Mastering Efficient Strategies for Large File Processing
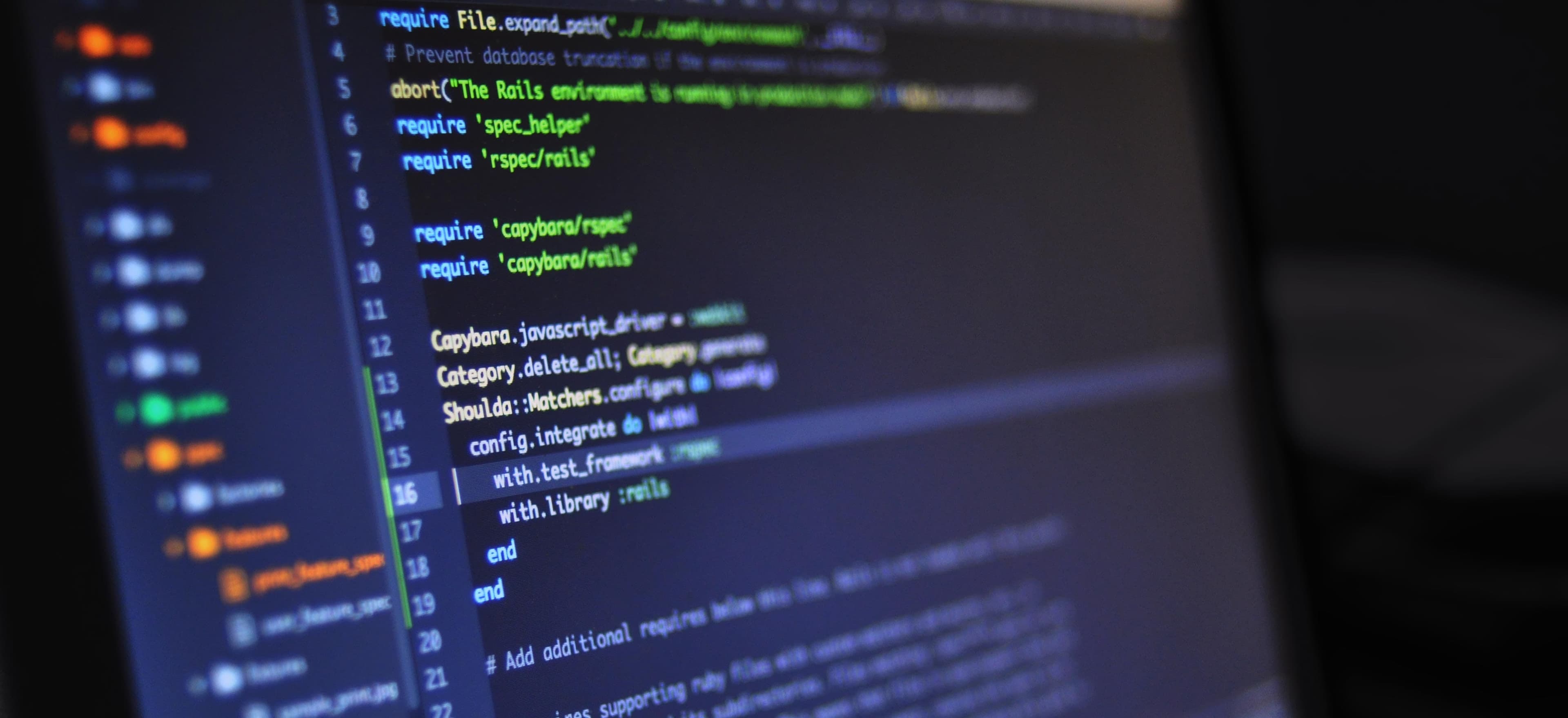
- Published on
Mastering Efficient Strategies for Large File Processing in Java
Handling large files is an essential skill for any Java developer. Whether you're processing logs, managing data files, or performing batch jobs, efficiency often dictates the success of your project. In this blog post, we will explore various strategies for processing large files effectively in Java, including memory management, file reading techniques, and optimization practices.
