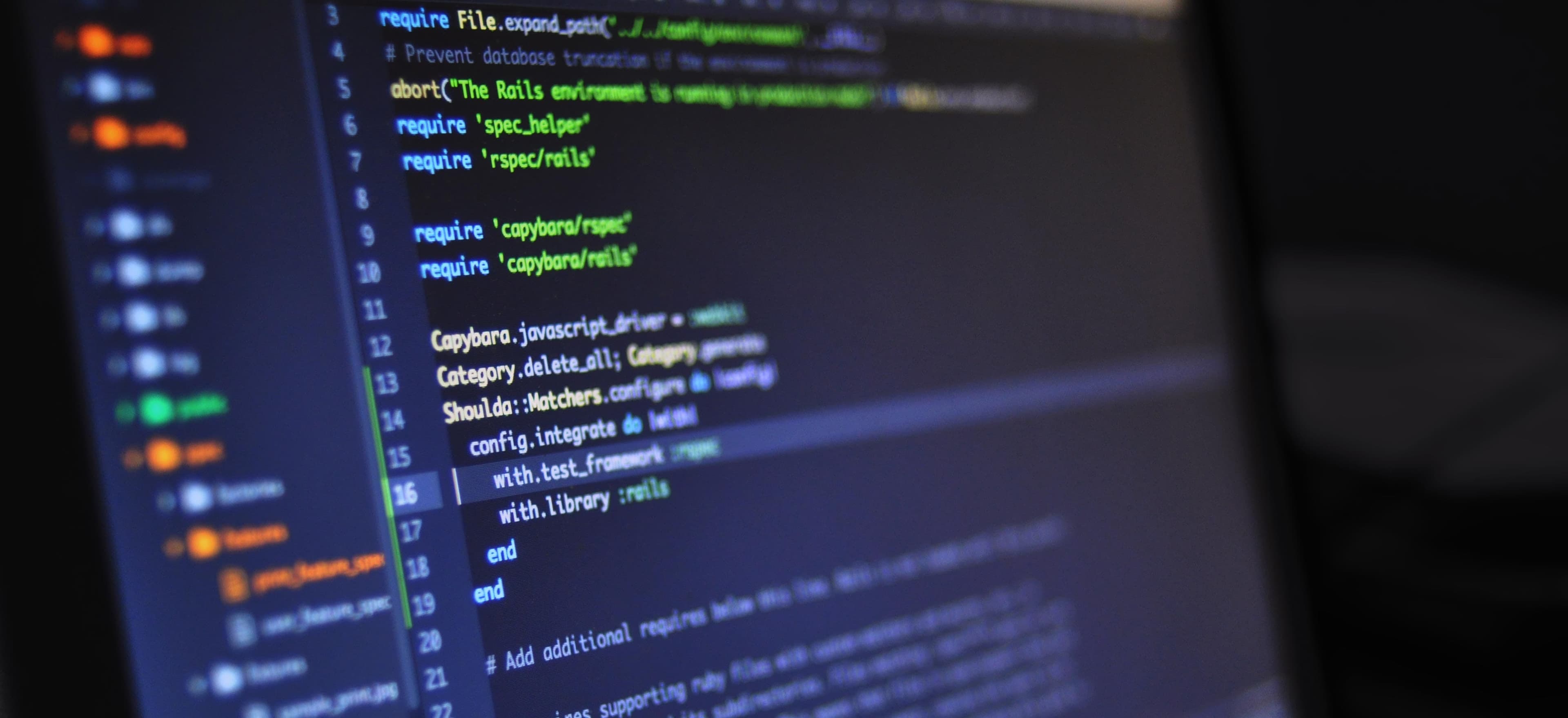
- Published on
Unraveling ThreadLocal: Common Java Concurrency Pitfalls
In the landscape of multithreading in Java, ThreadLocal
often surfaces as a powerful tool. It allows you to maintain per-thread contexts, simplifying the management of data that is specific to a thread. However, while it can alleviate some common concurrency challenges, its misuse can lead to unexpected pitfalls. This article explores ThreadLocal
, its proper usage, and the common mistakes developers often make.
Understanding ThreadLocal
ThreadLocal
is a class in Java that provides thread-local variables. Each thread accessing such a variable has its own, independently initialized copy of the variable. This isolation reduces contention in concurrent environments because threads can read and write these local copies without interference.
