Solving Deadlock Problems in Java ExecutorService
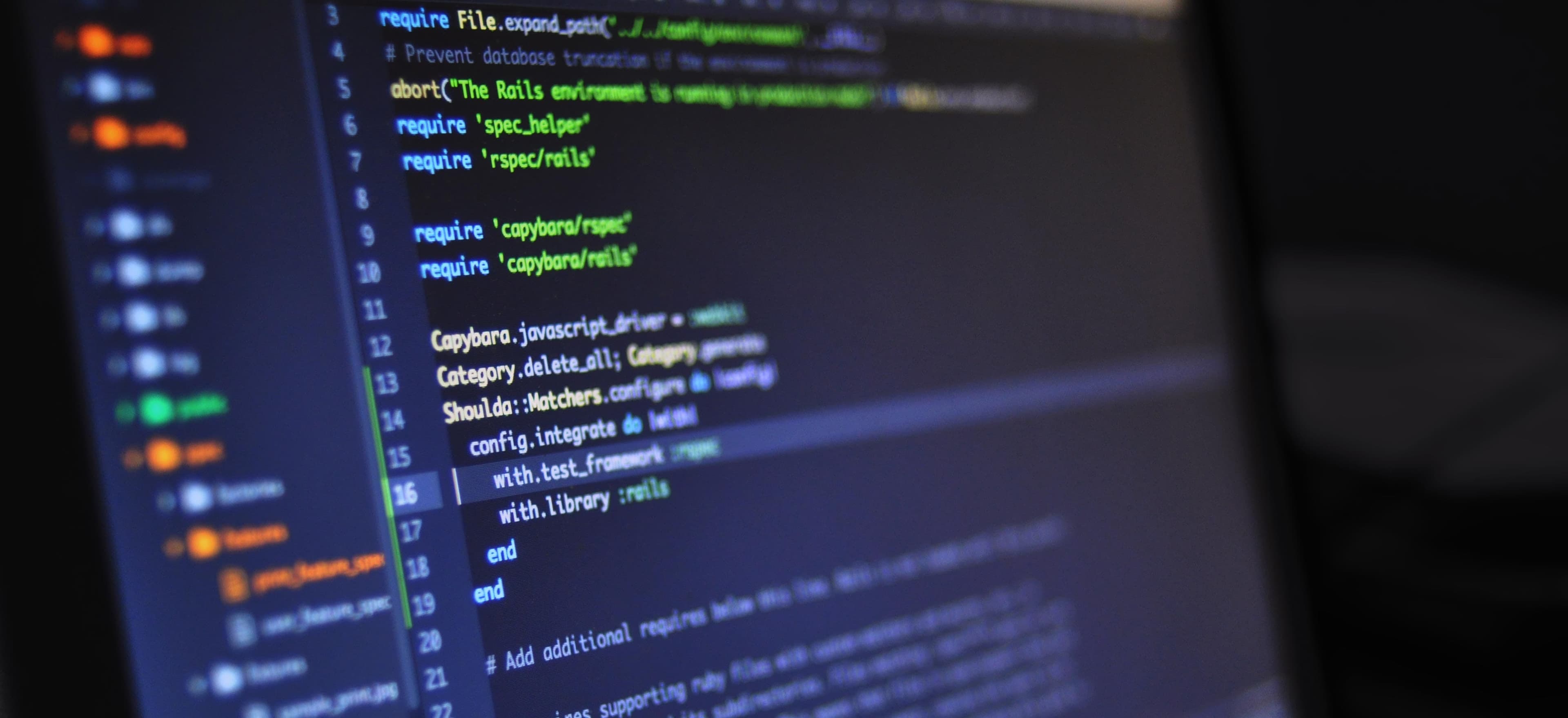
- Published on
Solving Deadlock Problems in Java ExecutorService
Deadlocks are one of the most notorious issues in concurrent programming, particularly when working with resources managed by an ExecutorService
. In Java, a deadlock occurs when two or more threads are blocked forever, each waiting for the other to release a resource that they need to continue execution. This can cause your application to hang, leading to poor user experience and high maintenance costs.
In this blog post, we’ll explore how to effectively prevent, detect, and resolve deadlock problems in Java, particularly when utilizing ExecutorService
. We’ll also provide working code snippets and best practices to ensure your applications remain responsive and efficient.
Understanding Deadlocks
Before diving into solutions, it helps to understand how deadlocks happen. A deadlock typically occurs in the following scenario:
- Thread A holds Resource 1 and waits for Resource 2.
- Thread B holds Resource 2 and waits for Resource 1.
