Mastering Java Socket Timeouts: Common Issues & Fixes
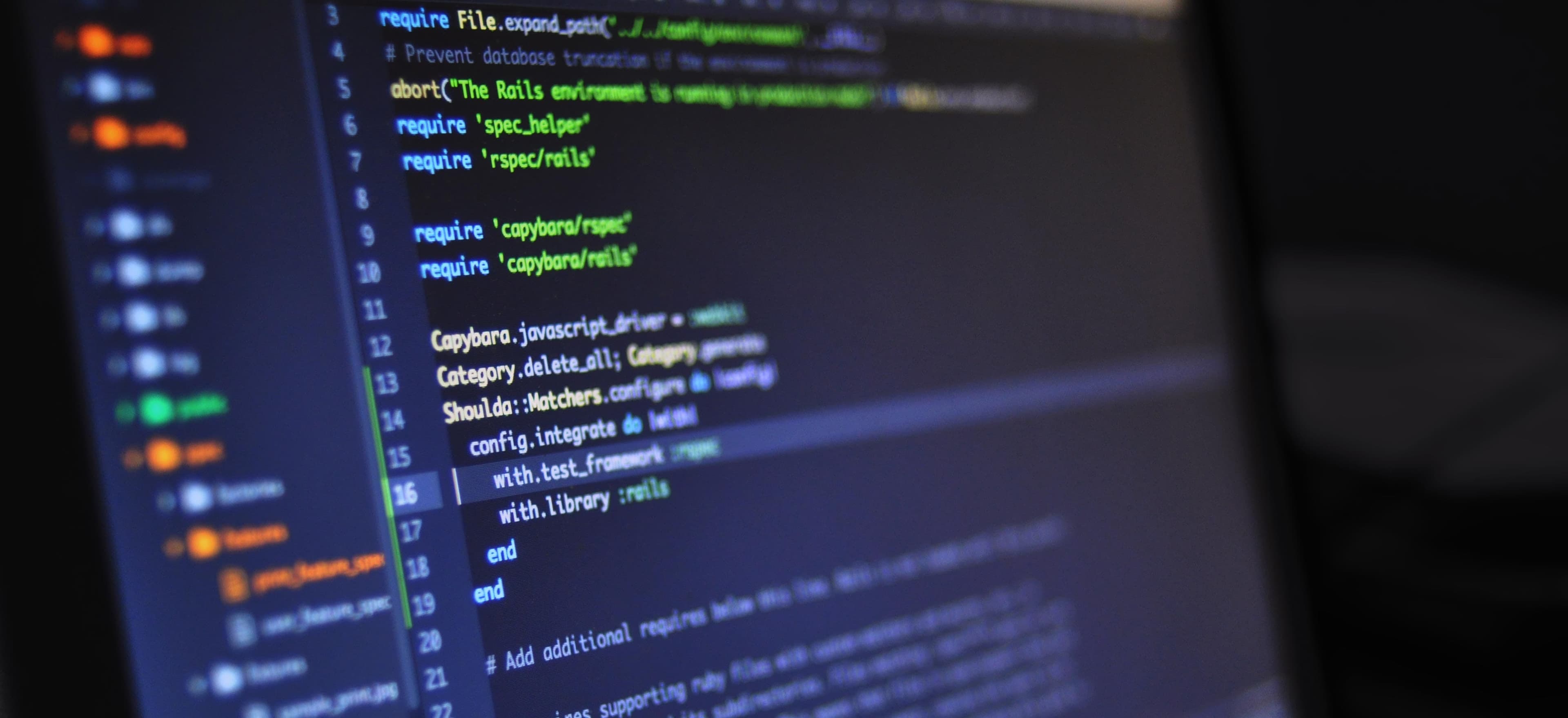
- Published on
Mastering Java Socket Timeouts: Common Issues & Fixes
As technology advances, network communication becomes integral to virtually every application. Java enhances this experience with its built-in support for sockets—enabling seamless communication between devices. However, one aspect that often causes confusion among developers is socket timeouts. This post will cover the fundamentals of Java Socket Timeouts, dive into common issues, discuss fixes, and provide practical code snippets for better understanding.
Understanding Java Sockets
Java sockets are a way for software applications to communicate over a network. They are part of the Java networking API, allowing data transfer using TCP/IP or UDP protocols. A socket includes an IP address and a port number, which uniquely identifies the endpoint of the communication.
The Importance of Timeouts
Timeouts play a crucial role in network communication by preventing operations from hanging indefinitely. They set a limit on how long the socket will wait for a response, protecting your application from delays and improving user experience.
