Optimizing Java String Intern Memory for Better Performance
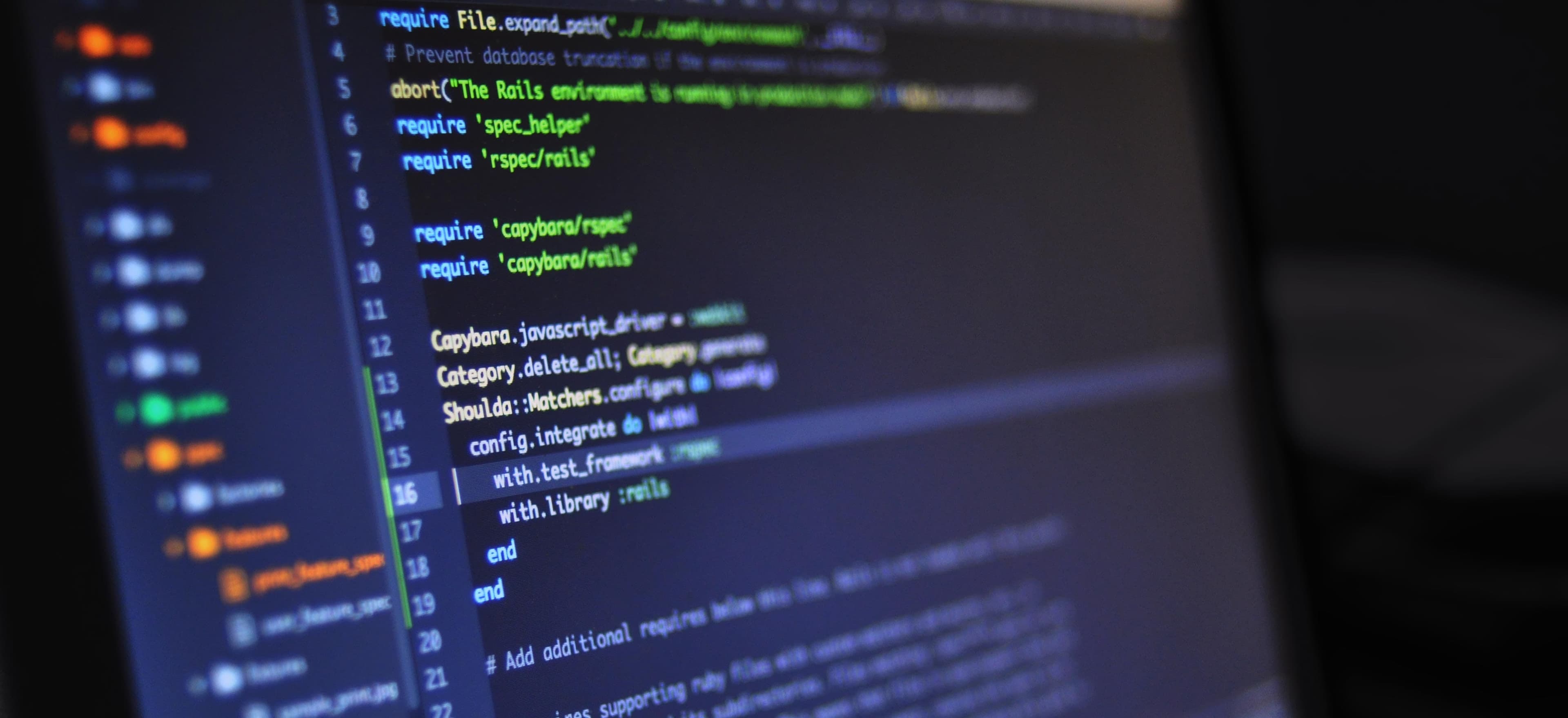
- Published on
Optimizing Java String Intern Memory for Better Performance
Java developers often face challenges related to memory management, especially when dealing with strings. One effective way to enhance performance and manage memory usage is through String interning. This blog post delves into the concept of string interning in Java, why it matters, and how it can be optimized for better performance.
