Mastering Edge Cases in Java Regex: Common Pitfalls Revealed
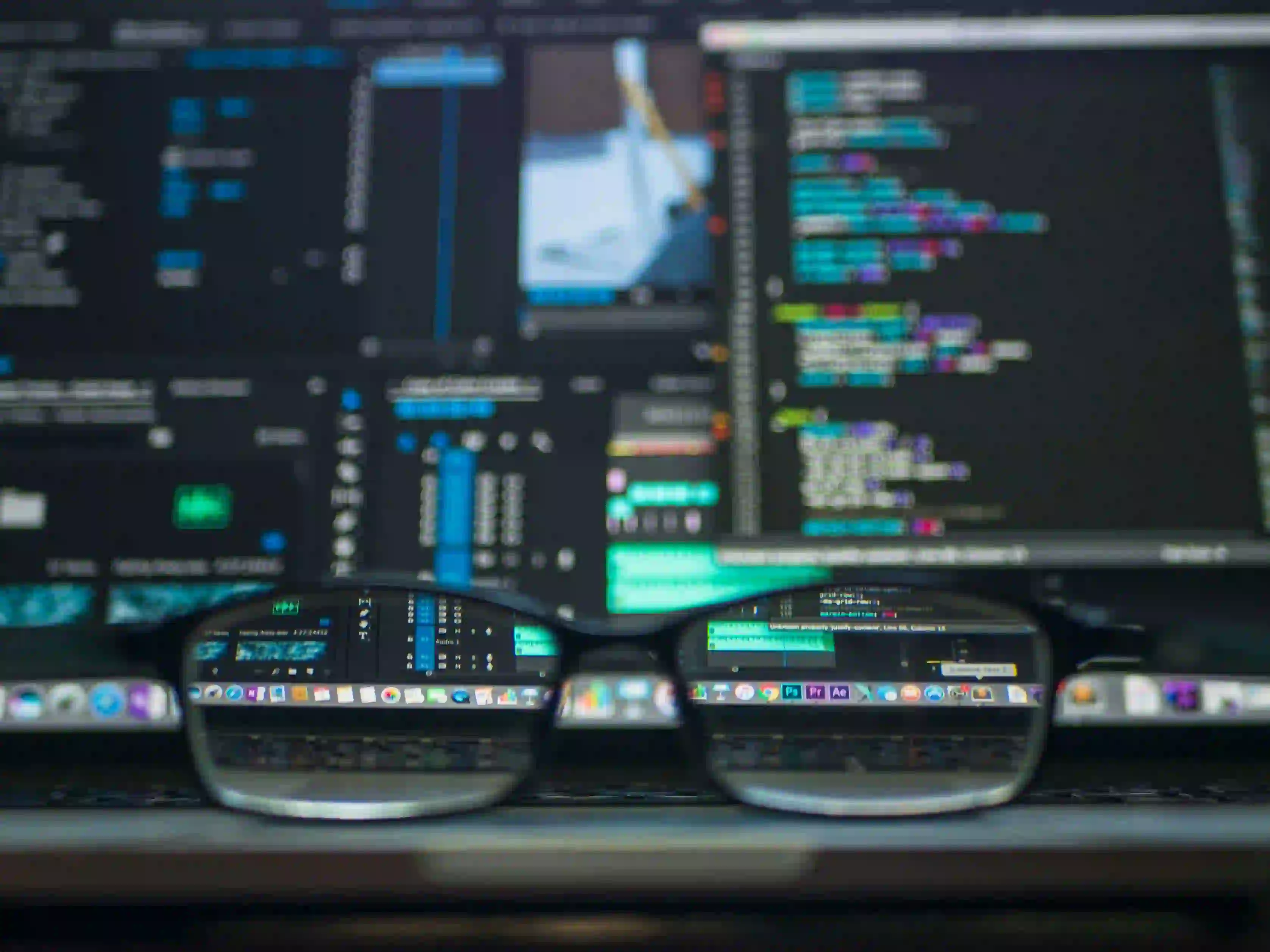
Mastering Edge Cases in Java Regex: Common Pitfalls Revealed
Regular expressions (regex) are a powerful tool in Java for string manipulation and validation. However, mastering them requires not only understanding the syntax but also knowing how to handle edge cases effectively. In this blog post, we'll explore common pitfalls in Java regex usage, how to identify edge cases, and provide best practices with code examples.
Understanding Java Regex Basics
Before diving deep into edge cases, let’s begin with a brief refresher on Java regex. Java provides the java.util.regex
package which includes classes like Pattern
and Matcher
.
Quick Syntax Review
Here are some basic regex constructs you'll encounter:
.
: Matches any character except a newline.^
: Anchors to the start of a string.$
: Anchors to the end of a string.*
: Matches 0 or more occurrences of the preceding element.+
: Matches 1 or more occurrences of the preceding element.?
