Mastering Java's ConcurrentModificationException: Solutions Inside
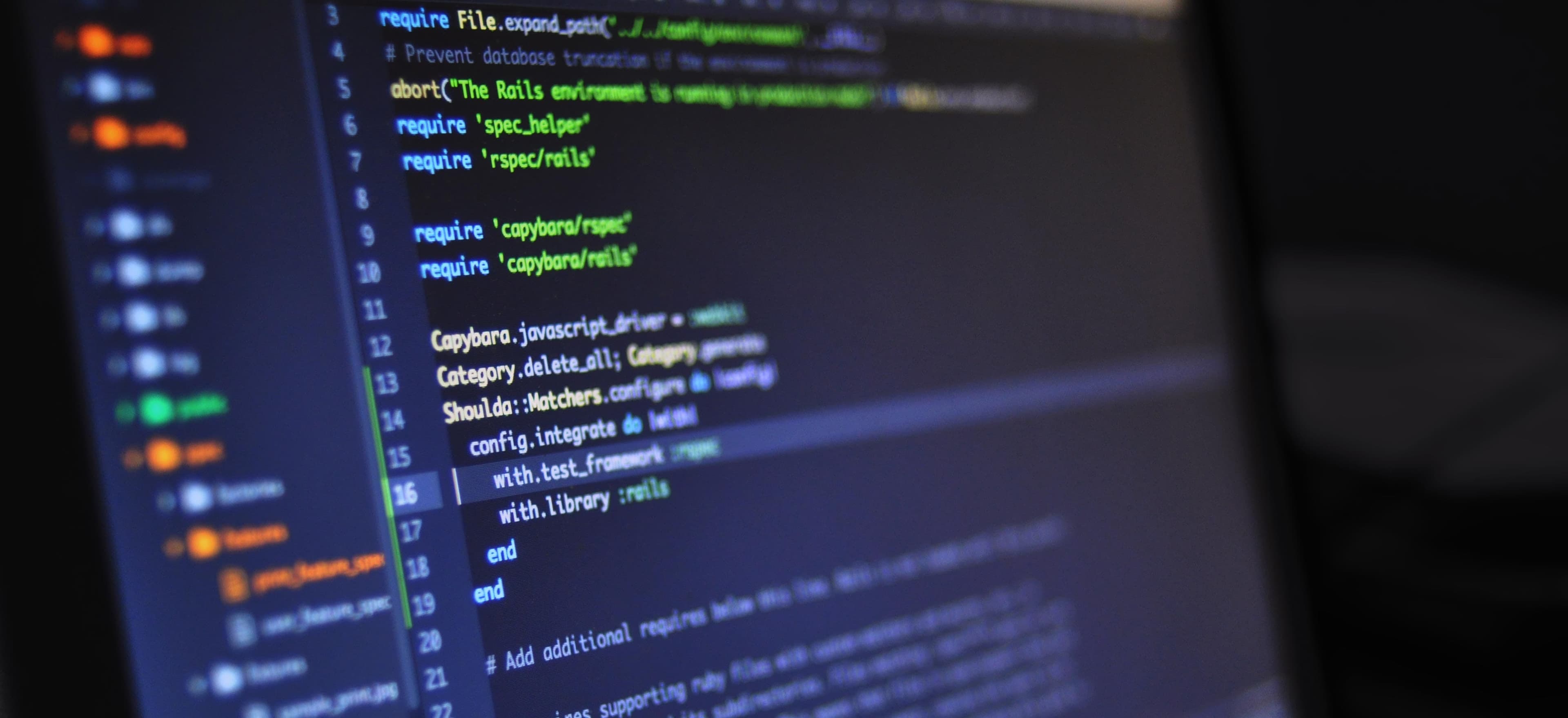
- Published on
Mastering Java's ConcurrentModificationException: Solutions Inside
Java’s ConcurrentModificationException is a common challenge faced by developers when working with collections. This exception serves as a safeguard mechanism that prevents concurrent modifications of collections during iteration. In this blog post, we’ll delve deep into what ConcurrentModificationException is, why it occurs, and most importantly, how to handle it effectively.
Understanding ConcurrentModificationException
What is ConcurrentModificationException?
In simple terms, ConcurrentModificationException
is thrown when a collection is structurally modified while it is being iterated through, except through the iterator's own remove
method. This typically happens in situations where one thread is iterating over a collection while another thread modifies it.
Why Does It Occur?
