Common Pitfalls in Capturing Mutable Variables in Java Lambdas
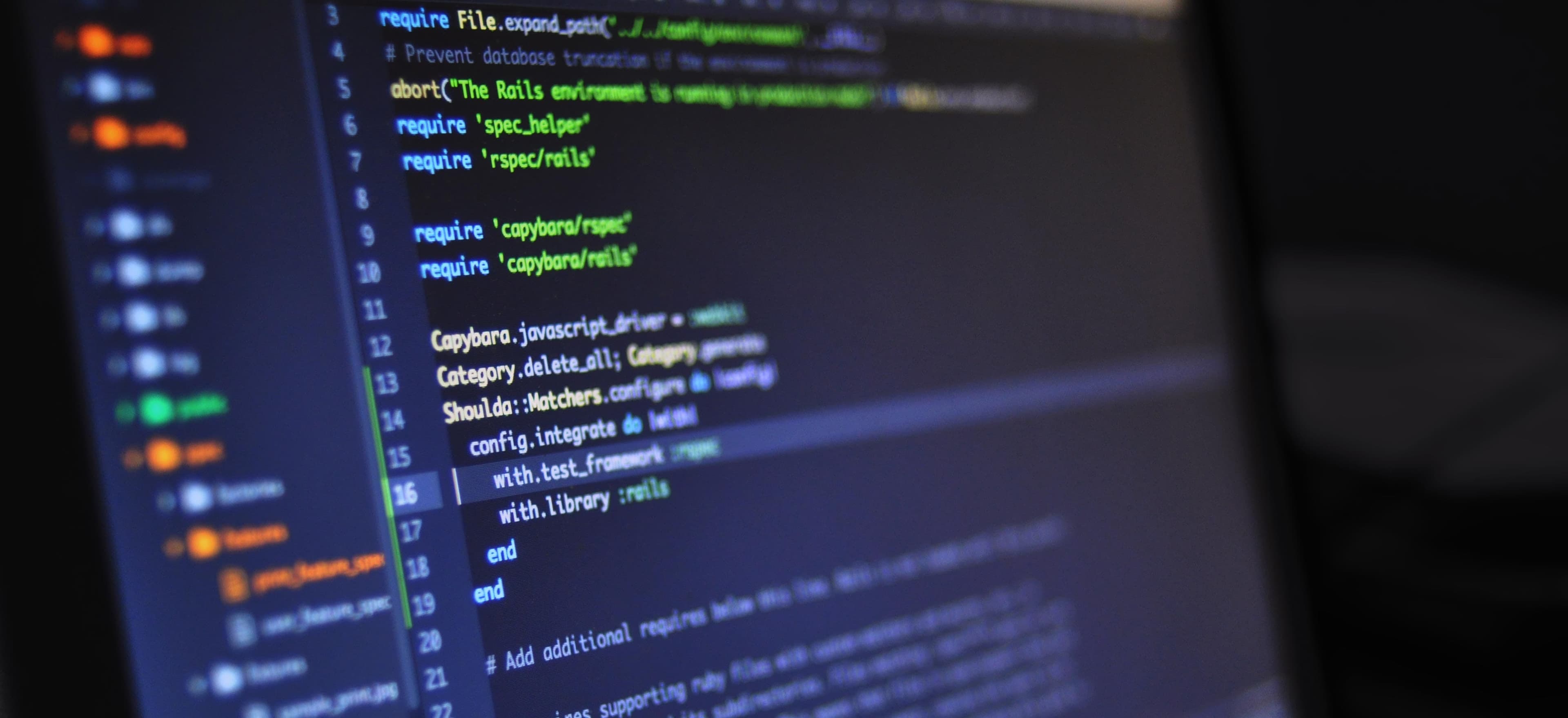
- Published on
Common Pitfalls in Capturing Mutable Variables in Java Lambdas
Java introduced lambdas in Java 8, a feature that significantly improved the expressiveness and conciseness of the language. However, the power of lambda expressions comes with its own set of complexities, particularly when dealing with mutable variables. In this blog, we will explore common pitfalls associated with capturing mutable variables in Java lambdas, ensuring that you understand the underlying mechanics and can leverage lambdas safely and effectively.
Understanding Lambdas and Variable Scope
Before diving into the pitfalls, it is vital to grasp how lambdas capture variables. In Java, lambdas can capture variables that are effectively final or final in nature. This behavior means you can use local variables inside a lambda, but you cannot modify them after they have been captured.