Both threads end up in a stalemate. A well-known example of deadlock can be illustrated like this:
public class DeadlockExample {
static class Resource {
String name;
Resource(String name) { this.name = name; }
}
private static final Resource resource1 = new Resource("Resource 1");
private static final Resource resource2 = new Resource("Resource 2");
public static void main(String[] args) {
// Thread 1
Thread thread1 = new Thread(() -> {
synchronized (resource1) {
try { Thread.sleep(100); } catch (InterruptedException e) { }
synchronized (resource2) {
System.out.println("Thread 1: Acquired both resources");
}
}
});
// Thread 2
Thread thread2 = new Thread(() -> {
synchronized (resource2) {
synchronized (resource1) {
System.out.println("Thread 2: Acquired both resources");
}
}
});
thread1.start();
thread2.start();
}
}
In this example, to demonstrate a deadlock, we initially acquire one resource in each thread and then try to acquire the other, thus creating a deadlock situation.
How ExecutorService Contributes to Deadlocks
ExecutorService
can exacerbate deadlock situations since it manages a pool of threads. If threads within the pool are already busy acquiring locks on resources, any new tasks that depend on those locks will also be blocked.
Here are a few common scenarios resulting in deadlocks when using ExecutorService
:
- Long-running tasks that hold locks longer than necessary.
- Improper management of shared resources between tasks.
- Tasks that depend on the completion of each other in a cyclical manner.
Prevention Techniques
Preventing deadlocks is generally easier than resolving them after they occur. Here are a few strategies:
1. Lock Ordering
A common technique is to enforce a consistent order in which locks are acquired. By ensuring that all threads request resources in a predefined order, you can eliminate cyclic waiting conditions.
public class LockOrderingExample {
static class Resource {
String name;
Resource(String name) { this.name = name; }
}
private static final Resource resource1 = new Resource("Resource 1");
private static final Resource resource2 = new Resource("Resource 2");
public void executeTasks() {
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.execute(() -> {
synchronized (resource1) {
// enforce ordering
synchronized (resource2) {
System.out.println("Task 1 acquired both resources.");
}
}
});
executor.execute(() -> {
synchronized (resource1) {
// Same order of locking
synchronized (resource2) {
System.out.println("Task 2 acquired both resources.");
}
}
});
executor.shutdown();
}
}
2. Using Timeouts
When acquiring a lock, always use timeouts. This way, if a thread can't acquire a resource within a specific time, it can react rather than waiting indefinitely.
public class LockWithTimeout {
public static void executeWithTimeout() {
Lock lock1 = new ReentrantLock();
Lock lock2 = new ReentrantLock();
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.execute(() -> {
try {
if (lock1.tryLock(1, TimeUnit.SECONDS)) {
try {
if (lock2.tryLock(1, TimeUnit.SECONDS)) {
try {
System.out.println("Task 1 acquired both resources.");
} finally {
lock2.unlock();
}
}
} finally {
lock1.unlock();
}
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
executor.shutdown();
}
}
In this example, tryLock()
is employed with a timeout, preventing the thread from waiting forever if it cannot acquire the necessary locks.
3. Clear Resource Management
Make sure to manage resource acquisition and release explicitly. Always use finally
blocks to ensure that locks are released even when exceptions occur.
Detecting Deadlocks
When deadlocks occur, detecting them can be challenging. However, there are tools and techniques you can utilize to identify deadlocks more easily:
-
Thread Dumps - You can generate thread dumps (via JVisualVM or using
jstack
) to inspect the state of your application and see if any threads are blocked. -
Java Management Extensions (JMX) - Java provides built-in monitoring which can help you keep an eye on thread states, including blocked and waiting states.
-
Deadlock Detection Algorithms - Implement algorithms, such as resource allocation graphs, to detect the possibility of deadlocks at runtime.
Resolving Deadlocks
If you've run into a deadlock situation despite your best efforts, you can resolve it:
- Kill or Restart Processes - In critical systems, you might have to kill and restart the affected threads or the entire application.
- Adjust Resource Allocation - Modifying the way resources are locked or release strategies can help resolve deadlocks.
- Refactor - Sometimes a complete redesign of how you manage locks and resources is necessary. Consider if all resources truly need to be locked.
Closing the Chapter
In conclusion, managing deadlocks in Java ExecutorService
is vital for creating robust applications. By understanding deadlocks and employing techniques like lock ordering, timeouts, and proper resource management, you can prevent, detect, and resolve deadlock scenarios effectively.
For more detailed insights on Java concurrency problems, check out Java Concurrency in Practice and Effective Java.
By following these principles and maintaining a vigilant approach to thread management, you can mitigate the risks of deadlocks and enhance the performance and reliability of your Java applications. Happy coding!
Checkout our other articles