Overcoming Limitations of Java Proxy Classes in Your Projects
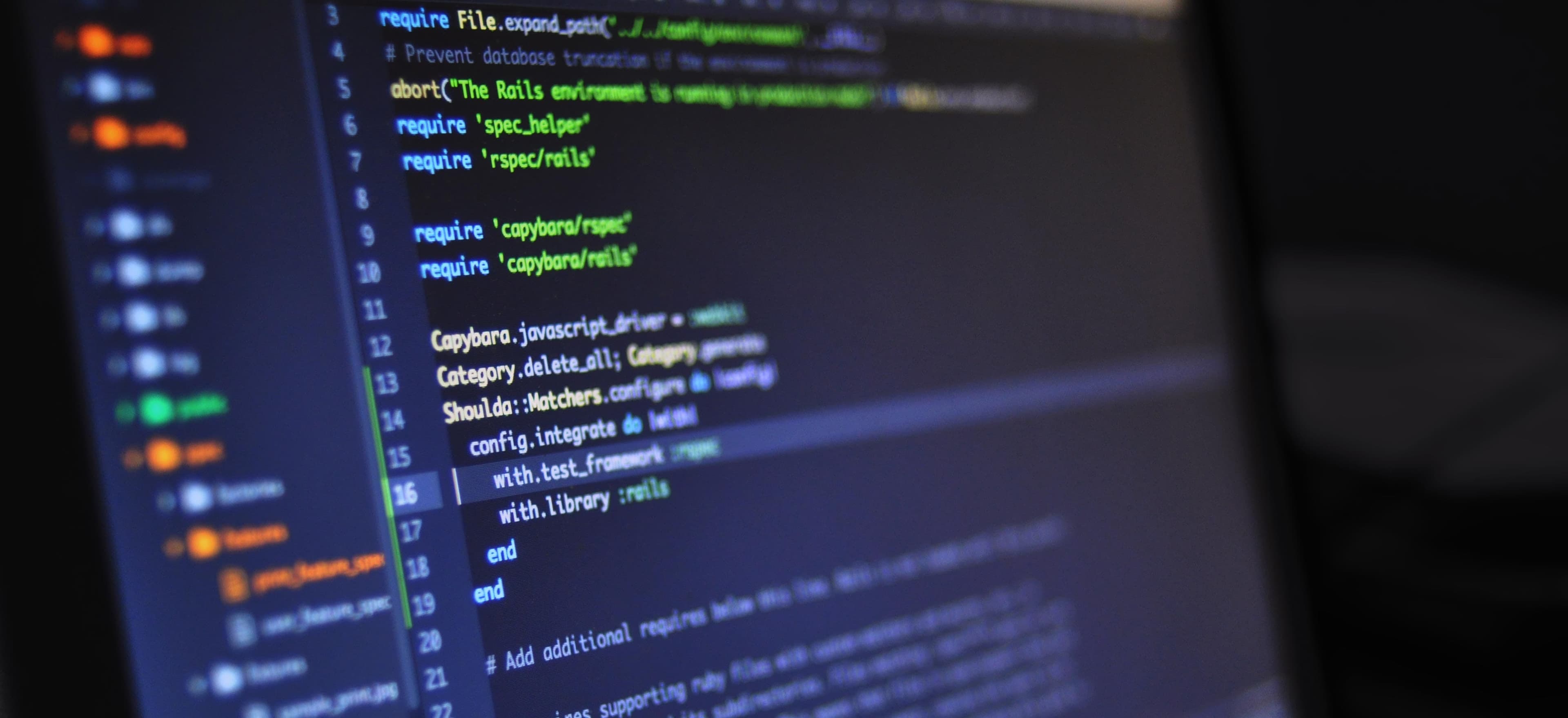
- Published on
Overcoming Limitations of Java Proxy Classes in Your Projects
Java proxy classes offer a robust method for implementing design patterns like the Proxy Pattern, which can be extremely useful in scenarios like algorithm delegation, security, and performance. However, navigating the limitations of these proxy classes requires an understanding of the underlying mechanisms and careful design considerations. This blog post will delve into the challenges posed by Java proxy classes and how to mitigate them effectively.
Setting the Stage to Java Proxy Classes
In Java, proxy classes are often utilized to create dynamic proxies that can implement one or more interfaces at runtime. The java.lang.reflect.Proxy
class is a core component that enables developers to dynamically create proxy objects. For a deeper understanding of proxy classes, refer to Oracle's documentation on Reflection.