Java collections (like ArrayList
, HashMap
, etc.) provide an iterator for traversing through elements. However, if a modification is made to the collection outside of the iterator, the iterator is rendered invalid. This results in the ConcurrentModificationException
.
Here’s a straightforward example demonstrating this situation:
import java.util.ArrayList;
import java.util.List;
public class Demo {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("First");
list.add("Second");
list.add("Third");
for (String item : list) {
if (item.equals("Second")) {
list.remove(item); // This causes ConcurrentModificationException
}
}
}
}
The Output
When you run the above code, you’ll see:
Exception in thread "main" java.util.ConcurrentModificationException
This usually indicates an issue that needs addressing.
Solutions to Handle ConcurrentModificationException
1. Use Iterator’s Remove Method
The easiest way to avoid this exception when removing elements is to use the remove
method of the iterator. Here’s how:
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class SafeRemoval {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("First");
list.add("Second");
list.add("Third");
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if ("Second".equals(item)) {
iterator.remove(); // Safe removal
}
}
System.out.println(list); // Output: [First, Third]
}
}
Why This Works
By using the Iterator
's remove
method, you are removing an element in a way that allows the iterator to manage the modification count, thus preventing the exception.
2. Use Copy-On-Write Collections
For scenarios involving multi-threading, Java provides concurrent collections that are safe for concurrent access. One example is CopyOnWriteArrayList
.
Here's an example:
import java.util.List;
import java.util.concurrent.CopyOnWriteArrayList;
public class CopyOnWriteExample {
public static void main(String[] args) {
List<String> list = new CopyOnWriteArrayList<>();
list.add("First");
list.add("Second");
list.add("Third");
for (String item : list) {
if ("Second".equals(item)) {
list.remove(item); // No exception thrown
}
}
System.out.println(list); // Output: [First, Third]
}
}
Why Use Copy-On-Write?
CopyOnWriteArrayList
creates a fresh copy of the underlying array whenever a modification occurs, allowing ongoing iterations to proceed without issues. This is ideal for scenarios where reads are far more frequent than writes.
3. Synchronizing Access
If you’re traditionally iterating over a collection shared by multiple threads, you can synchronize access to the collection, effectively preventing concurrent modifications.
Here’s how:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class SynchronizedList {
public static void main(String[] args) {
List<String> list = Collections.synchronizedList(new ArrayList<>());
list.add("First");
list.add("Second");
list.add("Third");
synchronized (list) {
for (String item : list) {
if ("Second".equals(item)) {
list.remove(item); // No exception thrown during synchronization
}
}
}
System.out.println(list); // Output: [First, Third]
}
}
Why Synchronization Helps
By synchronizing the block of code that accesses the list, you ensure that no other thread can modify the list until the current thread is done iterating, thus avoiding ConcurrentModificationException
.
4. Stream API
Java 8 introduced the Stream API, a powerful tool for processing sequences of elements. With streams, you can easily filter elements without directly modifying the collection.
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("First");
list.add("Second");
list.add("Third");
// Create a new filtered list
List<String> filteredList = list.stream()
.filter(item -> !"Second".equals(item))
.collect(Collectors.toList());
System.out.println(filteredList); // Output: [First, Third]
}
}
Why Use Streams?
Using streams for processing allows you to filter and collect elements in a more functional way, avoiding direct modification of the original collection during iteration.
Best Practices to Avoid ConcurrentModificationException
- Understand Iterators: Always be mindful of the rules of iterators. Avoid modifying collections outside the provided iterator where applicable.
- Choose the Right Collection: If concurrent access is expected, consider using collections designed for such situations, like
CopyOnWriteArrayList
orConcurrentHashMap
. - Use Streams: In scenarios where you want to filter or modify collections, prefer using streams.
- Synchronize Appropriately: For shared collections accessed by multiple threads, use synchronization where necessary.
Key Takeaways
Java's ConcurrentModificationException
can catch even experienced developers off-guard. However, understanding its causes and applying the right strategies can help you avoid it effectively. Utilize iterable-safe methods such as the iterator's remove
, opt for concurrent collections, consider synchronizing access, or embrace the Stream API for cleaner solutions.
For more advanced Java concurrency topics, you may want to check out the Java Concurrency in Practice book, which provides in-depth discussions on managing concurrent modifications effectively.
By mastering the nuances of ConcurrentModificationException
, you’ll bolster your Java programming skills significantly – unlocking new heights of efficiency and productivity in your applications. Happy coding!