Navigating Java's Garbage Collector Options: A Clear Guide
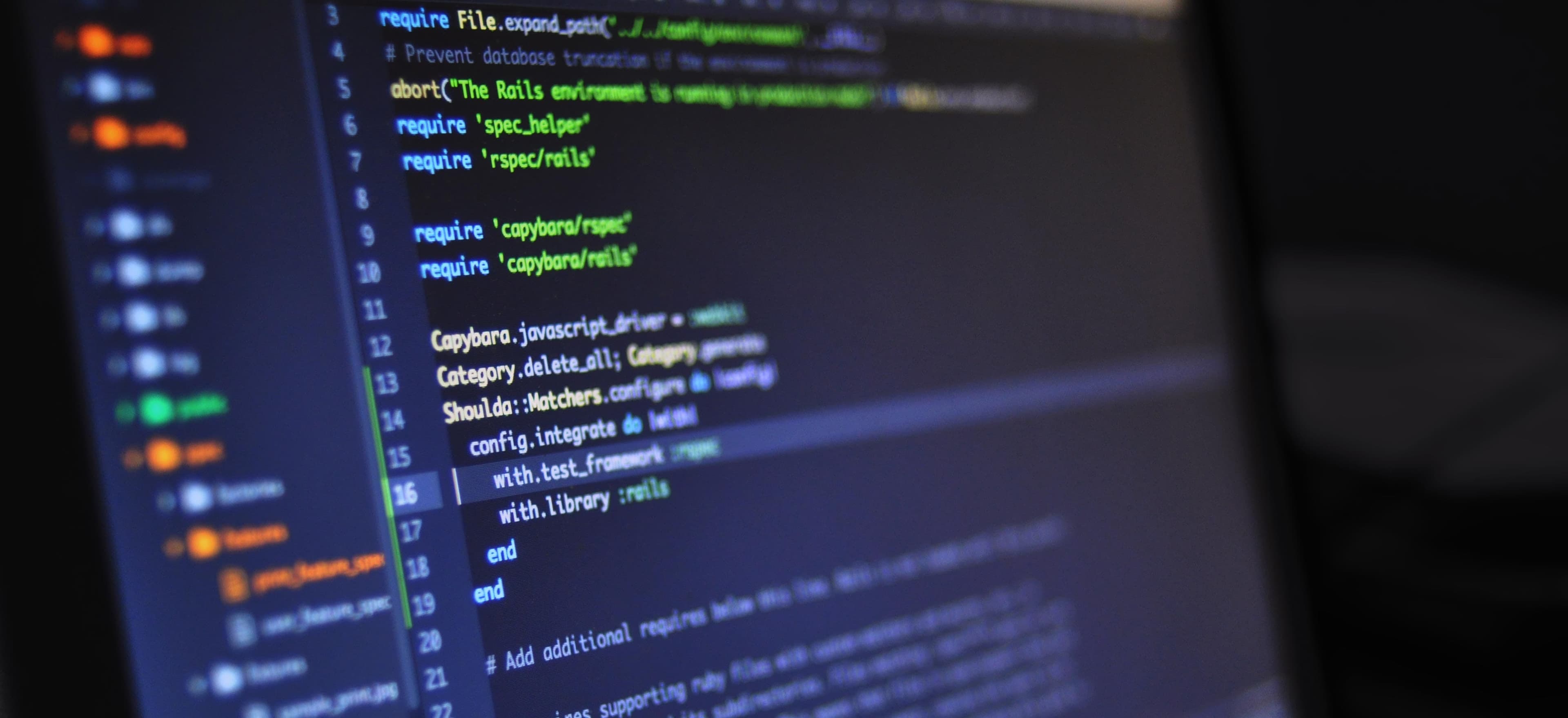
- Published on
Navigating Java's Garbage Collector Options: A Clear Guide
Java is a widely-used programming language that offers robust features and a dynamic ecosystem. One of its core strengths is its automatic memory management, powered by its Garbage Collector (GC). The Garbage Collector is a crucial component that helps maintain optimal memory usage in Java applications. Understanding the various garbage collector options available can empower developers to make informed decisions that can enhance application performance.
What is Garbage Collection?
Garbage Collection in Java refers to the process of identifying and disposing of objects that are no longer needed by a program. It frees up memory space, helping to optimize the application's performance. Unlike languages like C or C++, where manual memory management is mandatory, Java’s built-in garbage collection simplifies memory management.
