The Pitfalls of Java's Finalize Method: Safer Alternatives
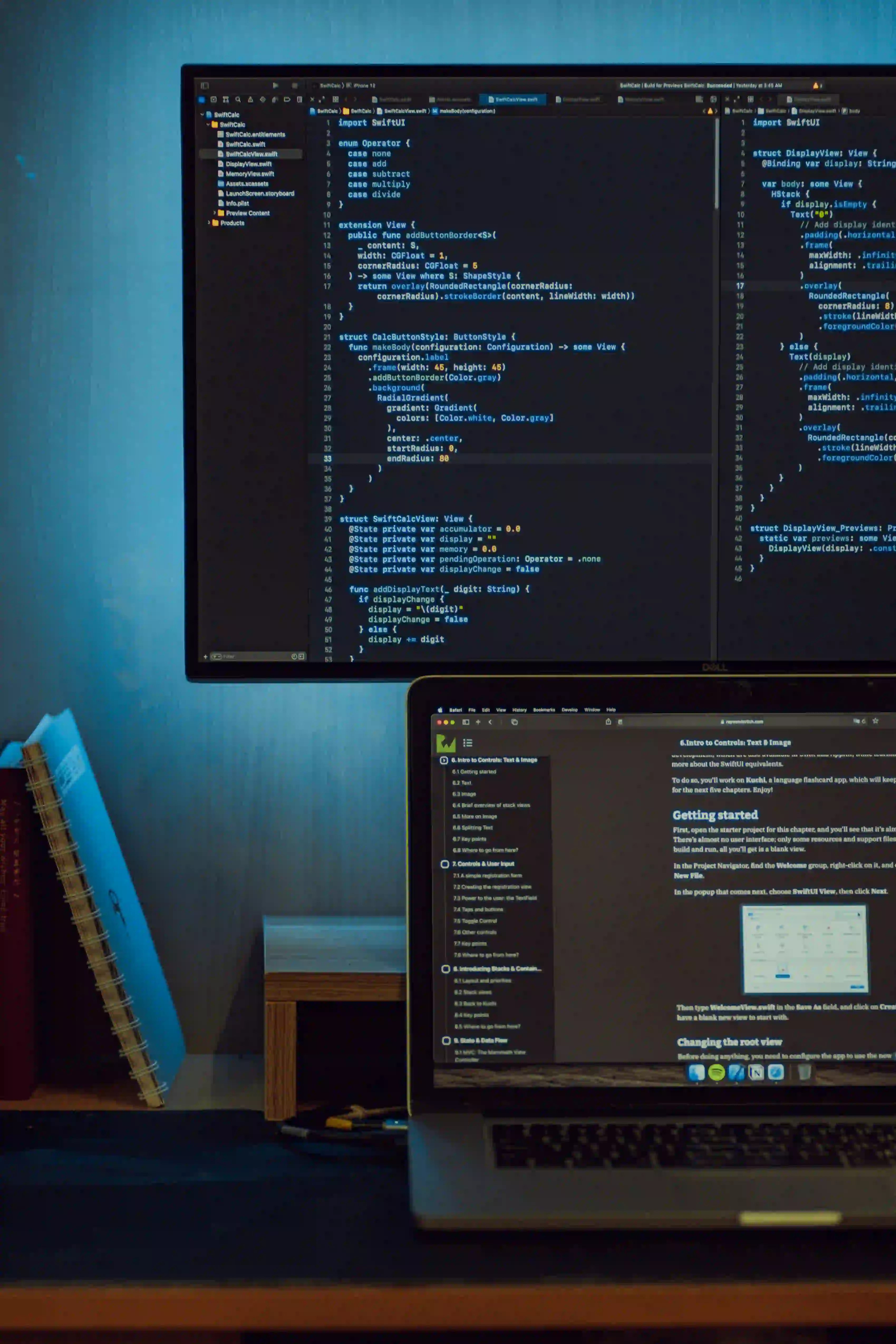
The Pitfalls of Java's Finalize Method: Safer Alternatives
Java is renowned for its robust memory management and garbage collection, yet it has provided developers with a mechanism that, at times, can lead to more confusion than clarity: the finalize()
method. As Java has evolved, best practices and recommendations have shifted, necessitating a deeper understanding of finalize()
—its pitfalls and safer alternatives.
In this blog post, we will explore the intricacies of the finalize()
method, its downsides, and what you can do instead to manage resource cleanup effectively.
