Handling Edge Cases with BigDecimal in Java
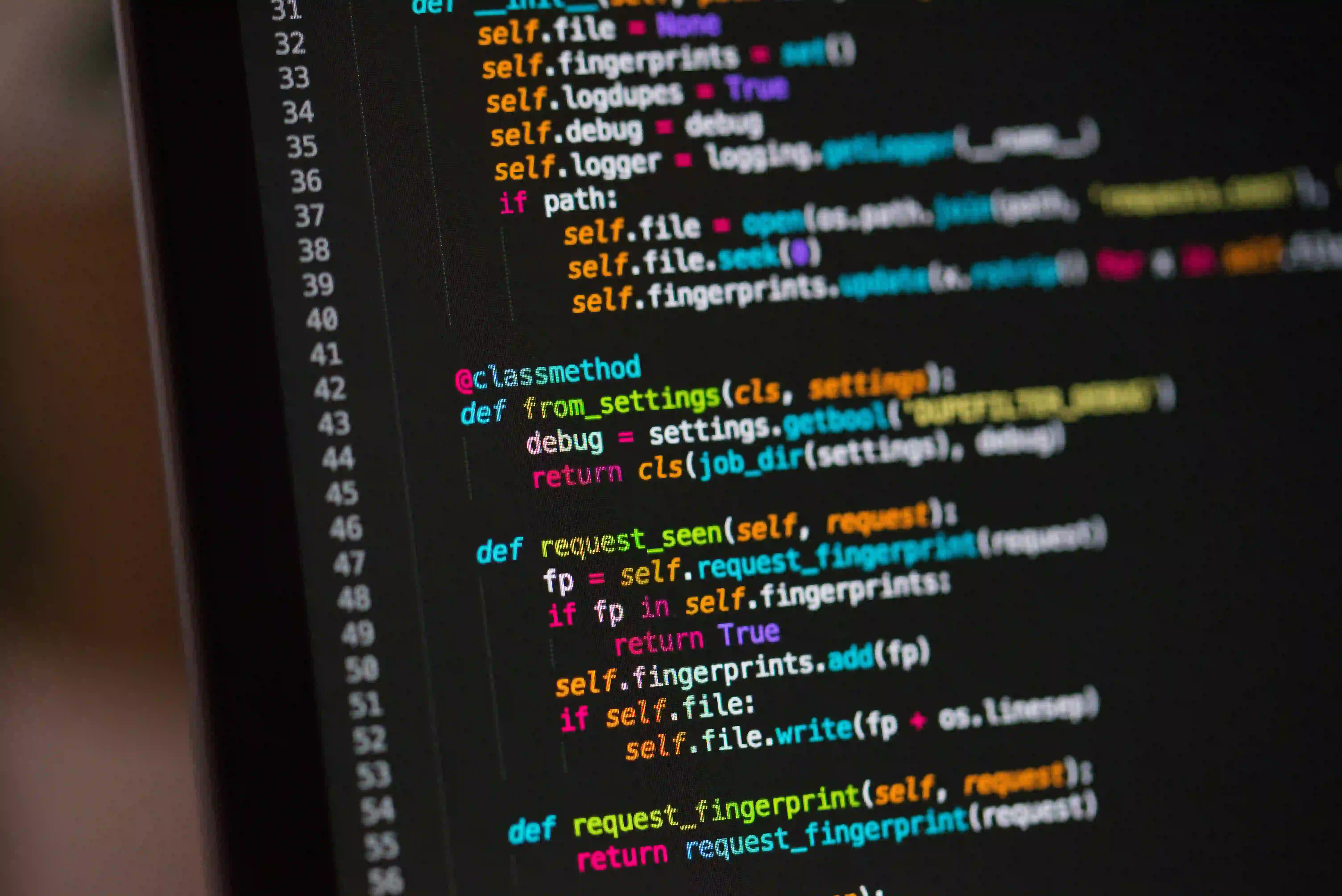
Handling Edge Cases with BigDecimal in Java
Java is known for its robust data types, especially when it comes to numerical representation. However, not all numerical types are created equal. When precision matters, the BigDecimal
class comes into play. This class is designed to handle monetary calculations and other situations where precision is critical. In this article, we will delve into how to handle edge cases with BigDecimal
, ensuring that your financial applications or any numerically sensitive Java programs maintain accuracy.
Understanding BigDecimal
BigDecimal
is part of the java.math
package, and it provides operations for arithmetic, scale manipulation, rounding, comparison, hashing, and format conversions.
import java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal number1 = new BigDecimal("123.45");
BigDecimal number2 = new BigDecimal("67.89");
BigDecimal sum = number1.add(number2);
System.out.println("Sum: " + sum); // Output: Sum: 191.34
}
}
In the example above, notice how the BigDecimal
constructor takes a String
argument. This is crucial because constructing a BigDecimal
from a double
can lead to precision issues. For instance:
BigDecimal bd1 = new BigDecimal(0.1);
BigDecimal bd2 = new BigDecimal(0.2);
BigDecimal result = bd1.add(bd2);
System.out.println("Result: " + result); // Might output 0.30000000000000004
Why Use BigDecimal?
