Mastering Java Multithreading: Beat Synchronization Woes!
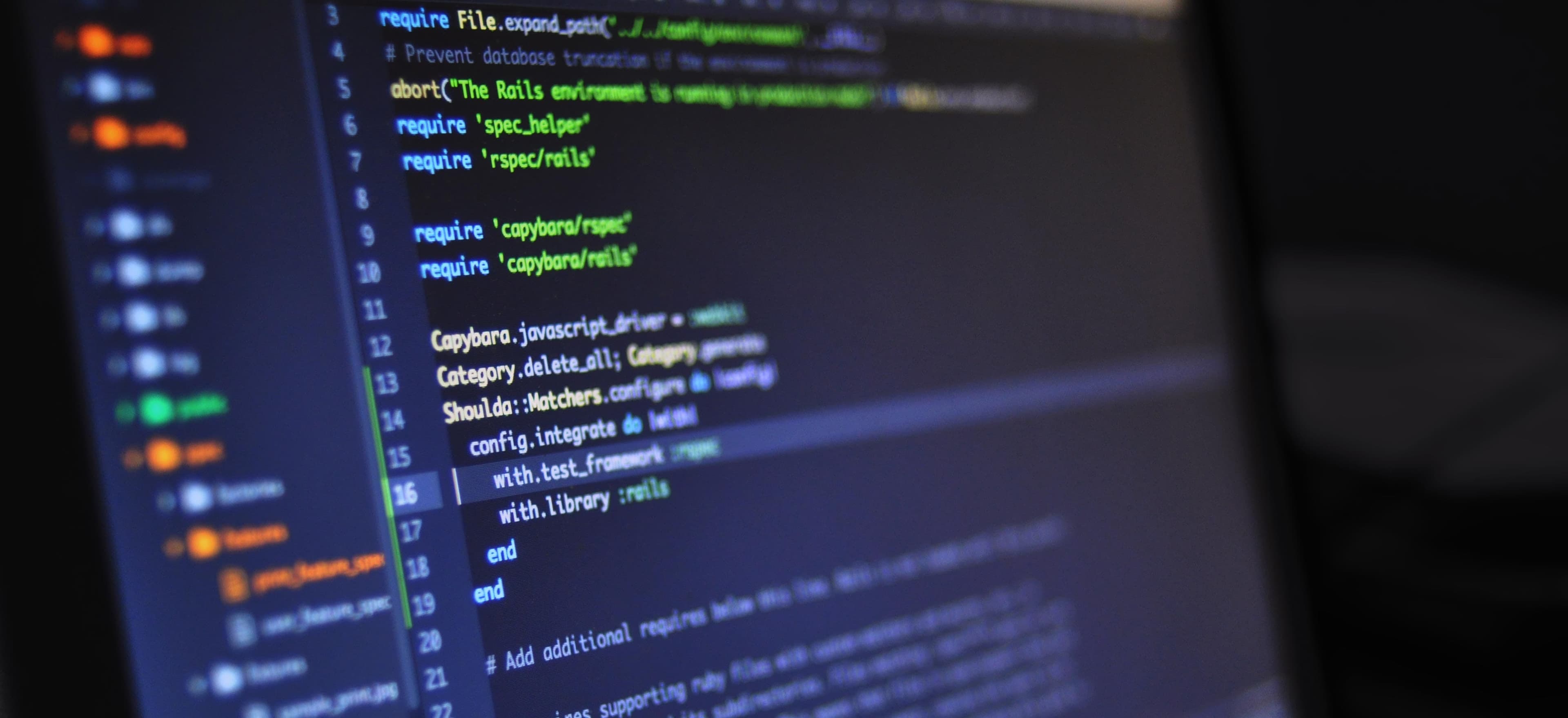
- Published on
Mastering Java Multithreading: Beat Synchronization Woes!
