Uncovering Java's Tricky Floating Point Pitfalls
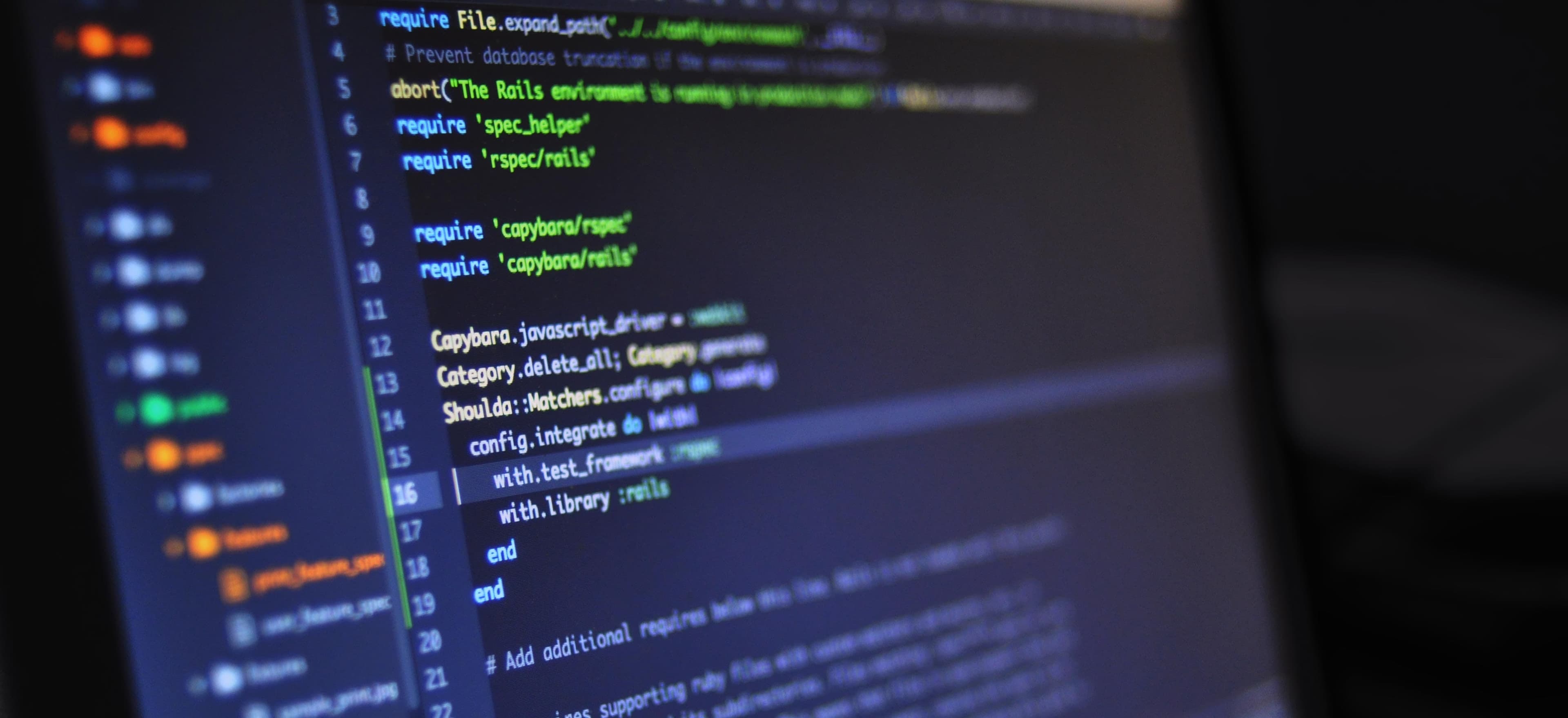
- Published on
Uncovering Java's Tricky Floating Point Pitfalls
Floating point numbers in Java can seem straightforward at first glance, but they come with their own set of challenges. Understanding these pitfalls is crucial for developers to avoid unexpected behavior in their applications. In this blog post, we will delve into the nuances of floating point arithmetic, explore common pitfalls, suggest best practices, and provide some code examples to illustrate these concepts.
Understanding Floating Point Representation
Java uses the IEEE 754 standard for representing floating point numbers, which means that it can accurately represent a finite number of decimal fractions. However, not all decimal numbers can be represented exactly in binary form. This can lead to errors in calculations, especially when dealing with monetary values and precise measurements.
