Why Synchronized Collections Can Cause Performance Bottlenecks
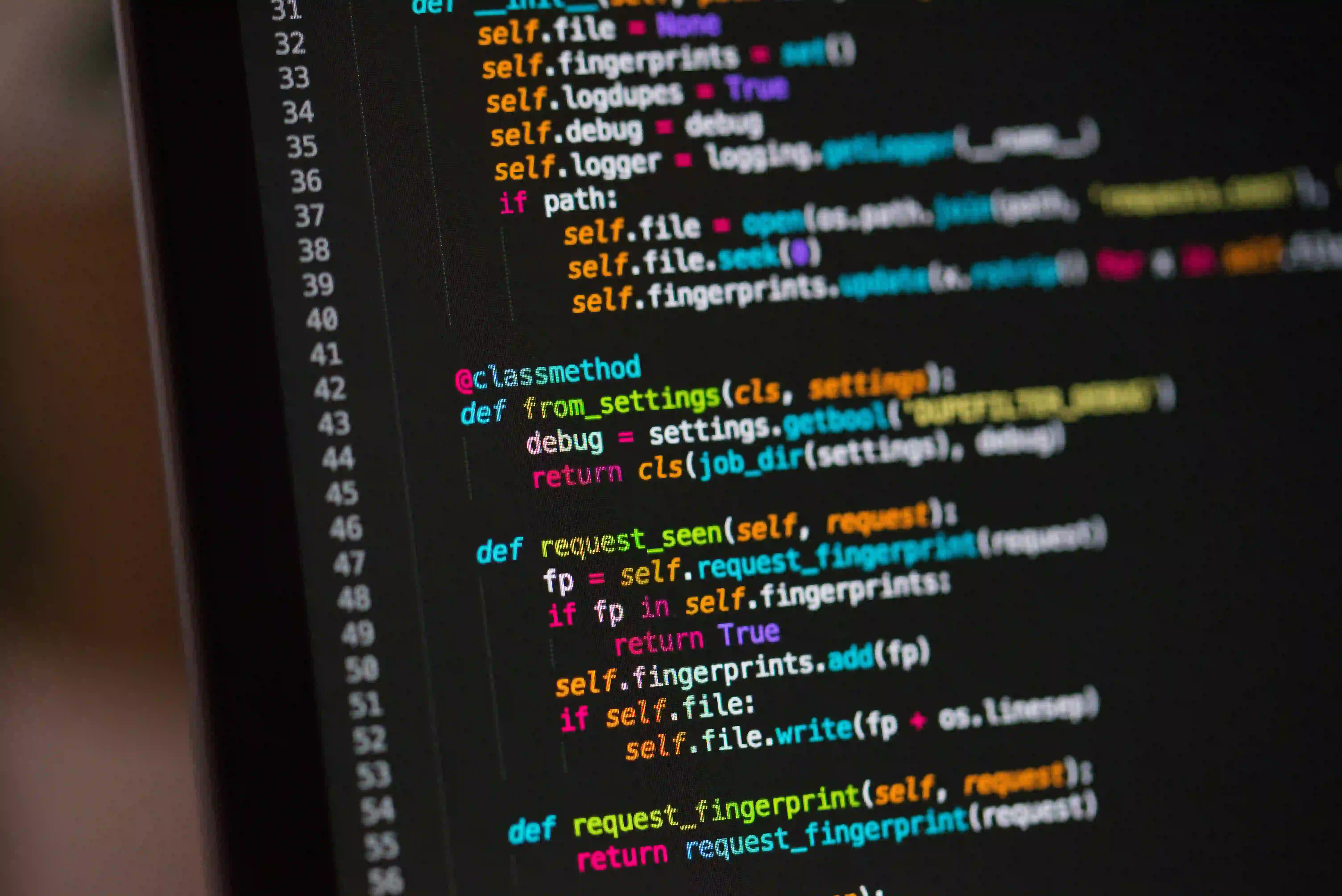
Why Synchronized Collections Can Cause Performance Bottlenecks
In the realm of Java programming, managing concurrent access to collections is a common challenge. Java offers synchronized collections to facilitate thread-safe operations. However, using these collections can result in performance bottlenecks, especially in high-throughput systems. This blog post delves into why synchronized collections may lead to significant slowdowns and how you can address these challenges.
Understanding Synchronized Collections
Java provides several synchronized collections, such as Vector
, Hashtable
, and those returned by Collections.synchronizedList()
, Collections.synchronizedMap()
, etc. These collections automatically synchronize their methods to ensure that only one thread can access the collection at a time.