Java has been a cornerstone in programming languages since its inception in the mid-90s. One of its most powerful features is multithreading, which allows multiple threads to run concurrently, improving application performance and responsiveness. However, with great power comes great responsibility—synchronization issues can significantly complicate the developer's life. In this blog post, we will delve into Java multithreading, discuss synchronization, and provide code snippets that illustrate how to handle threading effectively.
Understanding Java Multithreading
Before diving into the complexities of synchronization, let's briefly explore what multithreading is and why it's essential.
Multithreading enables a Java program to execute multiple threads concurrently. In Java, each thread can be thought of as a lightweight process within the application, sharing the same memory space but running independently. This allows developers to make efficient use of CPU resources.
The Need for Synchronization
While the benefits of multithreading are evident, it introduces challenges, primarily due to shared resources. When multiple threads modify a shared resource without proper control, it can lead to inconsistent data states, a situation known as a race condition. This is where synchronization comes into play. Synchronization ensures that only one thread can access the shared resource at a time, thereby preserving data integrity.
Implementing Synchronization in Java
Java provides several mechanisms for handling thread synchronization. Let's explore these mechanisms with code examples.
1. Using the synchronized Keyword
The simplest way to control access to a shared resource is by using the synchronized
keyword. By declaring a method as synchronized, we ensure that only one thread can execute it at any time.
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this example, the increment
and getCount
methods are marked as synchronized. When one thread calls increment
, any other thread trying to call either of these methods will be blocked until the first thread completes its operation. This ensures that the count
remains consistent.
2. Synchronized Blocks
While the synchronized
keyword is straightforward, it can lead to performance issues, particularly if the synchronized methods are long-running. A more refined approach is to use synchronized blocks. This allows more granular control over the synchronization.
class SynchronizedBlockExample {
private int count = 0;
public void increment() {
synchronized (this) {
count++;
}
}
public int getCount() {
return count;
}
}
Here, we only synchronize the critical section—the increment operation—rather than the entire method. This reduces wait times for other threads, leading to better performance, especially in high-contention scenarios.
Thread Communication
In some cases, synchronization alone is not enough—threads may need to communicate with each other, particularly in producer-consumer scenarios. Java provides wait()
, notify()
, and notifyAll()
methods for this purpose.
class SharedResource {
private int count = 0;
public synchronized void produce() throws InterruptedException {
while (count >= 1) {
wait(); // Wait if resource is full
}
count++;
notifyAll(); // Notify waiting threads
}
public synchronized void consume() throws InterruptedException {
while (count <= 0) {
wait(); // Wait if resource is empty
}
count--;
notifyAll(); // Notify waiting threads
}
}
In this example, the produce
method waits if the count
reaches the maximum limit, while consume
waits if the count
is at zero. After modifying the count, both methods call notifyAll()
, which wakes up all waiting threads, allowing them to check the condition again.
Advanced Synchronization: Locks
While synchronized methods and blocks are sufficient for many applications, they can be limiting. Java offers a more sophisticated way to handle synchronization through the java.util.concurrent.locks
package, specifically the Lock
interface.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
class LockExample {
private int count = 0;
private final Lock lock = new ReentrantLock();
public void increment() {
lock.lock(); // Acquire the lock
try {
count++;
} finally {
lock.unlock(); // Ensure the lock is released
}
}
public int getCount() {
return count;
}
}
Using locks gives you more control over synchronization, such as the ability to try acquiring a lock and imposing timeouts. This flexibility can be essential in complex applications.
Final Considerations
Mastering Java multithreading and synchronization is a vital skill for any developer who aims to create responsive and performant applications. By understanding synchronization techniques—from using the synchronized
keyword to employing locks—you can handle shared resources safely and avoid common pitfalls such as race conditions.
To deepen your knowledge of Java multithreading, consider exploring resources like the Java Tutorials or Java Concurrency in Practice.
Implementing effective multithreading strategies will significantly improve your applications' efficiency and reliability. Happy coding!
Checkout our other articles