Basic Example
Here's a simple example demonstrating how ThreadLocal
works:
public class ThreadLocalExample {
private static ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 0);
public static void main(String[] args) throws InterruptedException {
Runnable task = () -> {
Integer initialValue = threadLocalValue.get();
System.out.println(Thread.currentThread().getName() + ": Initial Value: " + initialValue);
threadLocalValue.set(initialValue + 1);
System.out.println(Thread.currentThread().getName() + ": Updated Value: " + threadLocalValue.get());
};
Thread thread1 = new Thread(task);
Thread thread2 = new Thread(task);
thread1.start();
thread2.start();
thread1.join();
thread2.join();
}
}
Explanation
- Initialization: The
ThreadLocal
variablethreadLocalValue
is initialized using a lambda expression. Each thread accessing this variable will start with an initial value of 0. - Thread Execution: When each thread runs the
task
, it retrieves its local value, increments it, and prints the updated value.
This encapsulation makes ThreadLocal
a convenient choice for managing user sessions, database connections, or other data requiring thread-specific integrity.
Common Pitfalls with ThreadLocal
While ThreadLocal
has clear advantages, improper usage can lead to issues, such as memory leaks or concurrency problems. Let’s dive into these common pitfalls.
1. Memory Leaks
One of the most critical issues arises when ThreadLocal
variables are not cleared after use. If threads remain in existence, such as in thread pools, the ThreadLocal
object holds a reference to its value, preventing garbage collection. This can lead to memory leaks.
Solution:
Always remove the ThreadLocal variable after use.
public static void cleanUp() {
threadLocalValue.remove(); // Clear thread-local value
}
2. Unintentional Sharing
If a ThreadLocal
variable is not declared explicitly as static, it may lead to unintended sharing across threads. This can create scenarios where one thread alters the value, affecting others.
Recommended Practice:
Keep the ThreadLocal
variable static unless there's a compelling reason not to.
private static ThreadLocal<Integer> threadLocalValue = ThreadLocal.withInitial(() -> 0);
3. Overusing ThreadLocal
While ThreadLocal
can simplify code, overusing it can make your codebase hard to maintain and understand. Sometimes, simpler constructs like passing objects between threads or using concurrent collections can achieve similar outcomes without the added complexity of ThreadLocal
.
Tips for Proper Use:
- Assess the necessity of
ThreadLocal
for each use case. - Use it when you have truly per-thread data that's expensive to compute.
- Consider alternatives like
ThreadPoolExecutor
for shared resources.
4. Complex Data Structures
Using ThreadLocal
with complex data structures can lead to issues if not carefully managed. For instance, if you store a collection or map in a ThreadLocal
, not taking care of its lifecycle can lead to unintended behavior when multiple threads access or modify it.
Example Caution:
private static ThreadLocal<Map<String, String>> threadLocalMap = ThreadLocal.withInitial(HashMap::new);
// Use with caution
public static void updateMap(String key, String value) {
threadLocalMap.get().put(key, value); // Ensure to manage the Map's lifecycle to avoid unintended sharing
}
5. Error-Prone Debugging
Debugging issues related to ThreadLocal
can be tricky. Since the state is maintained per thread, determining the cause of bugs can be challenging when the threads diverge in execution.
Debugging Strategies:
- Utilize logging effectively.
- Print thread details along with the thread-local values to monitor changes.
Optimal Use Cases for ThreadLocal
Despite its pitfalls, ThreadLocal
is invaluable when used correctly. Here are some scenarios where its application is beneficial:
- User Sessions: Store user-specific data (such as user authentication details) that should not leak between threads.
- Database Connections: Maintain unique connections for each thread, avoiding the overhead of establishing a connection for each operation.
- Transaction Context: Keep track of transaction states in applications managing concurrent requests.
More Resources
For deeper understanding and advanced concepts of concurrency in Java, consider checking out the following resources:
- Java Concurrency in Practice – An essential book for mastering concurrency in Java.
- Java Documentation on ThreadLocal – Official documentation providing in-depth insights into the class and its methods.
My Closing Thoughts on the Matter
ThreadLocal
is a powerful construct for managing thread-specific data in Java. By keeping the potential pitfalls in mind and adhering to best practices, developers can leverage its benefits while minimizing risks. As with any tool, the key lies in understanding when and how to use it effectively, ensuring that it enhances application performance without introducing unnecessary complexity.
Incorporate these insights into your next Java project to master concurrency and refine your coding approach!