Here’s a visual of what happens in a synchronized collection:
import java.util.Collections;
import java.util.List;
import java.util.ArrayList;
public class SynchronizedCollectionExample {
public static void main(String[] args) {
List<String> synchronizedList = Collections.synchronizedList(new ArrayList<>());
// Adding elements to synchronized list
synchronizedList.add("Java");
synchronizedList.add("Python");
synchronizedList.add("C++");
}
}
Why Synchronized Collections?
The primary reason for using synchronized collections is to maintain data integrity when multiple threads access the same collection. If thread A is writing to a collection while thread B reads from it, it may lead to inconsistent data states. By synchronizing collections, Java programmers can avoid these concurrency issues.
However, relying solely on synchronized collections poses a risk to application performance.
The Cost of Synchronization
-
Lock Contention
When multiple threads attempt to access synchronized collections, they are blocked if another thread is already accessing the collection. This contention for the lock can cause a significant delay in processing time.
For instance, imagine a scenario where ten threads are trying to read from a synchronized collection. If one thread is holding the lock for writing, all other threads must wait. This can lead to performance degradation during peak loads or in scenarios where you have a lot of concurrent access.
public class LockContentionExample {
private static final List<String> synchronizedList = Collections.synchronizedList(new ArrayList<>());
public static void main(String[] args) throws InterruptedException {
// Simulating multiple threads trying to access the synchronized list
for (int i = 0; i < 10; i++) {
Thread thread = new Thread(() -> {
synchronized (synchronizedList) { // Getting lock on collection
for (int j = 0; j < 1000; j++) {
synchronizedList.add("Element " + j);
}
}
});
thread.start();
thread.join(); // Ensure one thread completes before starting another
}
}
}
-
Granular Control
The tight coupling provided by synchronized collections means that they generally can’t differentiate between read and write operations. This lack of granularity means that even when data retrieval (read operations) could safely occur without conflict, threads are still blocked, leading to underutilization of available system resources.
Performance Bottlenecks in Real-World Applications
The issues with synchronized collections become more apparent as the complexity of an application increases. Consider the following:
1. High Transaction Throughput
In applications like web services or APIs that require high transaction throughput, the performance impact of synchronized collections can be severe. Here’s an example:
- Scenario: A web service handles a large volume of requests, each involving interactions with a synchronized list.
- Impact: Increased latency and bottlenecks as multiple requests align for access to the same collection.
To address this, consider implementing more sophisticated concurrent mechanisms such as ConcurrentHashMap
, which allows for thread-safe operations without blocking entire collections.
2. Increased Memory Usage
Synchronized collections often add overhead in terms of memory management. Each lock introduces a unique identifier that increases the amount of memory needed for synchronization. This can lead to excessive garbage collection processes and increased memory footprint.
Alternatives to Synchronized Collections
If synchronized collections introduce too much overhead, consider the following alternatives:
1. Concurrent Collections
Java offers concurrent collections, such as ConcurrentHashMap
and CopyOnWriteArrayList
, designed specifically to handle concurrent read/write operations. They provide improved performance over synchronized collections by allowing finer-grained locking:
Example: Using ConcurrentHashMap
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentCollectionExample {
public static void main(String[] args) {
ConcurrentHashMap<String, String> concurrentMap = new ConcurrentHashMap<>();
// Using putIfAbsent method for thread-safe insertion
concurrentMap.putIfAbsent("1", "Java");
concurrentMap.putIfAbsent("2", "Python");
concurrentMap.putIfAbsent("3", "C++");
}
}
2. Custom Synchronization
Develop your custom synchronization mechanisms. This approach offers maximum flexibility but requires careful implementation to avoid race conditions and other concurrency issues.
For example, you might want to use ReentrantLock
for explicit locking:
import java.util.*;
import java.util.concurrent.locks.ReentrantLock;
public class CustomSynchronizationExample {
private static final ReentrantLock lock = new ReentrantLock();
private static final List<String> list = new ArrayList<>();
public static void main(String[] args) {
// Custom add method with locking
try {
lock.lock();
list.add("Java");
list.add("Python");
} finally {
lock.unlock();
}
}
}
Final Considerations
While synchronized collections have their place in Java, they can produce performance bottlenecks that hinder application efficiency under concurrent loads. Understanding the implications of using synchronized collections is vital for any Java developer.
By choosing the correct data structures, like concurrent collections or implementing custom synchronization strategies, you can enhance your applications' performance significantly.
Thus, remember that in the world of concurrent programming, performance and safety can go hand in hand – if you make the right choices.
For further reading, check out the official Java documentation on concurrent collections or explore Java's threading model.
By implementing the insights from this post, you’ll not only understand the pitfalls of synchronized collections but also equip yourself with strategies to avoid them and optimize your applications effectively.