Conquering StackOverflowError in Your toString() Methods
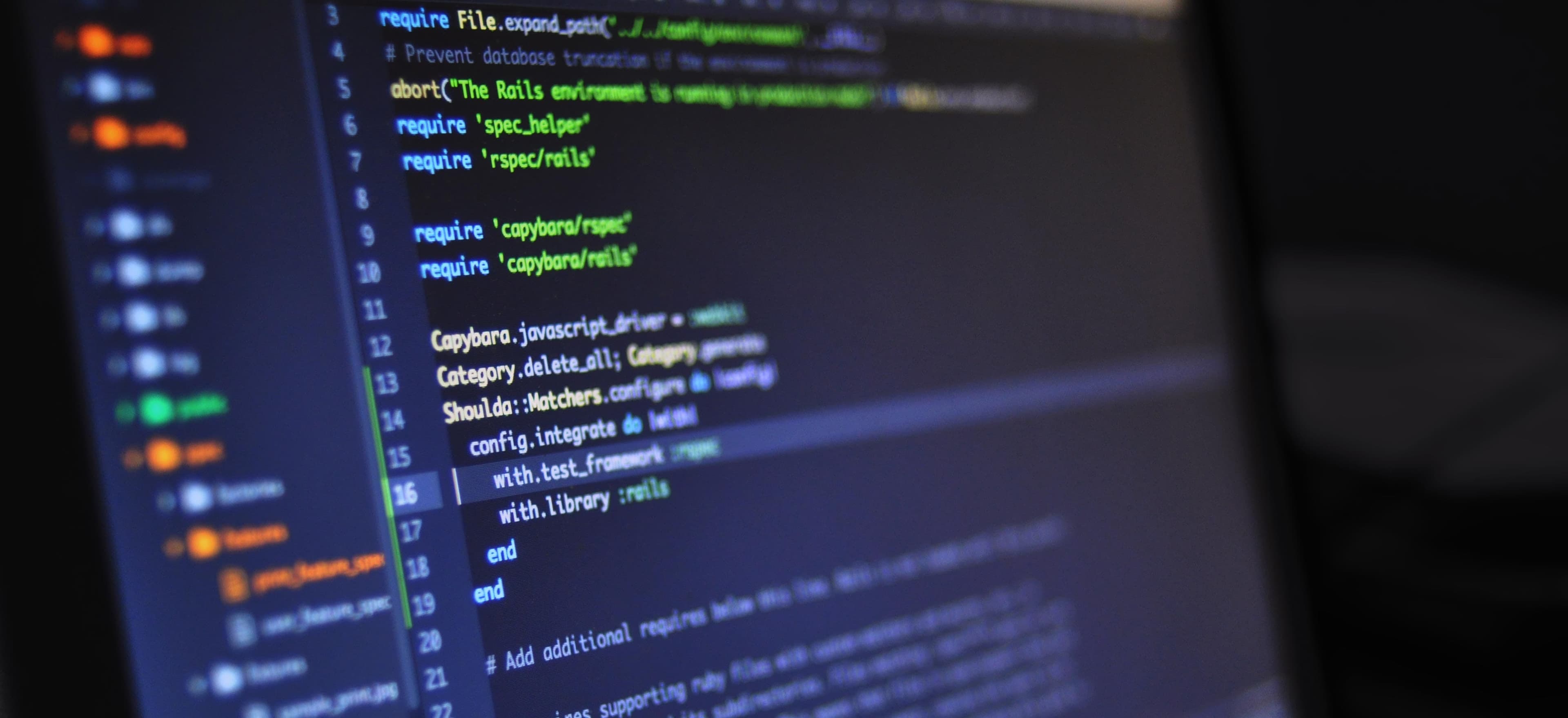
- Published on
Conquering StackOverflowError in Your toString() Methods
When developing in Java, one common pitfall that developers encounter is the dreaded StackOverflowError
. This error typically arises from excessive recursion, and it often rears its head when implementing the toString()
method in classes, especially those with bidirectional relationships. This blog post aims to dive deep into understanding and avoiding StackOverflowError
when crafting effective toString()
methods.
Understanding StackOverflowError
Before we delve into solutions for preventing StackOverflowError
, let's briefly discuss what it is. Java utilizes a call stack to keep track of method calls in a program. Each time a method is called, it is pushed onto the stack. If a method calls itself recursively without a proper base case to terminate the calls, it can lead to the stack exceeding its allocated limit, resulting in a StackOverflowError
.
