Common Java Serialization Mistakes and How to Fix Them
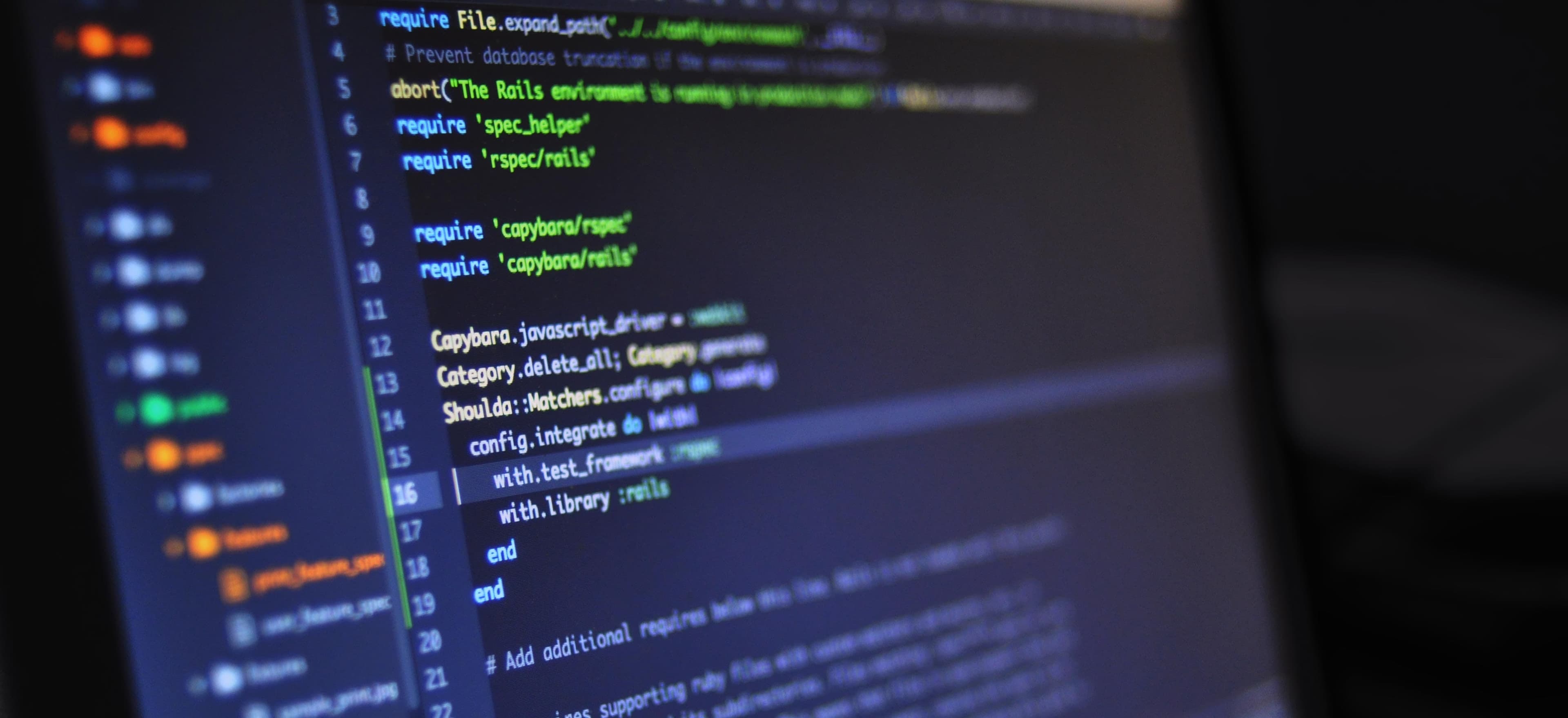
- Published on
Common Java Serialization Mistakes and How to Fix Them
Java serialization is a powerful feature that enables object persistence and transfer over a network. However, developers often encounter pitfalls during implementation. In this blog post, we will explore common serialization mistakes, their implications, and how to effectively address them. By the end, you'll gain a better understanding of best practices in Java serialization, ensuring robust and efficient object handling in your applications.
What is Serialization in Java?
Serialization in Java is the process of converting an object into a byte stream, making it easy to save it to a file or send it over a network. Conversely, deserialization is the process of converting a byte stream back into a Java object. The Serializable
interface plays a crucial role in this process.
Example of a Simple Serializable Class
import java.io.Serializable;
public class User implements Serializable {
private String username;
private String password;
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and Setters
}
In this example, the User
class implements the Serializable
interface, allowing its instances to be serialized.
Importance of the serialVersionUID
One of the most common mistakes in Java serialization is neglecting to define the serialVersionUID
. This unique identifier helps maintain version control of your serialized classes. If no serialVersionUID
is defined, Java generates one automatically, which can lead to InvalidClassException
during deserialization when the class structure changes.
Solution
Define a serialVersionUID
in your class to avoid versioning issues:
private static final long serialVersionUID = 1L;
The Pitfall of Transient Fields
Another common mistake is not using the transient
keyword for non-serializable fields. If you try to serialize an object with non-serializable member variables, it will throw a NotSerializableException
.
Example
import java.io.Serializable;
public class User implements Serializable {
private String username;
private transient String password; // Password should not be serialized
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and Setters
}
Explanation
In the example above, the password
field is marked as transient
. It ensures that sensitive information is not serialized, enhancing security.
Default Serialization and Customization
Java provides a default mechanism for serialization, but there are cases where you may desire more control. Relying solely on default serialization can lead to inefficiencies and errors, particularly with complex objects.
Customized Serialization Approach
You can customize the serialization process by implementing the writeObject
and readObject
methods.
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
out.defaultWriteObject(); // Serialize default fields
// Custom serialization logic for complex objects
}
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject(); // Deserialize default fields
// Custom deserialization logic for complex objects
}
In this example, writeObject
and readObject
modify the default behavior. By maintaining control, you can ensure data integrity during the serialization process.
Serializable Collections
Collections in Java, such as ArrayList
, are inherently serializable. However, the individual elements contained within the collection must also be serializable. A common mistake is to forget this, leading to unexpected exceptions.
Solution
Always ensure that all objects in a collection implement Serializable
. Here’s an example of a serializable list of users:
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
public class UserGroup implements Serializable {
private List<User> users = new ArrayList<>();
public void addUser(User user) {
users.add(user);
}
// More methods
}
The Impact of Circular References
Circular references pose another challenge during serialization. If two objects reference one another, it may result in a StackOverflowError
. Java's serialization mechanics will handle circular references, but this can still impact performance.
Solution
To mitigate this risk, carefully design your object relationships or utilize a third-party library (like Jackson) to handle serialization more efficiently.
Deserialization Vulnerabilities
Deserialization can introduce significant security risks, such as code injection attacks. Attackers may manipulate byte streams to exploit deserialization processes in your application.
Preventive Measures
- Use Whitelisting: Only allow known classes to be deserialized.
- Implement Custom Deserialization: Validate data before object creation.
- Adopt Libraries: Consider using safer libraries like Kryo or Protobuf for data persistence.
In Conclusion, Here is What Matters
Serialization in Java is essential, yet developers must navigate it carefully to avoid common mistakes. By implementing best practices—such as defining serialVersionUID
, utilizing the transient
keyword, customizing serialization methods, ensuring valid collections, and guarding against security vulnerabilities—you'll enhance your application's stability and security.
For more comprehensive insights, you can refer to Oracle's documentation on Serialization.
References
By avoiding common pitfalls and implementing these strategies, you can navigate the intricacies of Java serialization confidently. Happy coding!
Checkout our other articles