Overcoming Spin Loop Issues in Java NIO Selectors
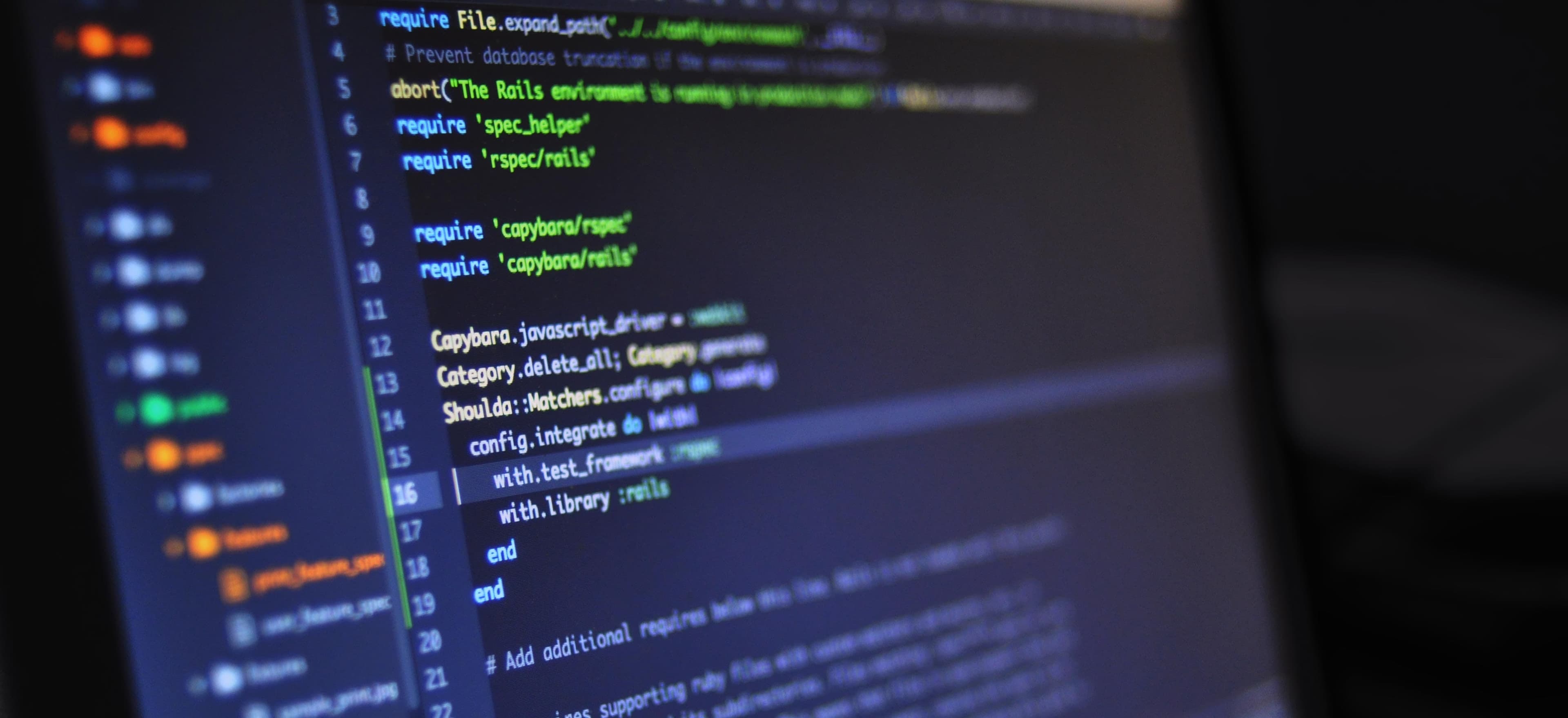
- Published on
Overcoming Spin Loop Issues in Java NIO Selectors
Java NIO (New Input/Output) provides a powerful framework for building scalable network applications. Among the pivotal components of NIO is the Selector
, which allows a single thread to manage multiple channels, thus optimizing resource use. However, the use of selectors can sometimes lead to performance issues, particularly spin loops. In this post, we will dive deep into spin loop issues associated with Java NIO selectors and explore strategies to mitigate them.