How Does Garbage Collection Work?
The Java Virtual Machine (JVM) reallocates memory by using various garbage collection algorithms. It typically revolves around two primary actions: Mark and Sweep. Here’s a simplified view of the process:
- Marking Phase: The JVM scans the heap and marks all reachable objects.
- Sweeping Phase: The JVM goes through the heap and cleans up all unmarked objects, thus releasing memory.
With each GC type offering unique performance characteristics, Java developers should be aware of the available options.
Types of Garbage Collectors in Java
Java provides several garbage collectors, each with its strengths and use cases. Let’s deep dive into the primary garbage collector implementations.
1. Serial Garbage Collector
The Serial Garbage Collector is a simple and straightforward GC algorithm designed for single-threaded environments. It uses a single thread to perform memory management.
Code Example:
public class Example {
public static void main(String[] args) {
// Enable Serial Garbage Collector
System.setProperty("java.opts", "-XX:+UseSerialGC");
// Simulate a task
for (int i = 0; i < 100000; i++) {
String str = new String("String " + i);
}
}
}
Why Use Serial GC?
The Serial GC is best suited for applications with small data sets and less overall memory usage. It is also the default collector for clients running on Java.
2. Parallel Garbage Collector
The Parallel Garbage Collector utilizes multiple threads for both marking and sweeping phases. This concurrency can significantly improve throughput in multi-core processors.
Code Example:
public class ParallelGCExample {
public static void main(String[] args) {
// Enable Parallel Garbage Collector
System.setProperty("java.opts", "-XX:+UseParallelGC");
// Simulate a task
for (int i = 0; i < 200000; i++) {
Integer[] integers = new Integer[1000];
}
}
}
Why Use Parallel GC?
Parallel GC is ideal for applications requiring lower latency and high throughput. It is often employed for server-side applications.
3. Concurrent Mark-Sweep (CMS) Garbage Collector
The CMS Garbage Collector is designed to minimize pauses. It tries to perform most of its work concurrently with the application threads.
Code Example:
public class CMSExample {
public static void main(String[] args) {
// Enable CMS Garbage Collector
System.setProperty("java.opts", "-XX:+UseConcMarkSweepGC");
// Simulate a task
for (int i = 0; i < 300000; i++) {
String[] strings = new String[500];
}
}
}
Why Use CMS?
CMS is particularly well-suited for applications requiring low latency, such as real-time systems. However, it can be less efficient in handling fragmentation over time.
4. G1 Garbage Collector
The Garbage First (G1) Collector is designed for applications with large heaps. It splits the heap into regions, allowing for more incremental garbage collection.
Code Example:
public class G1Example {
public static void main(String[] args) {
// Enable G1 Garbage Collector
System.setProperty("java.opts", "-XX:+UseG1GC");
// Simulate a task
for (int i = 0; i < 1000000; i++) {
double[] doubles = new double[10000];
}
}
}
Why Use G1?
G1 is beneficial for applications with large heaps, aiming for predictable pause times. It is the default GC in Java 9 and later versions.
Choosing the Right Garbage Collector
Choosing the right garbage collector requires a deep understanding of your application's requirements:
- Application Type: Determine if your application is batch-oriented, transactional, or requires low latency.
- Hardware Environment: Factor in the number of processor cores and the nature of the workload.
- Memory Usage: Assess overall memory footprint and decide based on allocation patterns.
Performance Monitoring
To evaluate GC performance, you can enable GC logging using the following JVM options:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:<file-path>
This logging can help you analyze the frequency and duration of garbage collection events, assisting in further optimization.
A Final Look
Understanding the various garbage collector options in Java can have a significant impact on application performance, resource allocation, and memory management. By assessing your project's unique needs, you can select the most suitable garbage collection strategy.
Further Reading
For an in-depth understanding, consider exploring more resources:
- Java Garbage Collection Basics
- Understanding Java Garbage Collection
- Java Performance Tuning
Navigating garbage collection can seem daunting at first, but with the right knowledge and tools, it can be effectively managed to improve performance and reliability in your Java applications. If you are looking to delve deeper into this topic, consider experimenting with different collectors on your own codebase to see real-world impacts!
Checkout our other articles