Mastering FileNotFoundException in Multithreaded Java Apps
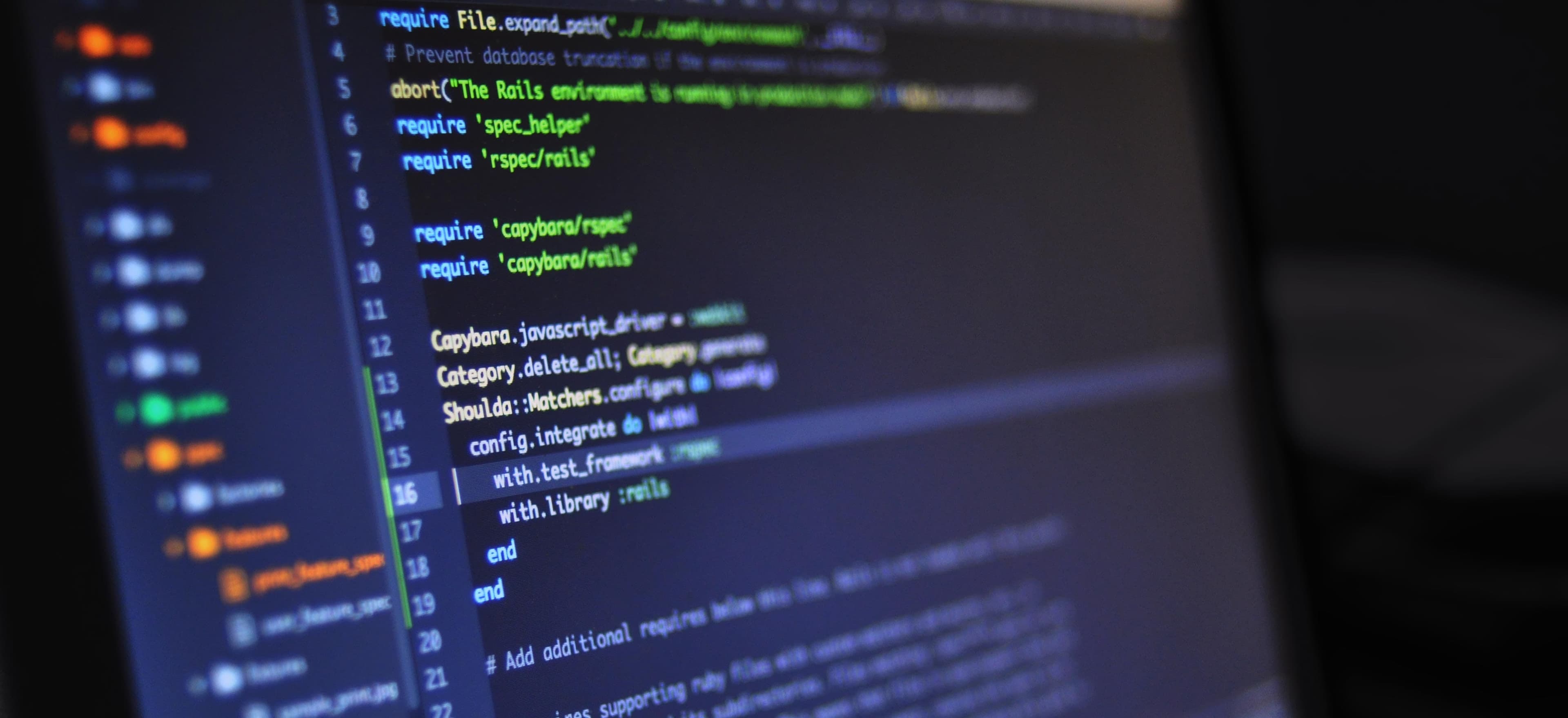
- Published on
Mastering FileNotFoundException in Multithreaded Java Apps
In the realm of Java programming, you have the power to create robust multithreaded applications. However, with great power comes great responsibility, especially in handling exceptions such as FileNotFoundException
. This article aims to provide an in-depth understanding of FileNotFoundException
in the context of multithreaded Java applications, alongside best practices to manage this exception effectively.
