Understanding Java String Interning: Common Pitfalls
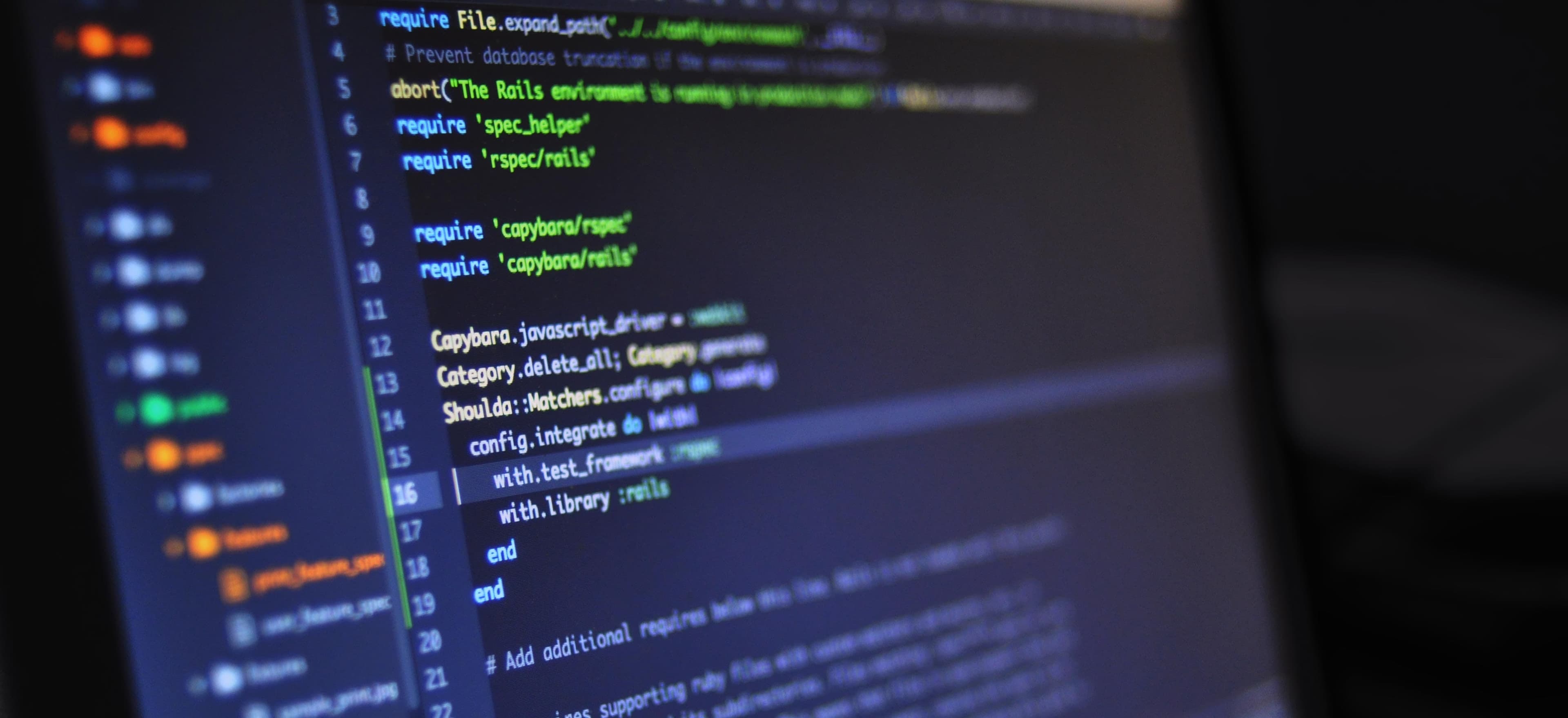
- Published on
Understanding Java String Interning: Common Pitfalls
Java developers often encounter strings. Whether they are manipulating user input or configuring output, strings are unavoidable. One feature in Java designed to optimize memory usage with strings is string interning. In this post, we will delve into the concept of string interning, examine common pitfalls, and discover best practices.
What is String Interning?
String interning is a method of storing only one copy of each distinct string value, which must be immutable. This means that any two String objects that have the same value will reference the same memory location. This can lead to significant memory savings, especially in applications that utilize many identical strings.
Why Intern Strings?
-
Memory Efficiency: When the same strings are used multiple times, interning saves memory usage by ensuring that identical strings refer to the same memory location.