Understanding Java NIO Selectors
Before we jump into dealing with spin loops, it's essential to grasp the core functionality of NIO selectors. A Selector
enables a single thread to monitor multiple channels for events such as connection incoming requests, data readiness, etc. This capability avoids the overhead of spinning up additional threads, providing an important advantage in high-performance scenarios.
Here's a simple example to illustrate the basic usage of selectors:
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
public class BasicSelectorExample {
public static void main(String[] args) throws IOException {
Selector selector = Selector.open();
ServerSocketChannel serverChannel = ServerSocketChannel.open();
serverChannel.bind(new InetSocketAddress(9999));
serverChannel.configureBlocking(false);
serverChannel.register(selector, serverChannel.validOps());
while (true) {
// Blocking wait for events
selector.select();
// Process selected keys...
}
}
}
In the example above, we set up a non-blocking server that listens for incoming connections on port 9999. However, the infinite loop with selector.select()
might lead to spin loop issues under certain conditions.
What Are Spin Loop Issues?
A spin loop occurs when a thread continuously checks for a condition without a pause, consuming CPU cycles unnecessarily. With NIO selectors, a spin loop could manifest if the thread calling select()
is constantly triggered but finds no selectable keys.
This can negatively affect performance, especially in a multi-threaded environment where other threads should share CPU resources.
Identifying Spin Loops
Before we can overcome spin loop issues, we need to identify them. Common signs of spin loops include:
- High CPU Usage: The application may utilize excessive CPU cycles, indicating that threads are stuck in loops.
- Response Lag: End-users may notice lag in response time as resources are monopolized by the spinning thread.
To diagnose these issues, use profiling tools such as VisualVM or Java Mission Control, which can help pinpoint high CPU consumption within your application.
Overcoming Spin Loop Issues
1. Use a Timeout
One effective way to break the cycle of spin loops is to utilize a timeout with the select()
method. By setting a timeout value, the selector will wait for a fixed time before returning, allowing the thread to yield and lessening CPU consumption.
public void run() {
while (true) {
try {
// Use a timeout of 100 milliseconds
int readyChannels = selector.select(100);
if (readyChannels == 0) {
continue;
}
// Process the selected keys...
} catch (IOException e) {
e.printStackTrace();
}
}
}
2. Use selectNow()
Instead of blocking indefinitely, you may call selectNow()
, which returns immediately without blocking. This approach allows you to perform non-blocking checks on the selector, which can be coupled with additional logic.
public void run() {
while (true) {
try {
int readyChannels = selector.selectNow();
if (readyChannels > 0) {
// Process the selected keys...
}
// Perform other tasks or simply sleep
Thread.sleep(10);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
3. Optimize Channel Registration
Another factor contributing to spin loops could be related to how channels are registered with selectors. It’s essential to manage the registration lifecycle properly by removing channels that no longer need monitoring. This keeps the selector's workload lighter.
public void unregisterChannel(SocketChannel channel) {
if (channel != null && channel.isOpen()) {
try {
channel.keyFor(selector).cancel();
channel.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
The above snippet efficiently removes a channel from monitoring, reducing the load on the selector and consequently mitigating spin loop tendencies.
4. Consider Asynchronous I/O (AIO)
If your application requirements permit, consider using Java's Asynchronous I/O (AIO) capabilities. AIO can be more efficient for certain types of I/O operations, freeing threads from waiting on blocking calls.
Closing the Chapter
Spin loops can severely impact the performance of applications utilizing Java NIO selectors. By employing strategies such as using a timeout, calling selectNow()
, optimizing channel registrations, and even considering asynchronous I/O, developers can significantly reduce or eliminate the detrimental effects of spin loops.
For a more profound understanding of Java NIO, you can check out Java NIO: The New Input/Output.
Remember, every application has unique needs, so while these techniques are broadly applicable, always tailor solutions to your specific scenarios.
Further Reading
- Java NIO Tutorial
- Understanding Java's Selector API
By applying these practices, you can ensure your Java NIO applications remain performant and responsive, effectively overcoming spin loop issues. Happy coding!