- Precision: Unlike floating-point types (
float
ordouble
),BigDecimal
provides an immutable, arbitrary-precision signed decimal number. - Control: It allows for precise control over scale and rounding.
- Financial Applications: In finance, where transactions depend upon cents,
BigDecimal
handles currency with utmost accuracy.
Common Edge Cases in BigDecimal
1. Rounding Modes
When performing arithmetic operations, rounding becomes an essential topic. Inconsistencies can arise when not specifying how to round off numbers:
BigDecimal value = new BigDecimal("2.675");
BigDecimal roundedValue = value.setScale(2, BigDecimal.ROUND_HALF_UP);
System.out.println("Rounded Value: " + roundedValue); // Might output 2.68
Why Rounding Matters: Different industries may follow various rounding rules, making it vital to choose the correct RoundingMode
based on the application context.
2. Scale issues
Scale defines the number of digits to the right of the decimal point. Weighty inconsistencies can arise if you do not explicitly set it:
BigDecimal bd = new BigDecimal("1.23456789");
System.out.println("Scale: " + bd.scale()); // Output: 8
BigDecimal bdScaled = bd.setScale(2, RoundingMode.HALF_UP);
System.out.println("Scaled: " + bdScaled); // Output: 1.23
3. Comparisons with Zero
Deciding how to handle comparisons can have larger implications. Comparing BigDecimal
objects with zero can yield surprising results:
BigDecimal zero = BigDecimal.ZERO;
BigDecimal negative = new BigDecimal("-0.000000000001");
System.out.println(zero.compareTo(negative)); // Output: 1 (means zero is greater)
Why this Matters: Such comparisons may determine whether a function proceeds or not. Understanding the return result of comparison operations (-1
, 0
, 1
) helps avoid logic errors.
4. Avoiding Conversion Issues
One common error is transitioning between types. A direct conversion from double
to BigDecimal
is not advisable. Instead, always use String
:
BigDecimal bdFromDouble = new BigDecimal(doubleValue); // Don't use this!
BigDecimal bdFromString = new BigDecimal(Double.toString(doubleValue)); // Prefer this!
5. Immutability and Modifications
BigDecimal
is immutable. Any modification returns a new instance, avoiding any confusion caused by mutable data types:
BigDecimal original = new BigDecimal("10.123");
BigDecimal updated = original.add(new BigDecimal("2.3"));
System.out.println("Original: " + original); // Output: 10.123
System.out.println("Updated: " + updated); // Output: 12.423
Why Immutability Matters: Programming with immutables helps to eliminate concurrency issues, making your code safer in multi-threaded environments.
6. Handling Special Values
Ensure that your code gracefully handles special values like NaN
(Not-a-Number) and Infinity
. These cases often occur during complex calculations:
BigDecimal nanValue = new BigDecimal("NaN");
System.out.println(nanValue.isNaN()); // Output: true
7. Performance Considerations
While BigDecimal
offers precision, it comes with performance trade-offs compared to primitive types. For heavy computational tasks with immense data, consider profiling your application to see if the trade-off makes sense.
Best Practices
- Always use
String
to constructBigDecimal
instances. - Establish detailed rounding rules defined by the application requirements.
- Normalize small decimal values to avoid precision issues over several operations.
- Use constants like
MathContext
for standardized configurations across your application.
Example: Implementing a Simple Financial Calculator
Here is a practical implementation of a financial application that uses BigDecimal
for precise monetary calculations:
import java.math.BigDecimal;
import java.math.RoundingMode;
public class FinancialCalculator {
public static BigDecimal calculateTotal(int quantity, BigDecimal pricePerUnit) {
BigDecimal quantityBD = new BigDecimal(quantity);
return quantityBD.multiply(pricePerUnit).setScale(2, RoundingMode.HALF_UP);
}
public static void main(String[] args) {
BigDecimal pricePerUnit = new BigDecimal("19.99");
int quantity = 5;
BigDecimal total = calculateTotal(quantity, pricePerUnit);
System.out.println("Total: " + total); // Output: Total: 99.95
}
}
Final Considerations
Working with BigDecimal
allows developers to handle precision in Java universally. It's crucial to understand its intricacies, including edge cases associated with conversion, scaling, and rounding. Use best practices, keep an eye on performance, and always validate your outputs.
For more information on BigDecimal
and its functionality, refer to the Java Documentation.
With this knowledge, you’re better equipped to handle numerical precision in Java, especially when building applications requiring accurate financial calculations or scientific computations.