Understanding FileNotFoundException
A FileNotFoundException
is a checked exception in Java that occurs when an attempt to open the specified file denoted by a pathname fails. This can happen for reasons such as:
- The file does not exist.
- The path is incorrect.
- The application has insufficient permissions to access the file.
Why Handle Exceptions?
Handling exceptions in your code ensures that your application can respond gracefully to unexpected situations. Instead of just crashing, your application can inform the user, log the error, or take corrective actions.
A Basic Example
Let’s begin with a simple example of reading a file that might throw a FileNotFoundException
.
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileReaderExample {
public static void main(String[] args) {
File file = new File("example.txt");
try {
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine());
}
scanner.close();
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
}
}
}
Commentary on the Code
-
File Object Creation: We create a
File
object representing the intended file. If the file does not exist, trying to read it will throw aFileNotFoundException
. -
Try-Catch: By wrapping the file reading logic in a try-catch block, we can handle the
FileNotFoundException
gracefully. -
Error Reporting: The error message provides feedback to the user about the issue.
Multithreading and File Handling
Now, let’s delve into multithreading. In a multithreaded environment, multiple threads may attempt to access the same file simultaneously. When working with files, it's essential to manage concurrent access to prevent data corruption or inconsistent state.
Example of Multithreading with File Handling
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
class FileReadingThread extends Thread {
private String filePath;
public FileReadingThread(String filePath) {
this.filePath = filePath;
}
@Override
public void run() {
File file = new File(filePath);
try {
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
System.out.println(Thread.currentThread().getName() + ": " + scanner.nextLine());
}
scanner.close();
} catch (FileNotFoundException e) {
System.err.println(Thread.currentThread().getName() + ": File not found - " + e.getMessage());
}
}
}
public class MultithreadedFileReader {
public static void main(String[] args) {
Thread thread1 = new FileReadingThread("example.txt");
Thread thread2 = new FileReadingThread("example.txt");
thread1.start();
thread2.start();
}
}
Key Insights from the Multithreading Code
-
Thread Creation: Each thread is created to read the same file. This mimics a scenario where multiple threads could compete for file access.
-
Output Identification: The use of
Thread.currentThread().getName()
helps distinguish between the output of different threads, providing clear visibility into which thread is executing. -
Handling Exception: Each thread independently handles its
FileNotFoundException
, ensuring that one thread's failure does not affect others.
Concurrency Issues
Running multiple threads to read from a file may introduce race conditions and cause unexpected behaviors such as data inconsistency. Here are some best practices to mitigate these issues:
1. Synchronized Blocks
Using synchronized blocks can help control access to shared resources.
private synchronized void readFile(String filePath) {
// File reading logic
}
2. Using File I/O Libraries
Consider Java NIO or Apache Commons IO for better performance with file operations. These libraries offer higher-level abstractions that handle concurrency seamlessly.
Learn more about Java NIO here.
3. Thread Pooling
Utilize ExecutorService
instead of managing threads manually. This approach allows you to limit the number of concurrent threads, improving resource management.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolFileReader {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(2);
executor.submit(new FileReadingThread("example.txt"));
executor.submit(new FileReadingThread("example.txt"));
executor.shutdown();
}
}
Logging the Exceptions
Effective logging of exceptions is crucial for debugging purposes. Instead of printing stack traces to the console, consider using a logging framework like SLF4J or Log4j.
Example of logging:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class FileReaderExample {
private static final Logger logger = LoggerFactory.getLogger(FileReaderExample.class);
// In the try-catch block
logger.error("File not found: ", e);
You can learn more about SLF4J here.
To Wrap Things Up
Handling FileNotFoundException
in multithreaded Java applications requires a profound understanding of exception management, concurrency, and resource access. Implementing proper synchronization, utilizing higher-level I/O libraries, and employing thread pooling can significantly improve performance and reliability.
By mastering these concepts, your applications will not only be robust against exceptions but also efficient in managing resources. Always be prepared for unexpected outcomes, and handle each potential failure points with care.
With these strategies in your coding arsenal, you’re now well-equipped to tackle any FileNotFoundException
that comes your way! Happy coding!
Further Reading
- Java Concurrency in Practice
- Effective Java by Joshua Bloch