Fixing ClassNotFoundException in Java Reflection: A Guide
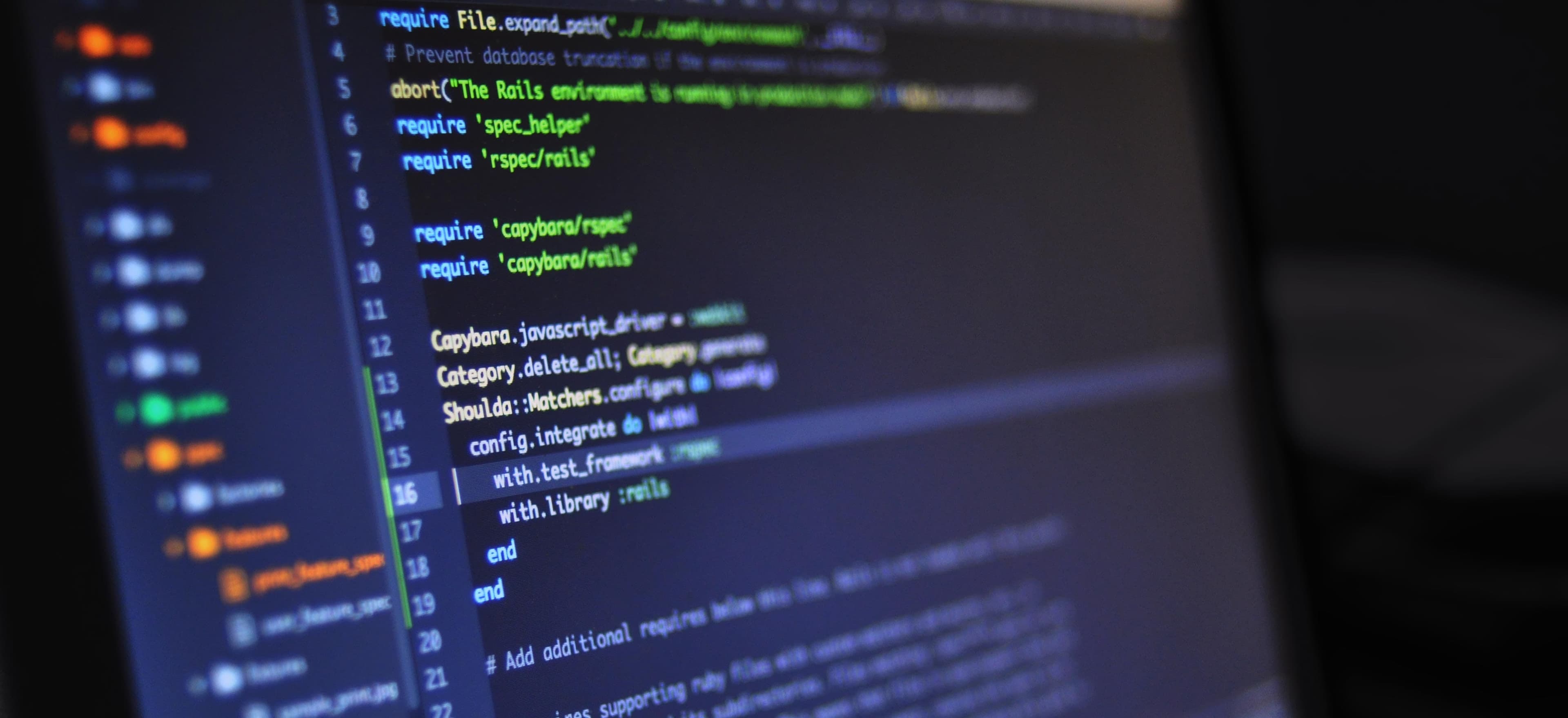
- Published on
Fixing ClassNotFoundException in Java Reflection: A Guide
Java Reflection is a powerful feature that enables developers to analyze and manipulate classes at runtime. However, when working with reflection, you might encounter a ClassNotFoundException
. This error can be frustrating, especially if you're unaware of the underlying causes. In this post, we'll explore the reasons for ClassNotFoundException
in Java Reflection, provide strategies to resolve it, and present best practices for using reflection in Java.
What is ClassNotFoundException?
Before diving into solutions, let’s understand what ClassNotFoundException
is. It occurs when an application tries to load a class at runtime using its string name but cannot find it in the classpath. This can happen due to several reasons, including:
- The class not being defined.
- Incorrect class name or package path.
- Class not present in the runtime classpath due to various reasons (like missing JAR files).
