Mastering Static Fields: Preventing Java Memory Leaks
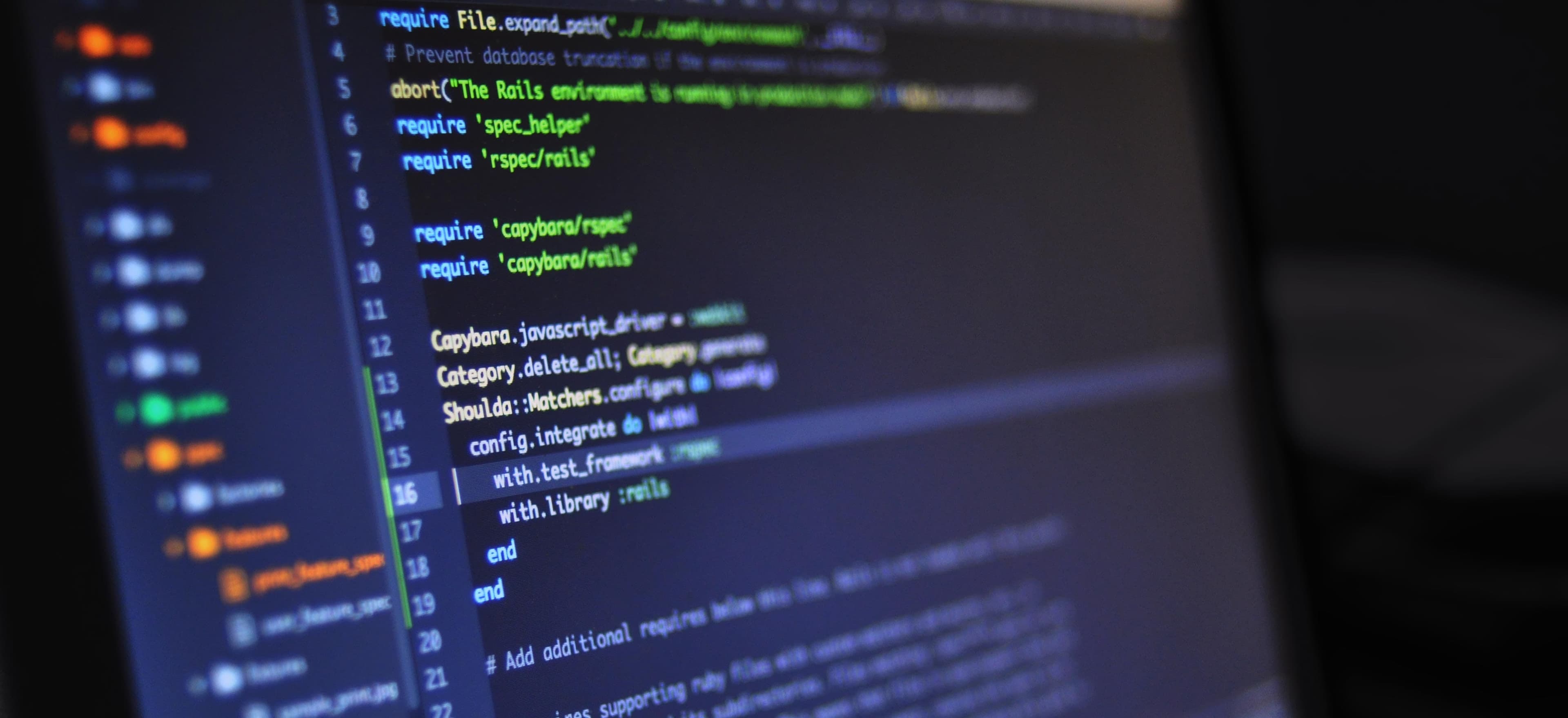
- Published on
Mastering Static Fields: Preventing Java Memory Leaks
In the world of Java programming, understanding memory management is pivotal. One of the more challenging aspects that developers must contend with is managing static fields. As harmless as they may seem, static fields can lead to serious memory leaks if mismanaged. This blog post aims to equip you with the knowledge to master static fields and prevent related memory leaks in your Java applications.
What are Static Fields?
Static fields are variables that belong to a class rather than a specific instance of that class. This means that all instances of the class share the same value for this field. Java uses the static
keyword to declare these fields, and they are initialized only once during the class's lifecycle.
Why Use Static Fields?