For example, the decimal number 0.1 cannot be precisely represented as a binary floating point number. As a result, calculations that involve floating point numbers can yield unexpected results.
Example: Precision Issues
Consider the following Java code snippet:
public class Main {
public static void main(String[] args) {
double a = 0.1;
double b = 0.2;
double sum = a + b;
System.out.println("Sum is: " + sum);
System.out.println("Is the sum equal to 0.3? " + (sum == 0.3));
}
}
Output:
Sum is: 0.30000000000000004
Is the sum equal to 0.3? false
Explanation
In the above example, although mathematically, 0.1 + 0.2 should equal 0.3, the actual result is slightly off due to how these numbers are represented in memory. This discrepancy illustrates why you should never rely on floating point numbers for equality checks.
Common Pitfalls with Floating Point Arithmetic
1. Precision Loss
As demonstrated, operations involving floating point numbers can result in precision loss. This is particularly problematic in financial calculations where accuracy is paramount.
2. Comparison Issues
Using the ==
operator to compare floating point numbers can lead to surprising results. Given the imprecision of floating point arithmetic, it's advisable to use a method that accounts for a small margin of error (epsilon).
Example: Safe Comparison Method
public class FloatingPointComparison {
public static boolean almostEqual(double a, double b, double epsilon) {
return Math.abs(a - b) < epsilon;
}
public static void main(String[] args) {
double a = 0.1 + 0.2;
double b = 0.3;
double epsilon = 0.00001;
System.out.println("Are they almost equal? " + almostEqual(a, b, epsilon));
}
}
Explanation
In this snippet, we define a function almostEqual
which checks if two floating-point numbers are close enough to be considered equal by allowing a small margin (epsilon). This is a better approach than using direct comparison.
3. Accumulative Errors
Repeatedly performing calculations can lead to error accumulation. For example, summing a sequence of floating point numbers can yield results that diverge significantly from expected values, especially for large datasets.
Example: Accumulatively Adding a Series of Floating Points
public class AccumulationExample {
public static void main(String[] args) {
double sum = 0.0;
for (int i = 0; i < 1000000; i++) {
sum += 0.1;
}
System.out.println("Accumulated sum: " + sum);
}
}
Output:
Accumulated sum: 100000.0
Explanation
While it appears that adding 0.1 a million times should result in 100000.0, floating point precision issues might subtly affect this result if the operation is done in certain ways or contexts.
Best Practices to Avoid Floating Point Pitfalls
1. Use BigDecimal for Precise Calculations
For financial applications or any scenario requiring high precision, consider using BigDecimal
. It provides exact decimal representation, avoiding the pitfalls of floating point arithmetic.
Example: Using BigDecimal for Accurate Representation
import java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal a = new BigDecimal("0.1");
BigDecimal b = new BigDecimal("0.2");
BigDecimal sum = a.add(b);
System.out.println("BigDecimal sum: " + sum);
System.out.println("Is the sum equal to 0.3? " + sum.compareTo(new BigDecimal("0.3")) == 0);
}
}
Benefits of Using BigDecimal
- Exact Representation: Avoids precision issues inherent with floating point types.
- Controlled Rounding: Allows you to define the rounding behavior explicitly.
2. Be Cautious with Implicit Conversions
Java may perform implicit type conversions that can lead to inadvertent precision loss. Always specify types explicitly when dealing with floating point operations.
Example: Explicit Type Declaration
public class ExplicitTypeExample {
public static void main(String[] args) {
float f = 5.0f; // use 'f' to denote float
double d = 2.0;
double result = f / d;
System.out.println("Result: " + result);
}
}
3. Regularly Review Calculations
Consistently review any computations performed using floating point numbers. Always consider potential sources of error and document any special cases or considerations in your code.
Wrapping Up
Floating point numbers are an integral part of programming in Java, and while they offer flexibility, they also bring about significant pitfalls. Understanding these nuances can help you write more reliable and accurate code. By leveraging best practices such as using BigDecimal
for precise calculations, avoiding direct comparisons, and being aware of accumulative errors, you can sidestep many of the common issues that arise around floating point arithmetic.
Keep learning, keep coding, and remember that awareness is the first step toward mastery in any programming domain.
For further reading, consider checking out the official Java documentation on floating point arithmetic and resources on the IEEE 754 standard.
Feel free to share your experiences with floating point arithmetic in the comments below! What pitfalls have you encountered? How did you address them? Your insights may benefit the broader community.
Checkout our other articles