Understanding Java Reflection
Java Reflection allows you to inspect classes, interfaces, fields, and methods at runtime, regardless of their access modifiers. For example, you can dynamically create objects, change field values, and invoke methods.
Here’s a simple example of using reflection to create an instance of a class:
public class Demo {
private String message;
public Demo(String message) {
this.message = message;
}
public void printMessage() {
System.out.println(message);
}
}
Using reflection to create an instance of the Demo
class:
try {
Class<?> demoClass = Class.forName("Demo");
Constructor<?> constructor = demoClass.getConstructor(String.class);
Object demoInstance = constructor.newInstance("Hello Reflection!");
Method method = demoClass.getMethod("printMessage");
method.invoke(demoInstance);
} catch (ClassNotFoundException e) {
System.err.println("Class not found: " + e.getMessage());
} catch (Exception e) {
e.printStackTrace();
}
Why the Code Works
Class.forName("Demo")
is meant to load the class namedDemo
.getConstructor(String.class)
looks for a constructor that takes aString
parameter.newInstance("Hello Reflection!")
creates an instance ofDemo
.- Finally,
invoke
calls theprintMessage
method on that instance.
If the class name supplied to forName()
is incorrect, however, a ClassNotFoundException
will be raised.
Common Causes of ClassNotFoundException
1. Incorrect Class Name or Package Path
Ensure that you are using the correct class name and package path. Java is case-sensitive, so "demo" is not the same as "Demo". Here’s how to check:
- Verify the class path.
- Check the spelling and case sensitivity.
2. Class Not in Classpath
Ensure the class is included in the runtime environment. If you’re using an IDE like IntelliJ or Eclipse, you might need to check your project structure to ensure all dependencies are correctly referenced.
3. Missing JAR Files
If your class exists within a JAR file, make sure that the JAR file is included in your classpath. You can manually add JARs to your classpath:
java -cp .:my-library.jar MyMainClass
4. Class Loading Issues with Different ClassLoaders
Sometimes, classes are loaded by different ClassLoaders in complex applications or server environments. Here’s how to troubleshoot:
- Use
Thread.currentThread().getContextClassLoader()
to get the current class loader. - Explicitly load the class via the correct class loader, if necessary.
How to Fix ClassNotFoundException
1. Double-Check Class Names
Make sure your class is defined correctly. If you notice a discrepancy, correct it:
// Correct usage
Class<?> myClass = Class.forName("com.example.Demo");
2. Check Your IDE Configuration
If you’re using an IDE, ensure that your build and runtime configurations are set up correctly. For instance, in IntelliJ IDEA:
- Go to Project Structure.
- Ensure all required libraries are properly set under Modules.
3. Verify Classpath Settings
Ensure that the classpath is set up correctly. This can often be a pain point in Java projects. Use the -cp
option appropriately depending on how you package and run your application.
4. Handle JAR Dependencies
When working with libraries, ensure you include the necessary JARs in your build tools (like Maven or Gradle).
Example in Maven:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
</dependency>
5. Utilize Exception Handling
Always use exception handling when working with reflection, which allows you to discern which specific action caused the failure.
try {
// Reflection code here
} catch (ClassNotFoundException e) {
logError("Class not found: " + e.getMessage());
} catch (NoSuchMethodException e) {
logError("No such method: " + e.getMessage());
} catch (InstantiationException | IllegalAccessException | InvocationTargetException e) {
logError("Error creating instance: " + e.getMessage());
}
6. Testing Class Loading
To test if your class loads successfully, you can write a simple loader:
public static void testLoadClass(String className) {
try {
Class.forName(className);
System.out.println("Class " + className + " loaded successfully.");
} catch (ClassNotFoundException e) {
System.err.println("Class " + className + " not found.");
}
}
public static void main(String[] args) {
testLoadClass("com.example.Demo");
}
Best Practices for Using Reflection in Java
- Limit its Use: Use reflection judiciously. It can make debugging more challenging and degrade performance since it bypasses compile-time checks.
- Static Typing: Where possible, prefer static typing over reflection which provides better readability and maintainability.
- Performance Monitoring: Regularly monitor performance when using reflection in application-critical parts of your code.
Final Thoughts
ClassNotFoundException
can be a hindrance when working with Java Reflection, but understanding the common pitfalls and employing best practices can help you navigate this easily. Always validate class paths, be careful with names, and ensure JAR files are included.
For a deeper dive into Java Reflection, you can check out the Java Tutorials provided by Oracle.
By addressing reflection-related issues methodically, you can leverage the power of runtime class manipulation without the headaches. Happy coding!
Checkout our other articles