Avoid These Common Mistakes with Java's Optional Class
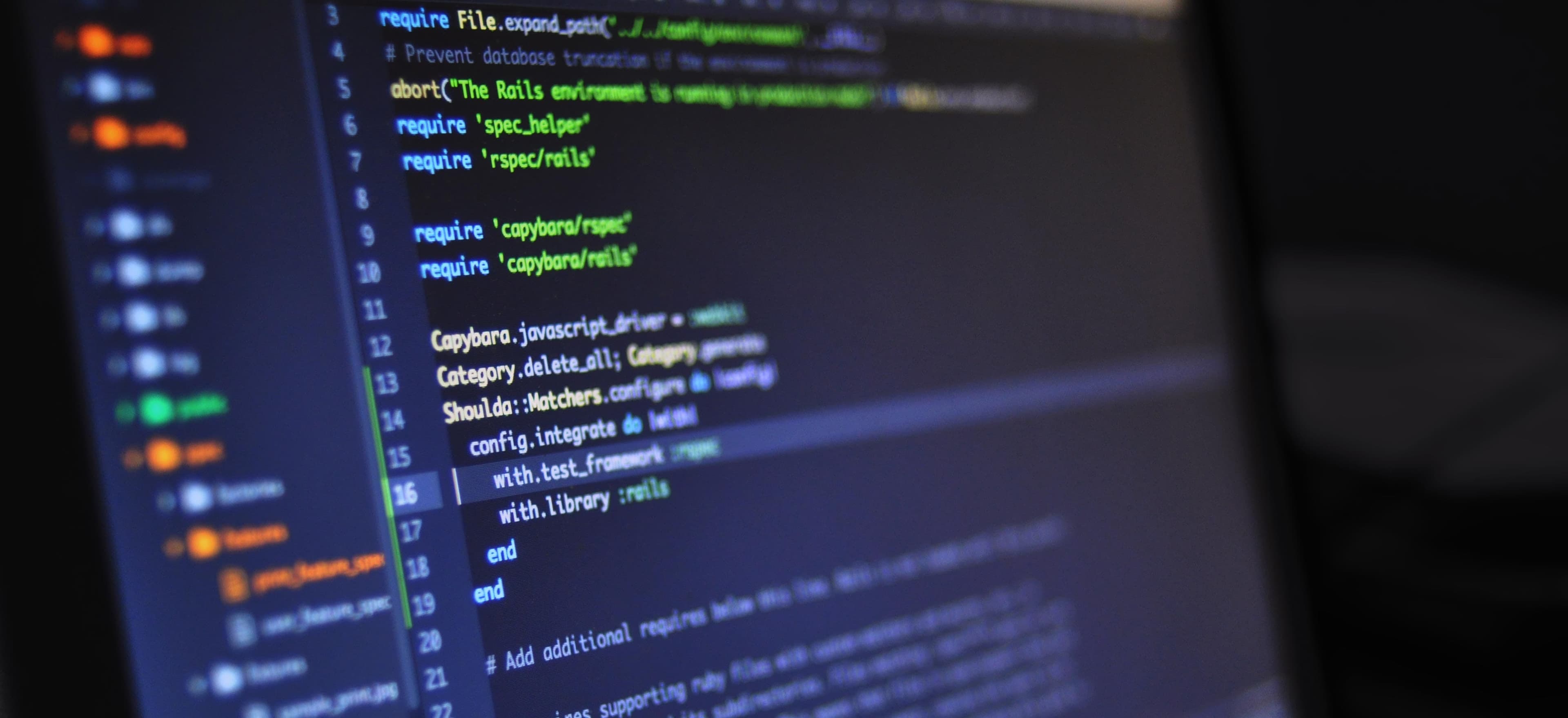
- Published on
Avoid These Common Mistakes with Java's Optional Class
Java's Optional
class, introduced in Java 8, aims to reduce the incidence of NullPointerExceptions
and help developers write cleaner, more readable code. However, despite its advantages, many programmers make common mistakes when using this feature. In this post, we'll explore these pitfalls and how to effectively leverage the Optional
class, with practical code samples that provide insight into best practices.
Understanding the Purpose of Optional
Before diving into mistakes, it's essential to understand why Optional
was introduced. In Java, null values have long been a source of errors. The Optional
class is designed to represent the notion of an available or absent value explicitly, thus providing a more robust solution.