: Matches 0 or 1 occurrence of the preceding element.[]
: Matches any one character within the brackets.
import java.util.regex.*;
public class RegexBasics {
public static void main(String[] args) {
String input = "abc123";
Pattern pattern = Pattern.compile("[a-z]+\\d+");
Matcher matcher = pattern.matcher(input);
if (matcher.matches()) {
System.out.println("Match found!");
} else {
System.out.println("No match.");
}
}
}
The above example checks if the input string consists of lowercase letters followed by digits.
Common Pitfalls in Regex Usage
1. Not Anchoring Your Regex
Failing to anchor your regex can lead to unintended matches.
Example
Pattern pattern = Pattern.compile("abc");
This will find any occurrence of "abc" within a string. If you only want to match strings that exactly equal "abc", you should use:
Pattern pattern = Pattern.compile("^abc$");
2. Ignoring Whitespace
Another common issue is not accounting for whitespace. This can lead to unexpected failures in matching.
Example
Suppose we want to match a string that starts with "Hello" and ends with "World", allowing for spaces in between:
Pattern pattern = Pattern.compile("^Hello\\s+World$");
Using the above regex, it will match "Hello World", "Hello World", but not "HelloWorld" or "Hello Universe".
3. Misusing .
Operator
The dot operator (.
) does not match newline characters.
Example
String input = "Hello\nWorld";
Pattern pattern = Pattern.compile("Hello.World");
This regex will not match because there’s a newline between "Hello" and "World". To include newlines, consider using the (?s)
flag:
Pattern pattern = Pattern.compile("(?s)Hello.World");
4. Neglecting Escape Characters
Certain characters, like ., *, ?, (, ), [, and | have special meanings in regex. If you're trying to match them literally, you must escape them with a backslash.
Example
String input = "1 + 2 = 3";
Pattern pattern = Pattern.compile("\\d \\+ \\d = \\d");
In the above case, each special character is escaped to ensure they are treated as literals.
Exploring Edge Cases
1. Empty Strings
An empty string can produce conflicting results depending on the context. By default, regex patterns allow for empty matches unless expected otherwise.
Example
String input = "";
Pattern pattern = Pattern.compile(".*");
Matcher matcher = pattern.matcher(input);
if (matcher.matches()) {
System.out.println("Matches an empty string");
}
A pattern of .*
matches zero or more occurrences, hence it will match an empty string. Depending on your needs, you may want to be more explicit if you wish to avoid matching empty strings.
2. Overlapping Matches
An interesting pitfall occurs with overlapping matches where a regex may unintentionally match part of another match.
Example
String input = "ABAB";
Pattern pattern = Pattern.compile("AB");
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
System.out.println("Found: " + matcher.group());
}
The output will be:
Found: AB
Found: AB
Here, the second match overlaps with the first. This may not always be the desired behavior, and thus understanding how your regex captures these overlaps is essential.
3. Lookaheads and Lookbehinds
Lookaheads and lookbehinds can help manage complex matching scenarios. They can be tricky due to their non-consuming nature.
Example
String input = "hello123";
Pattern pattern = Pattern.compile("(?=\\d)");
Matcher matcher = pattern.matcher(input);
if (matcher.find()) {
System.out.println("Number found");
}
This pattern checks for the presence of a digit without consuming it.
4. Unicode and Non-ASCII Characters
Java's regex also has support for Unicode. However, it is often misunderstood.
Example
To match a string containing a Unicode character:
String input = "café";
Pattern pattern = Pattern.compile("caf\\u00E9"); // 'é' in Unicode
Matcher matcher = pattern.matcher(input);
if (matcher.matches()) {
System.out.println("Match found: " + input);
}
Ensure your regex considers potential non-ASCII characters when writing patterns.
Best Practices for Regex in Java
- Always Test Your Patterns: Use tools like regex101.com to test your expressions before implementing them in your code.
- Readability: Sometimes, complex patterns can be hard to read. Use comments and break down the regex into simpler components if possible.
- Performance Optimization: Complex regex patterns can lead to performance issues, especially with backtracking. Always aim for efficiency.
- Avoid Greedy Matching: Be cautious about using greedy quantifiers (like
*
and+
) unless necessary. Prefer non-greedy variants (*?
and+?
) when appropriate.
A Final Look
Mastering Java regex involves understanding not only those structures and patterns but also recognizing how to handle edge cases and common pitfalls. By following the guidance in this post and employing well-structured regex, you'll be equipped to tackle a wide array of string manipulation tasks with precision.
For more information on regex in Java, consider visiting Java Regex Tutorial.
With practice and careful design, regex can be a vital tool in your Java arsenal, opening doors to robust string processing and validation for your applications.