Preventing Java Native Memory Leaks When Using JNI
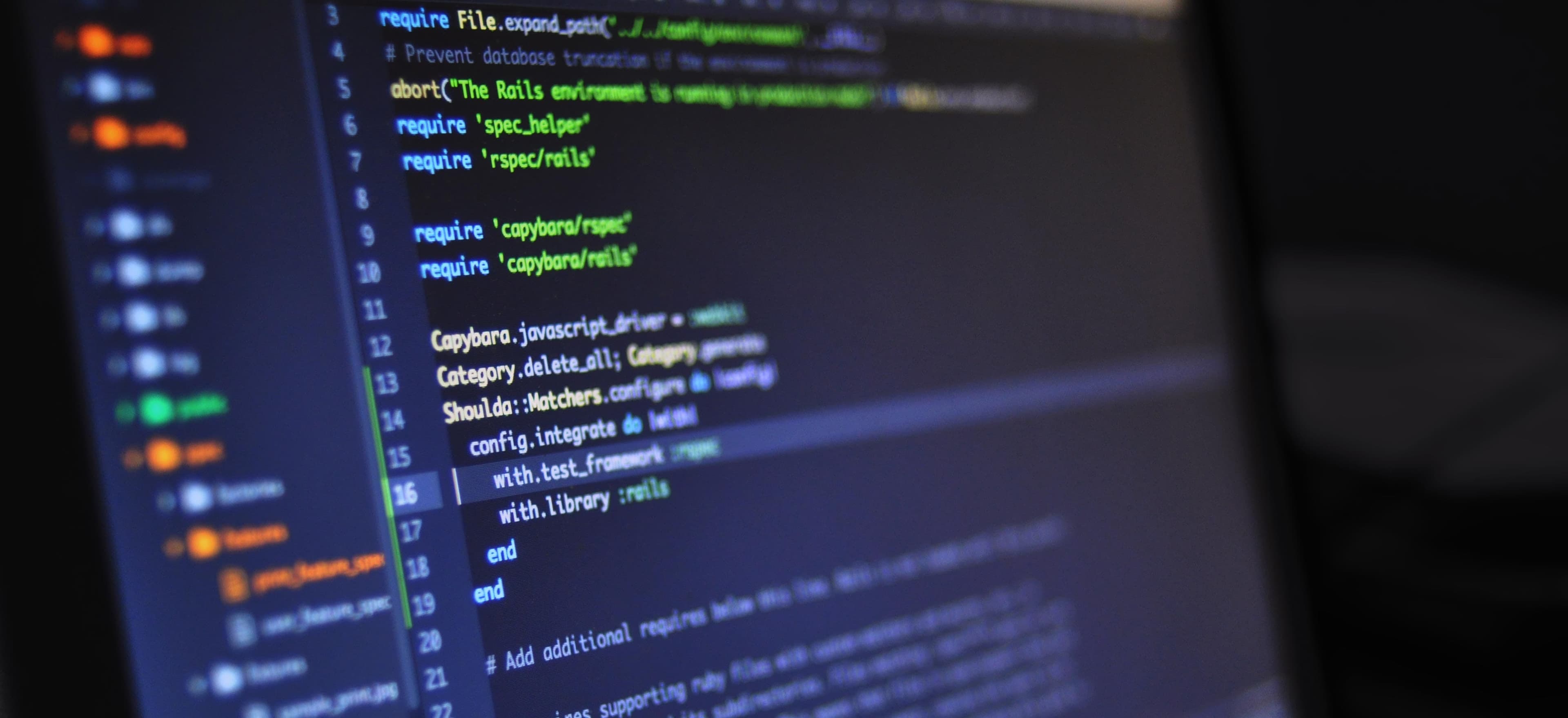
- Published on
Preventing Java Native Memory Leaks When Using JNI
Java Native Interface (JNI) allows Java code to interact and communicate with applications and libraries written in other languages, such as C or C++. While JNI is a powerful tool, it introduces complexities, particularly with memory management. One of the most critical issues developers face when using JNI is memory leaks. In this blog post, we will explore what native memory leaks are, why they occur, and how to prevent them effectively.
Understanding Java Native Memory Leaks
Native Memory refers to memory that is allocated outside of the Java Virtual Machine (JVM) heap. Unlike the memory managed by the JVM, native memory does not undergo automatic garbage collection. In the context of JNI, native memory is often allocated via C/C++ APIs, and if it's not managed correctly, it can lead to permanent memory leaks that may crash applications or degrade their performance over time.
What is a Memory Leak?