Understanding String Interning in Java
Before diving into the optimization techniques, let's clarify what string interning is. In Java, strings are immutable objects, which means once a string is created, it cannot be altered. The String intern method helps manage memory more efficiently. It ensures that all identical string literals in the application point to a single instance in the String pool, thereby saving memory and improving performance.
The String Pool
The String pool is a special memory region within the Java heap where Java stores string literals. When a string literal is created, the JVM checks the pool to see if a string with the same content already exists:
- If it does, the reference to the existing instance is returned.
- If not, a new string object is created and added to the pool.
String str1 = "Hello";
String str2 = "Hello";
System.out.println(str1 == str2); // Outputs: true
In the code above, since both str1
and str2
reference the same string literal in the String pool, they point to the same object in memory.
Why Optimize String Interning?
Even though string interning helps save memory, improper use can lead to several issues, such as:
- Memory Exhaustion: Excessive use of string interning for dynamic strings (non-literal strings) can lead to increased memory consumption.
- Performance Degradation: If string literals are interred unnecessarily, it can degrade performance due to the overhead of checking the pool.
- Garbage Collection Concerns: Interned strings, once added to the pool, remain in memory until the class loader is disposed of, which can lead to memory leaks in long-running applications.
Given these potential issues, optimizing string intern usage becomes crucial.
Techniques for Optimizing String Interning
1. Use Interning Judiciously
Interning should be reserved for strings that are reused frequently. Before interning a string, evaluate its potential for reuse.
String dynamicString = new String("Hello World!").intern();
In this example, while the string is interned, excessive dynamic strings should be avoided unless they will be reused.
2. Leverage StringBuilder
For string manipulation, utilize StringBuilder
instead of repeatedly creating new string objects. StringBuilder
generates string objects without permanently consuming them in memory.
StringBuilder builder = new StringBuilder();
for (int i = 0; i < 10; i++) {
builder.append("String ").append(i).append(" ");
}
String finalString = builder.toString(); // Optimized for performance
In this snippet, StringBuilder
efficiently appends strings without creating unnecessary intermediate string objects.
3. Precompute Common Strings
If you have a set of common strings that will be used frequently, precompute and intern them at the start of your application.
public class CommonStrings {
public static final String HELLO = "Hello".intern();
public static final String WORLD = "World".intern();
}
By doing so, you reduce the runtime overhead of intern calls during the application's lifecycle.
4. Benchmark Performance
Always benchmark the performance of string interning in your application. Using a profiling tool can help identify memory and performance bottlenecks associated with string use:
public static void main(String[] args) {
long startTime = System.nanoTime();
for (int i = 0; i < 10000; i++) {
String str = "Hello" + i; // Dynamically created
str.intern(); // Measure the impact of intern
}
long endTime = System.nanoTime();
System.out.println("Time taken: " + (endTime - startTime) + " ns");
}
This code snippet can help ascertain whether interning is beneficial or detrimental in your specific context.
5. Avoid Interning Large Strings
Interning large strings can significantly increase memory consumption. It's best to avoid interning strings that occupy a substantial amount of memory or are not expected to have multiple references.
6. Clean Up the String Pool
In some cases, cleaning the string pool explicitly may help. While this is generally not feasible due to limitations (e.g. accessing interned strings is not straightforward), proper management of application contexts and class loaders can help prevent excessive buildup of interned strings.
7. Use Java 9 and Beyond Features
From Java 9 onwards, you can utilize String::concat
to create new strings more efficiently. The concat()
method is optimized to utilize StringBuilder
internally, and the JVM can optimize the allocation.
String greeting = "Hello".concat(" World");
This approach gives more control over how string instances are created, reducing the need to rely on interning.
A Final Look
While string interning is a powerful mechanism to improve memory efficiency and performance in Java applications, it requires careful consideration and optimization. By using interning intelligently, leveraging StringBuilder
, precomputing common strings, and avoiding unnecessary interning of large strings, developers can achieve significant optimization.
Incorporating these strategies will lead to more efficient memory usage and improved application performance. For further reading on Java memory management, consider checking the Java Documentation which provides in-depth insights into string handling and memory allocation.
By keeping interning in check, we not only foster better performance but also create a more maintainable codebase, laying the groundwork for efficient application development. Happy coding!
Checkout our other articles