Here's a basic example of how to create a proxy in Java:
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
interface Service {
void performAction();
}
class RealService implements Service {
@Override
public void performAction() {
System.out.println("Performing action in RealService");
}
}
class ServiceProxy implements InvocationHandler {
private Object realService;
public ServiceProxy(Object realService) {
this.realService = realService;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before action");
Object result = method.invoke(realService, args);
System.out.println("After action");
return result;
}
}
public class ProxyDemo {
public static void main(String[] args) {
RealService realService = new RealService();
ServiceProxy proxy = new ServiceProxy(realService);
Service service = (Service) Proxy.newProxyInstance(
realService.getClass().getClassLoader(),
realService.getClass().getInterfaces(),
proxy
);
service.performAction();
}
}
This code snippet demonstrates a simple proxy mechanism. The InvocationHandler
intercepts calls to the performAction
method, allowing you to add additional behavior like logging.
Limitations of Java Proxy Classes
While proxy classes can streamline many processes, they come with inherent limitations:
-
Interface Restriction: Java's dynamic proxies can only implement interfaces. If your target class has no interfaces, you're out of luck.
-
Performance Overhead: The reflective nature of proxies adds a level of overhead that can affect performance, especially if mismanaged.
-
Complexity in Error Handling: Errors in the dynamic proxy invocation can lead to complex debugging scenarios, as they often require tracing through multiple method calls.
-
Difficulty in State Management: Proxies generally don't maintain state unless specifically coded to do so, which can make managing shared data between real and proxy classes challenging.
-
Limited Support for Final Classes: Proxy classes cannot extend final classes or override their methods, which can be a significant limitation when dealing with legacy systems.
Overcoming Interface Restriction
One common workaround is to use the Adapter Pattern. This pattern allows a class to implement multiple interfaces, thus enabling proxy capabilities even if the original class does not expose the necessary interfaces.
Example of Using the Adapter Pattern
Consider the following example:
class LegacyService {
public void oldMethod() {
System.out.println("Old method in LegacyService");
}
}
interface NewService {
void performAction();
}
class Adapter implements NewService {
private LegacyService legacyService;
public Adapter(LegacyService legacyService) {
this.legacyService = legacyService;
}
@Override
public void performAction() {
legacyService.oldMethod();
}
}
In this scenario, the Adapter
class allows the use of LegacyService
in a new context, effectively enabling proxy-like behavior.
Minimizing Performance Overhead
When it comes to managing performance, here are some strategies:
-
Limit Proxy Invocation: Use phases or flags to limit when proxy methods are invoked.
-
Caching: If a proxy returns the same result multiple times, cache the results to avoid unnecessary overhead.
-
Compile-time Proxies: In certain cases, consider using libraries like ByteBuddy or Javassist to generate cached or compile-time proxies rather than relying on reflection.
Error Handling Best Practices
Given that proxies can complicate error handling, it’s essential to implement robust logging and validation techniques:
Example of Error Handling in Proxies
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
try {
System.out.println("Before action");
Object result = method.invoke(realService, args);
return result;
} catch (Throwable throwable) {
System.err.println("Error occurred: " + throwable.getMessage());
throw throwable; // Let it bubble up
} finally {
System.out.println("After action");
}
}
Tips for Effective Logging
- Use a logging framework like SLF4J or Log4j to capture proxy interaction reliably.
- Record invocation parameters and results to enable better debugging insights.
Managing State Effectively
Java proxies do not inherently manage state. Implementing your state management mechanism requires careful design:
-
Decorator Pattern: Utilize the Decorator Pattern to maintain state in the proxy while adding new responsibilities.
-
State Holder Classes: Create separate classes or interfaces responsible for maintaining state across proxies.
Example of Using a State Holder
class StateHolder {
private int state;
public int getState() {
return state;
}
public void setState(int state) {
this.state = state;
}
}
class StateServiceProxy implements InvocationHandler {
private final Object realService;
private final StateHolder state;
public StateServiceProxy(Object realService, StateHolder state) {
this.realService = realService;
this.state = state;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
// Access state before invoking the actual service method
System.out.println("Current state: " + state.getState());
return method.invoke(realService, args);
}
}
Handling Final Classes
If you need to work with final classes, there are a few options:
-
Wrapper Classes: Introduce your own classes that encapsulate the final classes, allowing you to proxy around their methods.
-
Interface-Based Design: Revisit your design to allow for interface-based definitions, which can help circumvent this limitation altogether.
Key Takeaways
Java proxy classes provide essential capabilities for adding abstraction layers and enhancing application design. However, to capitalize on their potential, developers must understand their limitations and adopt strategic design patterns and solutions.
By utilizing interfaces effectively, mitigating performance issues, ensuring error resilience, managing state efficiently, and navigating restrictions with creative design strategies, you can overcome these obstacles and implement proxy classes successfully in your Java projects.
For further reading and resources, consider exploring:
By leveraging these insights and techniques, your project will be better equipped to handle the powerful yet complex world of Java proxy classes. Happy coding!
Checkout our other articles