Mastering Unchecked Exceptions in Java Streams API
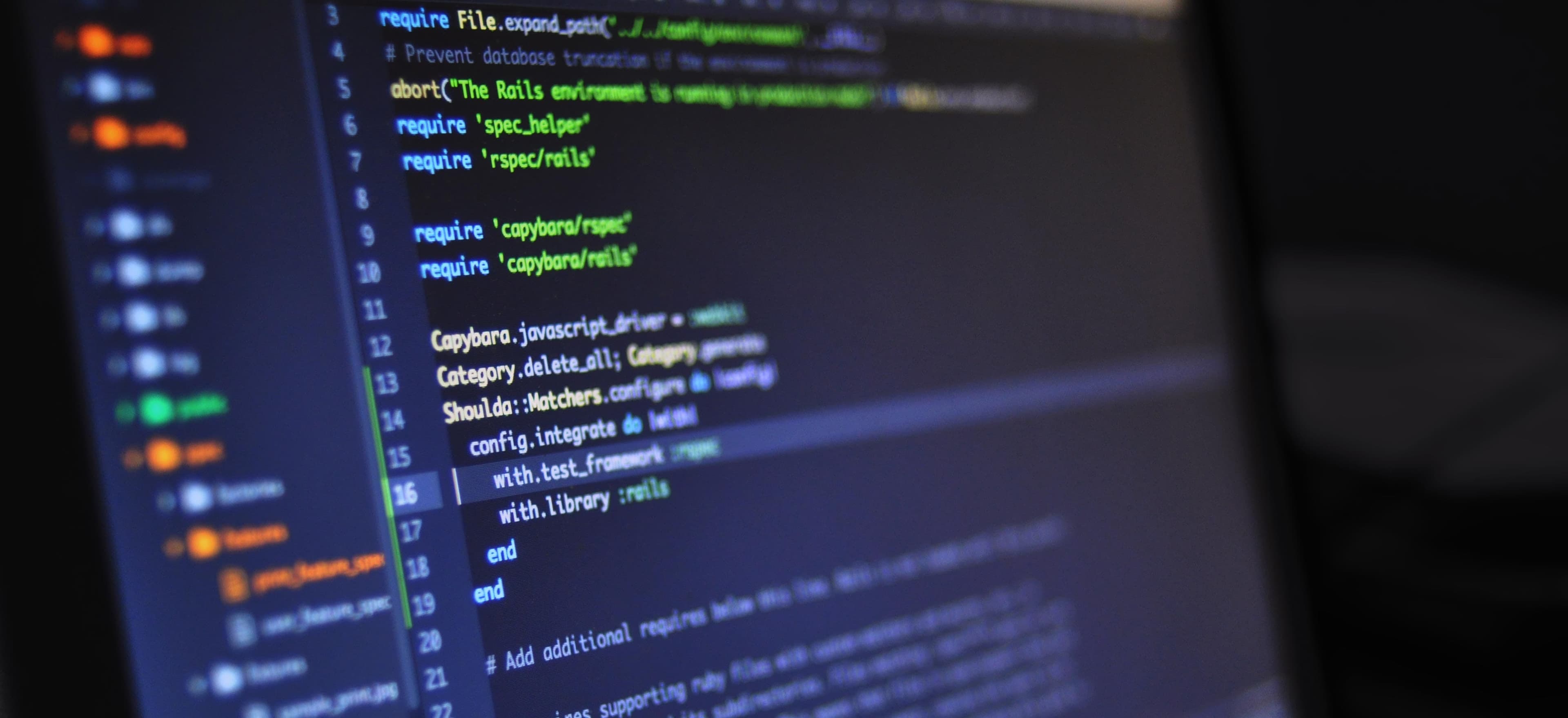
- Published on
Mastering Unchecked Exceptions in Java Streams API
Java Streams API, introduced in Java 8, revolutionized the way we handle collections, providing a rich, fluent interface for processing data. With its advantages come intricacies, particularly when dealing with exceptions. Understanding unchecked exceptions in the context of Java Streams can greatly enhance your ability to write robust and maintainable code.
In this blog post, we will explore unchecked exceptions in Java Streams, focusing on scenarios when they might occur, how to manage them effectively, and best practices to avoid pitfalls. We will also incorporate practical examples that highlight best practices during your streams manipulation.
What are Unchecked Exceptions?
Unchecked exceptions are exceptions that are not checked at compile-time. This means the compiler does not require you to handle these exceptions (e.g., IOException, NullPointerException). They often result from programming errors and can be prevented through careful coding practices.
On the other hand, checked exceptions are anticipated and can be caught or declared to be thrown. Unchecked exceptions can originate from legitimate issues in your data, making them critical to handle properly in streams.