Common Issues with Socket Timeouts
-
Connection Timeout: This occurs when a connection cannot be established within a specified time frame. It can happen due to network congestion or if the server is unresponsive.
-
Read Timeout: This happens when a socket is open but doesn't receive data. If the server fails to send data within a certain period, the read operation times out.
-
Write Timeout: Similar to read timeouts, but when attempting to send data to the server. If the server cannot accept data within a specified time, the write operation will fail.
Example: Setting Timeouts in Java Sockets
Setting socket timeouts is straightforward in Java. The default sockets can be modified to include connection and read timeouts, which helps manage network latency effectively.
import java.io.IOException;
import java.net.Socket;
import java.net.SocketTimeoutException;
public class TimeoutExample {
public static void main(String[] args) {
try {
Socket socket = new Socket();
// Set the connection timeout to 5 seconds
socket.connect(new InetSocketAddress("example.com", 80), 5000);
// Set the read timeout to 10 seconds
socket.setSoTimeout(10000);
} catch (SocketTimeoutException e) {
System.out.println("Socket timeout: " + e.getMessage());
} catch (IOException e) {
System.out.println("I/O error: " + e.getMessage());
}
}
}
Commentary on the Code
-
socket.connect(...)
: The method takes the server address and port with a timeout value as its parameter. If the connection isn’t established in 5 seconds, aSocketTimeoutException
will be thrown. -
socket.setSoTimeout(...)
: This method sets the read timeout. In this case, if no data is read for 10 seconds, the socket will terminate the read operation.
Real-World Scenarios
Consider a web client that fetches data from an external API. If the API server is slow or down, connection and read timeouts will help you handle such situations gracefully. Using appropriate timeout configurations allows developers to retry requests or present informative messages to users.
Best Practices for Handling Socket Timeouts
-
Always Set a Timeout: Even if you're on a reliable network, always specify a timeout to avoid unexpected delays.
-
Exponential Backoff for Retries: If a timeout occurs, implement a retry mechanism with exponential backoff. This approach prevents flooding the server with repeated requests.
-
Detailed Error Handling: Use try-catch blocks to handle exceptions gracefully, informing users of timeouts without crashing the application.
-
Logging: Implement logging to capture timeout events, helping you diagnose network issues down the line.
Troubleshooting Common Timeout Issues
1. Increasing Timeout Values
Sometimes, connection issues persist even after adjusting timeout values. Consider whether your timeouts are too short for the task at hand.
// Increase connection timeout to 10 seconds and read timeout to 30 seconds
socket.connect(new InetSocketAddress("example.com", 80), 10000);
socket.setSoTimeout(30000);
2. Verifying Network Connectivity
Perform basic checks like pinging the server or using tools like traceroute
to identify potential connectivity issues.
3. Server Configuration
In some instances, server settings can contribute to timeout problems. Ensure that the server can handle incoming connections and that there are no excessive firewalls or network issues.
4. Use of Asynchronous Calls
Consider using asynchronous socket calls with the java.nio
package to improve responsiveness in your application. This is especially helpful in GUI applications where blocking operations can freeze the user interface.
Example: Using Asynchronous Sockets
Java NIO (New Input/Output) utilizes channels and buffers for a non-blocking way of handling sockets.
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.channels.SocketChannel;
public class AsyncSocketExample {
public static void main(String[] args) {
try {
SocketChannel socketChannel = SocketChannel.open();
socketChannel.configureBlocking(false); // Set to non-blocking mode
if (socketChannel.connect(new InetSocketAddress("example.com", 80))) {
System.out.println("Connection established!");
} else {
System.out.println("Attempting to connect...");
}
} catch (IOException e) {
System.out.println("Error during connection: " + e.getMessage());
}
}
}
Commentary on NIO
-
configureBlocking(false)
: This sets the socket to non-blocking mode. The connection attempt may take some time to complete, but the program proceeds without waiting. -
Connection Handling: The code checks if the connection is established. If not, it will continue its operations, allowing the program to remain responsive.
Closing Remarks
Mastering Java socket timeouts is a crucial skill for any developer working with network applications. By understanding the common issues related to timeouts and implementing best practices, you will enhance the reliability of your applications.
Explore additional resources to deepen your knowledge:
- Java Networking Tutorial
- Handling Socket Timeouts in Java
Integrate these concepts into your projects, adjust timeout settings as necessary, and you will successfully mitigate the frustrations that socket timeouts can bring. Happy coding!
Checkout our other articles