Example of a StackOverflowError
Consider the following simplistic implementation of a class that defines a person and attempts to print the person’s details:
class Person {
String name;
Person friend;
// Constructor
public Person(String name, Person friend) {
this.name = name;
this.friend = friend;
}
@Override
public String toString() {
return "Person{name='" + name + "', friend=" + friend + '}';
}
}
public class Main {
public static void main(String[] args) {
Person john = new Person("John", null);
Person jane = new Person("Jane", john);
john.friend = jane; // Creates a circular reference
System.out.println(john);
}
}
In this example, when we attempt to print john
, it will lead to an infinite loop as both john
and jane
reference each other. Consequently, Java will throw a StackOverflowError
.
Best Practices for Implementing toString()
1. Avoid Circular References
To ensure you don't end up in a loop, always review object references. If your objects can reference each other (for instance, two objects referring to each other), implement your toString()
methods carefully.
Example of a Safe Implementation
Here’s how we can modify the toString()
method to avoid infinite loops:
import java.util.HashSet;
import java.util.Set;
class Person {
String name;
Person friend;
// Constructor
public Person(String name, Person friend) {
this.name = name;
this.friend = friend;
}
@Override
public String toString() {
return toString(new HashSet<>());
}
private String toString(Set<Person> visited) {
if (visited.contains(this)) {
return "Person{name='" + name + "', friend=...}";
}
visited.add(this); // Mark this object as visited
return "Person{name='" + name + "', friend=" + (friend != null ? friend.toString(visited) : "null") + '}';
}
}
Commentary
In this implementation:
- We use a
Set<Person>
to keep track of the objects we have visited during the traversal. - Before attempting to print the friend's details, we check if that person has already been visited.
- If they have, we substitute the string with "friend=...".
This prevents the recursive calls from infinitely looping.
2. Limit Recursion Depth
Limiting the depth of recursion can prevent very deep structures from triggering a StackOverflowError
.
Example with Depth Limit
class Person {
String name;
Person friend;
private static final int MAX_DEPTH = 3;
// Constructor
public Person(String name, Person friend) {
this.name = name;
this.friend = friend;
}
@Override
public String toString() {
return toString(0);
}
private String toString(int depth) {
if (depth >= MAX_DEPTH) {
return "Person{name='" + name + "', friend=...}";
}
return "Person{name='" + name + "', friend=" + (friend != null ? friend.toString(depth + 1) : "null") + '}';
}
}
Commentary
In this example:
- We maintain a depth variable that increments with each recursion.
- If we exceed the defined
MAX_DEPTH
, we use a placeholder instead of navigating further.
3. Use JSON Libraries
Another effective way to circumvent potential StackOverflowError
is to use libraries like Gson or Jackson that automatically manage circular references well.
Example using Gson
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
class Person {
String name;
Person friend;
// Constructor
public Person(String name, Person friend) {
this.name = name;
this.friend = friend;
}
}
public class Main {
public static void main(String[] args) {
Gson gson = new GsonBuilder().setExclusionStrategies(new GsonExclusionStrategy()).create();
Person john = new Person("John", null);
Person jane = new Person("Jane", john);
john.friend = jane; // Creates a circular reference
System.out.println(gson.toJson(john));
}
private static class GsonExclusionStrategy implements com.google.gson.ExclusionStrategy {
@Override
public boolean shouldSkipField(com.google.gson.FieldAttributes f) {
return f.getName().equals("friend"); // Customize as needed
}
@Override
public boolean shouldSkipClass(Class<?> clazz) {
return false;
}
}
}
Commentary
In this example with Gson:
- We leverage a JSON serialization library to handle object transformation.
- By creating a custom exclusion strategy, we can control which fields to skip, thereby preventing infinite loops.
My Closing Thoughts on the Matter
While the toString()
method is a fundamental aspect of Java programming, it's vital to implement it with caution, especially in the presence of circular references. By avoiding circular references, limiting recursion depth, or utilizing libraries like Gson for serialization, you can effectively dodge the stack overflow pitfall.
Furthermore, remember that writing clean and maintainable code is essential. Always consider the implications of your method implementations and strive for clear, concise, and safe representations of your objects.
Additional Resources
- Java Documentation on Exceptions
- Gson GitHub Repository
- Best practices for implementing toString() in Java
By mastering these techniques, you will be well-equipped to write robust toString()
methods that won’t crash your application due to StackOverflowError
. Happy coding!
Checkout our other articles