-
Memory Efficiency: Since static fields are shared among all instances of a class, they provide a convenient way to conserve memory.
-
Global Access: They can be accessed directly through the class name, making them ideal for constants or utility functions.
-
State Management: Static fields can manage state across instances, which is useful in certain design patterns like Singleton.
Example
class Configuration {
static String appName = "MyApplication";
public static String getAppName() {
return appName;
}
}
In this example, appName
is shared among all instances of Configuration
. When any instance gets appName
, they access the same static field.
Understanding Memory Leaks
Memory leaks occur when objects are not released after they are no longer needed, leading to an increase in memory usage over time. In Java, the Garbage Collector (GC) automatically deallocates memory for objects that are no longer in use. However, if static fields hold references to objects that are not released, the GC cannot reclaim that memory.
Common Sources of Memory Leaks with Static Fields
-
Unintentional Circular References: If a static field holds a reference to a non-static singleton, and it in turn holds a reference back to the static field, both will prevent garbage collection.
-
Event Listeners: If a static field is used to store listeners, and these listeners hold references to UI components, the components won't be eligible for garbage collection.
-
Static Caches: Caches that hold onto objects indefinitely can lead to memory leaks if they aren't cleared.
Example of a Memory Leak
class EventListener {
private static List<Listener> listeners = new ArrayList<>();
public static void addListener(Listener listener) {
listeners.add(listener);
}
}
// Usage
EventListener.addListener(new Listener());
In the above example, if your application keeps adding new listeners without removing them, the list will grow indefinitely, occupying memory that can never be reclaimed.
Strategies to Prevent Memory Leaks
Here are some strategies to efficiently use static fields and avoid memory leaks:
1. Use Weak References
Using WeakReference
allows the garbage collector to reclaim the referenced object even if it is still available, alleviating memory leak concerns.
import java.lang.ref.WeakReference;
class Cache {
static Map<String, WeakReference<Object>> cache = new HashMap<>();
public static void put(String key, Object value) {
cache.put(key, new WeakReference<>(value));
}
public static Object get(String key) {
WeakReference<Object> ref = cache.get(key);
return (ref != null) ? ref.get() : null;
}
}
In this code, putting an object into the cache retains a weak reference to it. If another part of your program doesn't hold a strong reference to that object, it will be eligible for garbage collection.
2. Clear Static Fields
Whenever you are done using static fields, explicitly clear them to release resources.
class Configuration {
private static List<String> cache = new ArrayList<>();
public static void addConfig(String config) {
cache.add(config);
}
public static void clearCache() {
cache.clear();
}
}
When clearCache()
is called, you explicitly remove the references held in the cache
list, allowing for potential garbage collection.
3. Utilize WeakHashMap for Caching
If you're caching objects, consider using WeakHashMap
. This will allow the cached objects to be garbage-collected when they're no longer in use.
import java.util.WeakHashMap;
class Cache {
private static WeakHashMap<String, Object> cache = new WeakHashMap<>();
public static void put(String key, Object value) {
cache.put(key, value);
}
// Additional methods...
}
WeakHashMap
automatically removes entries when the keys are no longer referenced elsewhere, which helps in managing memory efficiently.
4. Use a Lifecycle Management System
Design your application to manage resources effectively, particularly when using static fields. Setting up a lifecycle management system that explicitly cleans up resources can help in preventing memory leaks. For example, you could implement a method to clean up or reset static fields when your application context is closing.
Best Practices for Using Static Fields
-
Resist overusing static fields: Prefer instance fields when appropriate, as they are tied to a particular object's lifecycle.
-
Limit the scope: Keep them as private as possible to avoid unintended references.
-
Document your static fields: Make it clear for your future self and your teammates what purpose they serve and how they should be used.
-
Monitor memory usage: Use profiling tools such as VisualVM or Java Mission Control to keep track of memory allocation and identify potential leaks.
Closing the Chapter
Static fields can be a powerful tool in Java programming. However, with great power comes great responsibility. Mismanagement can lead to memory leaks and drain application performance. By understanding static fields' capabilities, knowing common pitfalls, and employing effective strategies, you can prevent memory leaks while harnessing the benefits of static fields in your applications.
For deeper insights into managing memory in Java, you may refer to Java Memory Management Overview and explore the intricacies of the Garbage Collection process.
The mastery of static fields is certainly achievable. Equip yourself with knowledge, follow the best practices, and your Java applications will run as efficiently as ever. Happy coding!