A memory leak occurs when a program consumes memory but fails to release it after use. In Java, this generally does not pose an issue because the Garbage Collector (GC) handles memory management. However, when using JNI, developers must manually allocate and deallocate native memory, increasing the risk of leaks.
Common Causes of Native Memory Leaks with JNI
-
Failure to Release Allocated Memory: A common issue arises when developers forget to free native memory once they are done using it.
// Example: Allocating memory in C int* array = (int*) malloc(10 * sizeof(int)); // ... use the array ... // Missing free(array);
-
Repeated Allocations: Continuously allocating memory without corresponding releases.
-
Mismanagement of JNI Reference Types: JNI allows for different types of references (local, global, and weak). If these references aren’t managed properly, it can lead to increased memory usage.
-
Exceptions Not Handled: If an exception occurs after memory allocation but before it is released.
Best Practices to Prevent Native Memory Leaks
1. Always Release Your Memory
The golden rule for managing native memory is: Always release what you allocate. In C/C++, this typically means using free()
for memory allocated with malloc()
or delete
for memory allocated with new
.
void releaseMemory(int* array) {
if (array != NULL) {
free(array);
}
}
In your JNI code, ensure that after each JNI call that allocates memory, there is a corresponding deallocation.
public native void useNative();
public void performAction() {
useNative(); // allocates memory in C/C++
// Do other work...
// Ensure native memory is released properly after use
}
2. Use JNI Reference Types Wisely
When dealing with JNI, it is crucial to understand reference types. Local references are automatically released when the native method exits, but global references need to be cleared manually.
Creating a global reference requires using NewGlobalRef()
:
jobject global_ref = (*env)->NewGlobalRef(env, local_ref);
// Utilize global_ref...
(*env)->DeleteGlobalRef(env, global_ref); // Release it after use
3. Check for Exceptions
When working with JNI, checking for exceptions after each JNI call is vital. If an exception occurs, memory might not be freed properly.
if ((*env)->ExceptionCheck(env)) {
(*env)->ExceptionClear(env); // clear the exception
free(array); // Clean up resources
return; // or handle accordingly
}
4. Utilize Smart Pointers (C++ Only)
If you're using C++, leverage smart pointers (like std::unique_ptr
) for automatic memory management, which reduces manual intervention and thus the potential for leaks.
std::unique_ptr<int[]> array(new int[10]);
// No need to manually free; it will be automatically freed.
5. Comprehensive Testing
Perform rigorous testing, particularly around areas that allocate and deallocate memory. Tools like Valgrind can help you identify memory leaks in your native code.
valgrind --leak-check=full java -jar YourJarFile.jar
6. Use Appropriate Libraries
Consider using libraries that handle JNI interactions and can manage memory automatically, such as JavaCPP.
7. Leverage Profilers
Utilize profilers that can track native memory usage, like YourKit or the built-in memory profiling tools in IDEs like IntelliJ IDEA.
Example
Let's walk through a simple example of how to interact with native memory correctly:
Native Code (C)
#include <jni.h>
#include <stdlib.h>
#include "YourClass.h"
JNIEXPORT jfloatArray JNICALL Java_YourClass_getFloatArray(JNIEnv* env, jobject obj) {
jfloatArray array = (*env)->NewFloatArray(env, 10);
if (array == NULL) {
return NULL; // Out of memory
}
float* data = (float*)malloc(10 * sizeof(float));
for (int i = 0; i < 10; i++) {
data[i] = i * 1.0f;
}
(*env)->SetFloatArrayRegion(env, array, 0, 10, data);
free(data); // Free the allocated memory
return array; // Return the filled array
}
JNIEXPORT void JNICALL Java_YourClass_nativeCleanup(JNIEnv* env, jobject obj) {
// Perform any required cleanup here
}
Java Code
public class YourClass {
static {
System.loadLibrary("YourLibrary");
}
public native float[] getFloatArray();
public static void main(String[] args) {
YourClass instance = new YourClass();
float[] values = instance.getFloatArray();
// Use the values...
// Cleanup and release native resources if necessary
}
}
Bringing It All Together
Preventing memory leaks in JNI requires diligence and proper understanding of both Java and native languages. By adhering to best practices for memory allocation and deallocation, utilizing exception handling correctly, and leveraging tools for profiling and testing, developers can significantly mitigate the risk of native memory leaks.
Always remember: with great power comes great responsibility. JNI is a powerful tool, but it must be wielded with care. By following the guidelines outlined in this post, you can create JAVA applications that interact with native code without the pitfalls of memory leaks.
For further exploration of JNI, check out the JNI Documentation that provides a comprehensive guide to its usage and best practices.
Feel free to share your experiences and suggestions on managing JNI memory in the comments below!
Checkout our other articles