Here’s a simple lambda that captures a local variable:
public class LambdaExample {
public static void main(String[] args) {
final int x = 10; // effectively final
Runnable runnable = () -> System.out.println(x);
runnable.run(); // Prints: 10
}
}
In this case, x
is declared as final, which allows the lambda to access its value without any issues.
While this is straightforward, mutable variables present challenges. Let’s look at some common pitfalls when lambdas capture mutable variables.
Pitfall 1: Modifying Mutable Variables
The first pitfall involves modifying a mutable variable within a lambda. Since Java captures a snapshot of the variable's state when the lambda is created, subsequent modifications can lead to unexpected results.
Consider the following code snippet:
import java.util.ArrayList;
import java.util.List;
public class MutableVariableExample {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
int[] sum = {0}; // mutable variable
Runnable runnable = () -> {
for (int i = 0; i < 5; i++) {
sum[0] += i; // modifying mutable variable
list.add(i);
}
};
runnable.run();
System.out.println("Sum: " + sum[0]); // Prints: Sum: 10
System.out.println("List: " + list); // Prints: List: [0, 1, 2, 3, 4]
}
}
While the code compiles and runs without errors, relying on mutable state can lead to hard-to-track bugs, especially in multi-threaded environments. Therefore, it's generally best to avoid modifying variables that are referred to within a lambda.
Why This Is Problematic
When using mutable variables, if the state is altered by other threads simultaneous to the lambda execution, it could lead to unpredictable behavior. This issue arises primarily due to how lambdas are treated by the Java compiler. They live beyond the scope of the methods that create them, which means their outer variables can be modified externally while they are executing.
Pitfall 2: Shadowing Variables
Another common pitfall is variable shadowing, which happens when a lambda captures a variable with the same name as a variable in an outer scope. This can lead to confusion about which variable you're referring to.
Consider this example:
public class ShadowingExample {
public static void main(String[] args) {
int number = 5;
Runnable runnable = () -> {
int number = 10; // This variable shadows the outer variable
System.out.println("Inner number: " + number); // Prints: Inner number: 10
};
runnable.run();
System.out.println("Outer number: " + number); // Prints: Outer number: 5
}
}
Why It Matters
In this example, the inner variable number
shadows the outer variable. This can lead to bugs if developers are not careful about which variable they're modifying. Consequently, it is crucial to use unique variable names inside lambdas to avoid shadowing and ensure clarity.
Pitfall 3: Variable Capture in Loops
Capturing loop variables in Java lambdas can lead to unexpected results as well. This typically occurs when the lambda is created within a loop, and you expect it to capture the current value of the variable at each iteration.
import java.util.ArrayList;
import java.util.List;
public class LoopCaptureExample {
public static void main(String[] args) {
List<Runnable> runnables = new ArrayList<>();
for (int i = 0; i < 5; i++) {
Runnable runnable = () -> System.out.println(i); // Capturing loop variable
runnables.add(runnable);
}
for (Runnable r : runnables) {
r.run(); // Prints: 5 five times
}
}
}
The Solution
In the above code, the lambda captures the variable i
, but since i
is modified after it is captured, all the lambdas in the list print 5
instead of the expected values from 0
to 4
. To fix this, you can create a final copy of the loop variable:
for (int i = 0; i < 5; i++) {
final int j = i; // Capture a final variable
Runnable runnable = () -> System.out.println(j);
runnables.add(runnable);
}
By declaring j
as final
, each lambda now captures its own value of i
, yielding the expected results.
Best Practices
-
Avoid Mutable State in Lambdas: Whenever possible, prefer immutable objects or use local final variables instead of mutable ones.
-
Use Unique Variable Names: This minimizes the chance of shadowing and improves readability.
-
Capture Loop Variables Safely: If you need to capture a loop variable, create a new final variable within the loop to avoid capturing the variable itself.
-
Consider Thread-Safety: Be aware of the context in which your lambdas are executed, especially if you are using them in a multi-threaded environment.
Final Thoughts
Lambdas in Java provide powerful capabilities for functional programming, enabling cleaner and more efficient code. However, it's crucial to understand how they capture variables—particularly mutable ones—to avoid potential pitfalls.
For deeply diving into functional programming in Java, consider checking the official Java documentation on Lambda Expressions or exploring Java's Functional Interfaces for a comprehensive understanding.
Now that you are aware of these common pitfalls, you can write more effective and less error-prone Java code using lambdas. Happy coding!
Checkout our other articles