Common Unchecked Exceptions in Streams
- NullPointerException: This is the most common unchecked exception that arises when a program attempts to access an object or call a method on a null reference.
- ClassCastException: Occurs when you try to cast an object to a subtype to which it is not an instance.
- IllegalArgumentException: Typically thrown to indicate the method has been passed an illegal or inappropriate argument.
- ArrayIndexOutOfBoundsException: Triggered when an array has been accessed with an illegal index.
The Importance of Exception Handling in Streams
When using Streams API, it is imperative to handle exceptions effectively because:
- Streams operations are often chained, making it hard to pinpoint where an exception occurred.
- Unchecked exceptions can cause entire operations to fail, thus terminating your program unexpectedly.
- Managing exceptions properly can improve user experience by providing meaningful feedback instead of a crash.
Working with Streams: Best Practices to Handle Exceptions
Let us dive into the best practices for handling unchecked exceptions in Java Streams. I'll provide various examples and insights to guide you through potential pitfalls.
1. Using try-catch Within Lambda Expressions
Using a try-catch block within your lambda can allow you to handle an exception gracefully.
List<String> numbers = Arrays.asList("1", "2", "3", "a", "5");
List<Integer> result = numbers.stream()
.map(s -> {
try {
return Integer.valueOf(s);
} catch (NumberFormatException e) {
System.err.println("Invalid number: " + s);
return null;
}
})
.filter(Objects::nonNull)
.collect(Collectors.toList());
System.out.println(result); // Output: [1, 2, 3, 5]
In this example, we handle the NumberFormatException
directly within the map function. Unhandled invalid input will not cause program failures, and we return null
for invalid inputs, later filtered out.
2. Creating Custom Wrapper Methods
If your stream processing might lead to common exceptions, consider creating utility methods to encapsulate exception handling.
public class StreamUtils {
public static <T, R> Function<T, R> wrap(Function<T, R> function) {
return t -> {
try {
return function.apply(t);
} catch (Exception e) {
System.err.println("Error processing: " + t + " - " + e.getMessage());
return null;
}
};
}
}
// Usage
List<Integer> safeResult = numbers.stream()
.map(StreamUtils.wrap(Integer::valueOf))
.filter(Objects::nonNull)
.collect(Collectors.toList());
System.out.println(safeResult); // Output: [1, 2, 3, 5]
Here, we defined a utility method wrap
that allows us to clean up our stream processing code while handling exceptions uniformly.
3. Logging Exceptions Properly
Logging is crucial for diagnosing issues in production. Make sure to log exceptions meaningfully wherever necessary.
import java.util.logging.Logger;
public class StreamExample {
private static final Logger logger = Logger.getLogger(StreamExample.class.getName());
public static void main(String[] args) {
List<String> values = Arrays.asList("1", "2", "three", "4");
values.stream()
.map(s -> {
try {
return Integer.valueOf(s);
} catch (NumberFormatException e) {
logger.severe("Failed to parse: " + s);
return null;
}
})
.filter(Objects::nonNull)
.forEach(System.out::println); // Output: 1, 2, 4
}
}
Logging gives a full trail of what went wrong, informing stakeholders without causing temporary loss of service.
4. Avoiding Modification of Input Data During Stream Operations
Often, ConcurrentModificationException
can occur if you modify the collection while using it within a stream. Avoid modifying the collection or use concurrent collections instead.
List<String> inputList = new ArrayList<>();
inputList.add("Hello");
inputList.add("World");
inputList.stream()
.map(String::toUpperCase)
.forEach(s -> inputList.add(s)); // This will throw ConcurrentModificationException
Changing your data during a stream operation violates the internal iteration principle. If you need to apply changes, make a copy of the list before the stream operation.
5. Using Optional for Better Null Handling
Using Optional
can assist in handling null values effectively, preventing NullPointerException
without explicit checks.
List<String> optionalNumbers = Arrays.asList("1", "2", "three", "4");
List<Integer> optionals = optionalNumbers.stream()
.map(s -> Optional.ofNullable(s)
.map(Integer::valueOf)
.orElse(null))
.filter(Objects::nonNull)
.collect(Collectors.toList());
System.out.println(optionals); // Output: [1, 2, 4]
By employing Optional
, the need for null checks becomes a lot simpler and cleaner.
Closing the Chapter
Mastering unchecked exceptions in Java Streams API is essential for creating resiliency in your applications. With best practices that include effective logging, custom wrapper methods, and the use of Optional
, you can write clearer and more maintainable code.
Helpful Resources
By following these guidelines, you’ll enhance your skillset and contribute towards the development of robust Java applications. Happy coding!