Understanding the Finalize Method
In Java, the finalize()
method is defined in the java.lang.Object
class. It is invoked by the garbage collector when an object is about to be reclaimed. This offers developers a chance to perform any necessary cleanup actions before the object is destroyed. The method signature looks like this:
protected void finalize() throws Throwable {
// Cleanup code
super.finalize();
}
The Role of Finalize
Here is why the finalize()
method was originally alluring:
- Automatic Resource Management: It allowed for automatic callback mechanisms for releasing resources.
- Encapsulation of Cleanup Logic: The cleanup logic could reside within the object itself, offering encapsulation and coherence.
While it seems beneficial, the reality is less rosy.
The Pitfalls of Using Finalize
1. Unpredictable Timing
The execution timing of the finalize()
method is uncertain. The garbage collector does not guarantee when, or even if, finalize()
will be executed.
public class Resource {
@Override
protected void finalize() throws Throwable {
try {
// Clean up resources
} finally {
super.finalize();
}
}
}
In this example, you may expect the cleanup to occur immediately after the object becomes unreachable. However, this does not happen in a predictable manner, leading to potential resource leaks.
2. Performance Overhead
Invoking finalize()
adds overhead to garbage collection. The garbage collector has to maintain a list of objects with finalizers, which can hinder performance.
3. Dependence on Finalization
Relying on finalize()
for essential resource management is dangerous. If an object is never garbage collected, such as in the presence of circular references, the finalization will never occur.
4. Subtle Bugs
Using finalize()
can easily introduce hard-to-track bugs, especially if it references other objects. If those objects are also finalized, it can lead to a chain of calls that results in exceptions or inconsistent states.
public class FinalizerExample {
@Override
protected void finalize() throws Throwable {
try {
// Potentially using other objects here
} finally {
super.finalize();
}
}
}
This might lead to confusing scenarios where object states become unpredictable.
Safer Alternatives to Finalize
Given the downsides of finalize()
, Java provides several safer alternatives to manage resources.
1. Try-With-Resources Statement
The try-with-resources statement simplifies resource management for classes that implement the AutoCloseable
interface. This approach ensures that resources are closed promptly and properly.
public class Resource implements AutoCloseable {
public void useResource() {
// Resource usage logic here
}
@Override
public void close() {
// Resource cleanup logic here
}
}
// Usage
try (Resource resource = new Resource()) {
resource.useResource();
} catch (Exception e) {
// Handle exceptions
}
In this scenario, once the try
block is exited, either through successful execution or an exception, Java’s garbage collector will automatically call the close()
method for any resources opened.
2. Explicit Cleanup Methods
If the automatic nature of try-with-resources
does not suffice, consider implementing an explicit cleanup method.
public class ExplicitCleanup {
private boolean resourceInUse;
public void useResource() {
resourceInUse = true;
// Resource usage logic here
}
public void cleanup() {
if (resourceInUse) {
// Cleanup logic
resourceInUse = false;
}
}
}
// Usage
ExplicitCleanup ec = new ExplicitCleanup();
try {
ec.useResource();
// Other logic
} finally {
ec.cleanup(); // Ensures cleanup is called explicitly
}
3. Using Weak References
Another alternative is to use WeakReference instances, which allow you to reference an object while still allowing it to be garbage collected.
import java.lang.ref.WeakReference;
public class WeakReferenceExample {
private WeakReference<Resource> resourceRef;
public void initializeResource() {
Resource resource = new Resource();
resourceRef = new WeakReference<>(resource);
}
public void useResource() {
Resource resource = resourceRef.get();
if (resource != null) {
// Use the resource
} else {
// Resource has been garbage collected
}
}
}
This pattern is particularly useful when managing caches or holding references without preventing garbage collection.
4. Frameworks and Libraries
Lastly, consider leveraging existing libraries or frameworks, such as Apache Commons IO or Spring, which provide utilities for resource management.
These encapsulated libraries reinforce best practices and can simplify your code by replacing manual cleanup logic.
Final Thoughts
In summary, while the finalize()
method offers a mechanism for cleanup, its unpredictability, performance overhead, and potential to introduce subtle bugs make it a risky choice.
Instead, adoption of safer alternatives like try-with-resources, explicit cleanup methods, weak references, and already established libraries will provide you with more control over resource management in your Java applications.
If you’re looking to implement best practices for resource handling in Java effectively, steering clear of finalize()
is wise. Choose any of the alternatives above and streamline your code while maintaining clarity and efficiency.
Additional Resources
By understanding these corners of Java's memory management, you lay the groundwork for writing safer, more robust applications while minimizing overhead. Happy coding!