-
Performance Optimization: Interning can lead to faster comparisons since strings are compared using reference equality (==) instead of content equality (.equals).
-
Cache Benefits: The JVM maintains a constant pool of string literals. When you intern a string, it retrieves the string from this pool, ensuring fast access.
The Intern Method
Interning can be explicitly controlled using the intern()
method. Here’s an example:
public class StringInternExample {
public static void main(String[] args) {
String str1 = new String("hello");
String str2 = new String("hello");
// Without interning, str1 and str2 reference different objects
System.out.println(str1 == str2); // Output: false
// Intern the strings
String internedStr1 = str1.intern();
String internedStr2 = str2.intern();
// Both internedStr1 and internedStr2 reference the same object now
System.out.println(internedStr1 == internedStr2); // Output: true
}
}
How Interning Works
Interning takes place when a string is defined as a string literal or when the intern()
method is used. When a string is created, the JVM first checks the pool to see if an identical string already exists. If it does, the method returns a reference to the pooled instance; otherwise, it adds the new string to the pool.
Common Pitfalls When Using String Interning
While beneficial, string interning can lead to misunderstandings and performance issues when not used correctly. Let’s explore some common pitfalls.
1. Unintended Memory Usage
Interning every string can actually cause the opposite effect: excessive memory usage. When interned strings are no longer needed, they remain in the pool as long as the JVM is running. This can lead to increased memory consumption, especially in applications that heavily use dynamic string creation like GUI applications or web servers.
Think twice before interning strings, particularly those that are not reused often.
public class ExcessiveInterning {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
String temp = new String("Example String " + i);
temp.intern(); // This will continuously fill the string pool
}
// Memory consumption will skyrocket here.
}
}
2. Equality Mistakes
Utilizing ==
to compare interned strings can result in unexpected behavior if the strings are not interned. For example, strings taken from a database or an external source may not be interned by default.
public class EqualityMistake {
public static void main(String[] args) {
String dbValue = getStringFromDatabase(); // Suppose this returns "test"
String hardCodedValue = "test";
// This will return false because dbValue is not interned.
System.out.println(dbValue == hardCodedValue);
}
}
To ensure equality, use .equals()
for string content comparison.
3. Performance Overheads
While interning can improve performance through faster string comparison, the overhead of interning itself (i.e., the memory lookup) can add unnecessary time overhead when done repeatedly in a loop or a frequently called method:
public class PerformanceOverhead {
public static void main(String[] args) {
for (int i = 0; i < 100000; i++) {
String internedString = new String("Sample").intern(); // Overhead increases in frequent calls
}
}
}
4. Unintended Shares
Interning strings means you’re sharing the same instance. Any changes made through one reference will affect all others.
public class UnintendedShares {
public static void main(String[] args) {
String str1 = "hello";
String str2 = str1.intern();
// Using a mutable operation on `str2`, although it's immutable, you might think same as `str1`
System.out.println(str1.toUpperCase() == str2.toUpperCase()); // Output: true
}
}
Best Practices for String Interning
To avoid these pitfalls and utilize string interning effectively, consider the following best practices:
-
Intern Strings Judiciously: Only intern strings that are reused frequently. Avoid excessive interning for unique strings.
-
Use
.equals()
for Comparison: Prioritize content comparison over reference comparison, especially when dealing with user-generated or external input. -
Avoid Overuse in Loops: Repeated interning inside loops can be inefficient and may lead to performance hits.
-
Garbage Collection Awareness: Remember that once a string is interned, it remains in memory until the JVM exits. Monitor your application for potential memory increases when using interning extensively.
-
StringBuilder for Pre-Interning Operations: When constructing strings dynamically, consider using
StringBuilder
before converting to a single interned string.
public class EfficientStringConstruction {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 100; i++) {
sb.append("String " + i);
}
String finalString = sb.toString().intern(); // Intern the final output only
}
}
The Closing Argument
String interning can be a powerful tool for optimizing memory usage and performance in Java applications. However, as we have seen, it comes with its own set of pitfalls. Awareness of these issues can lead to better coding practices and ultimately, more efficient applications.
By adhering to best practices, you can reap the benefits of string interning while avoiding the common pitfalls. Always remember: while performance is essential, so is writing clear, maintainable code.
For further reading on Java performance optimizations and string handling, check out these resources:
- Official Java Documentation
- Java String Interning: Everything You Need To Know
Feel free to share your thoughts or experiences with string interning in the comments section below! Happy coding!