Understanding the Challenges
Large files pose numerous challenges, including:
- Memory Consumption: Loading an entire file into memory can lead to
OutOfMemoryError
. - I/O Performance: Disk I/O can be a bottleneck, especially with traditional file-reading methods.
- Speed: Inefficient algorithms can significantly slow down processing times.
Before diving into strategies, let's stand on a solid foundation of tools and techniques.
Choosing the Right Tools
Java provides several classes that help in file processing, such as File
, BufferedReader
, FileInputStream
, along with NIO (Non-blocking I/O) for improved performance. Using the right tools is critical for efficient processing.
Scenario: Reading a Large Text File
Imagine we have a large text file containing log data. Here’s a simple and efficient way to read the file line by line using BufferedReader
.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class LargeFileReader {
public static void main(String[] args) {
String filePath = "path/to/your/largefile.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
processLine(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
private static void processLine(String line) {
// Process each line (e.g., parsing the log entry)
}
}
Why Use BufferedReader?
-
Buffered Reading:
BufferedReader
uses an internal buffer, allowing it to read larger chunks of data at once from disk. This reduces the number of I/O operations. -
Efficiency: By reading one line at a time, we minimize memory usage, making it scalable for large files.
Utilizing Java NIO for File Processing
Java NIO, introduced in Java 7, provides a more flexible and efficient file I/O mechanism. Use the Files
and Path
classes for straightforward and optimized file handling.
Here’s how to read a large file using NIO.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class LargeFileNIO {
public static void main(String[] args) {
Path path = Paths.get("path/to/your/largefile.txt");
try {
List<String> lines = Files.readAllLines(path);
for (String line : lines) {
processLine(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
private static void processLine(String line) {
// Process each line (e.g., parsing the log entry)
}
}
When to Use Java NIO?
-
Read All Lines:
Files.readAllLines()
is simple but not for ultra-large files that exceed memory limits. Consider using streaming methods for those cases. -
Asynchronous I/O: NIO allows for asynchronous file processing. This can lead to performance improvements, especially in applications that handle many file operations.
Implementing Streaming for Extremely Large Files
For very large files, leveraging streaming to avoid loading the entire file into memory is optimal. The following example demonstrates how to utilize Files.lines()
for streaming file content:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class StreamingFileReader {
public static void main(String[] args) {
Path path = Paths.get("path/to/your/largefile.txt");
try (Stream<String> lines = Files.lines(path)) {
lines.forEach(line -> processLine(line));
} catch (IOException e) {
e.printStackTrace();
}
}
private static void processLine(String line) {
// Process each line (e.g., parsing the log entry)
}
}
Benefits of File Streaming
-
Memory-Saving: Only a small portion of the file is loaded at any given time, significantly lowering memory usage.
-
Performance: Classic I/O operations are often slower; however, streaming can improve I/O performance as data can be processed almost immediately as it is read.
Optimizing Through Parallel Processing
When working with large files, you may benefit from parallel processing. By leveraging the parallelStream()
method, you can effectively utilize multiple CPU cores.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class ParallelFileProcessing {
public static void main(String[] args) {
Path path = Paths.get("path/to/your/largefile.txt");
try (Stream<String> lines = Files.lines(path)) {
lines.parallel().forEach(line -> processLine(line));
} catch (IOException e) {
e.printStackTrace();
}
}
private static void processLine(String line) {
// Process each line (e.g., parsing the log entry)
}
}
When to Use Parallel Processing?
-
High CPU Demand: If the processing of each line is CPU-intensive, parallel processing can significantly speed up the complete operation.
-
Independent Tasks: Ensure that each task (processing a line) is independent to avoid race conditions and ensure thread safety.
Closing Remarks
Processing large files in Java requires a systematic approach and understanding of your specific needs. From buffered reading to the powerful capabilities of Java NIO, choosing the right tool can make a significant difference.
For additional reading on advanced file handling in Java, check out Java’s NIO Package and Java’s File I/O.
The chosen strategy should always depend on your application requirements and expected performance outcomes. Start implementing these techniques in your projects to create scalable, efficient, and robust file-processing applications. Happy coding!