Basic Syntax of Optional
To create an Optional
instance, you can either use Optional.of()
, Optional.empty()
, or Optional.ofNullable()
:
Optional<String> nonEmptyOptional = Optional.of("Hello, World");
Optional<String> emptyOptional = Optional.empty();
Optional<String> nullableOptional = Optional.ofNullable(null);
In this setup:
Optional.of()
throws an exception if the value is null.Optional.empty()
creates an emptyOptional
.Optional.ofNullable()
returns anOptional
that may be empty.
Common Mistakes Using Optional
Let's explore some common missteps developers find themselves in while working with Optional
.
Mistake 1: Overusing Optional in Method Parameters
Many developers mistakenly use Optional
as a method parameter type. This practice can lead to unnecessary complexity and reduces the clarity of the API.
Example:
public void process(Optional<String> name) {
// Logic here
}
Why It's a Mistake
Using Optional
in method parameters signifies that the caller must potentially deal with an absent value, which could lead to confusion. A better approach is to simply accept the concrete type or null
as acceptable input and handle it internally.
Correct Approach
Instead, you should handle the possibility of absence within the method:
public void process(String name) {
if (name == null) {
// Handle the absence of value appropriately
}
// Proceed with the processing logic
}
Mistake 2: Ignoring Optional's Functional Operations
Another frequent oversight is not taking advantage of the functional capabilities that Optional
provides. Many ignore methods like map
, flatMap
, and filter
, opting instead to write verbose null-checking code.
Example:
Optional<String> possibleValue = Optional.of("Hello");
if (possibleValue.isPresent()) {
System.out.println(possibleValue.get());
}
Why It's a Mistake
This lengthy approach is not only verbose but also defeats the purpose of using Optional
. Instead, utilize its functional style:
Correct Approach
optionalValue.map(value -> value.toUpperCase())
.ifPresent(System.out::println);
This approach is more concise and expressive. With functional operations, you can handle the value seamlessly without cluttering your logic with null checks.
Mistake 3: Misusing get() Method
The get()
method is often misused, especially when developers rely on it without first checking if the value is present.
Example:
Optional<String> optionalValue = Optional.ofNullable(null);
String value = optionalValue.get(); // Throws NoSuchElementException
Why It's a Mistake
Using get()
without confirming presence can easily lead to runtime exceptions, defeating the purpose of Optional
.
Correct Approach
Always check for the presence of the value before calling get()
or prefer using the built-in methods designed for this:
String value = optionalValue.orElse("Default Value");
System.out.println(value);
Here, orElse()
provides a default value if the Optional
is empty, helping you avoid exceptions.
Mistake 4: Using Optional for Collections
Many developers find it tempting to wrap collections within an Optional
, which can lead to confusion and exception-prone code.
Example:
Optional<List<String>> optionalList = Optional.of(new ArrayList<>());
Why It's a Mistake
This design does not leverage the benefits of Optional
. If the list is empty, it's usually more appropriate to just return an empty list.
Correct Approach
List<String> list = getList(); // returns an empty list or a populated one
if (!list.isEmpty()) {
// Process list
}
Returning an empty collection directly allows you to avoid overusing Optional
, streamlining your code.
Mistake 5: Combining Optional with Other Nullability Solutions
Using Optional
in conjunction with other methods for handling null can create confusion and complicate your code unnecessarily.
Example:
String result = Optional.ofNullable(value).orElseGet(() -> fetchValue());
Why It's a Mistake
By combining multiple solutions, you might dilute the purpose of having an Optional
in the first place.
Correct Approach
Choose one strategy and stick to it. If you decide to utilize Optional
, do so consistently:
String result = Optional.ofNullable(value).orElse("Default Value");
My Closing Thoughts on the Matter
Java's Optional
is a powerful tool designed to help developers manage the presence or absence of values effectively. However, to maximize its benefits, it’s crucial to avoid the common pitfalls we discussed above. By following best practices, you can write cleaner and more maintainable code that aligns with Java's design principles.
For more insights on using Optional
, consider checking out the official Java documentation or the Effective Java book by Joshua Bloch, which provides in-depth advice on best practices in Java programming.
By remaining mindful of these mistakes and adopting best practices, you can enhance both your code quality and your understanding of Java's Optional
class. Happy coding!