Overcoming Java Serialization Compatibility Issues
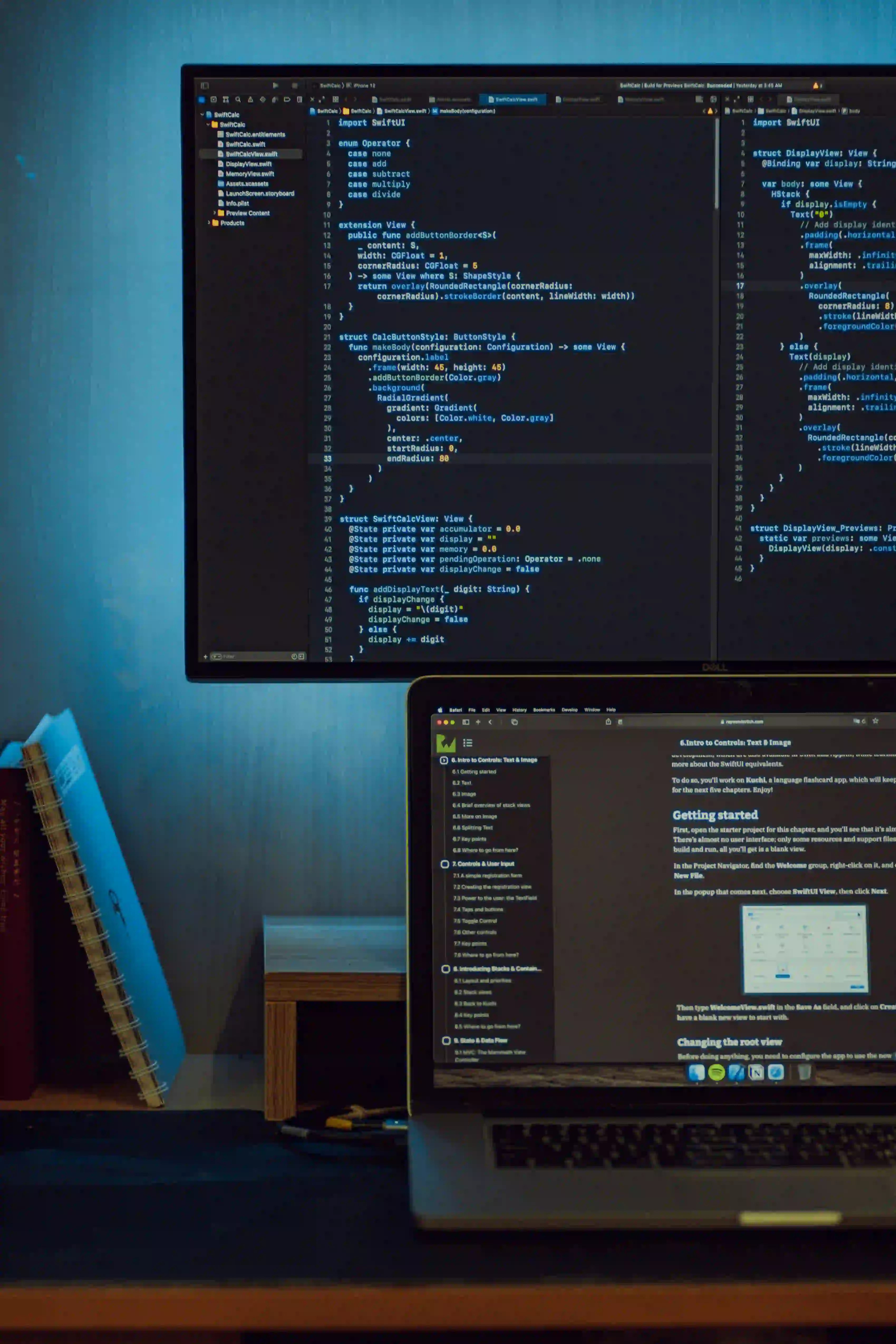
Overcoming Java Serialization Compatibility Issues
Serialization is a crucial mechanism in Java that enables the conversion of an object into a byte stream, allowing the object to be easily saved to a file or transmitted over a network. However, when dealing with the evolving nature of an application, serialization can lead to compatibility issues that challenge developers. In this blog post, we will delve into the intricacies of Java serialization, discuss common compatibility issues that arise, and explore strategies to mitigate these problems.
Understanding Java Serialization
Serialization in Java is achieved using the Serializable
interface. When an object is serialized, its current state is converted into a byte stream, which can then be stored or sent.
import java.io.Serializable;
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String name;
private int age;
// Constructors, getters, and setters
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
In this example, the User
class implements Serializable
, and we define a serialVersionUID
to uniquely identify the version of the class. The serialVersionUID
is crucial for maintaining serialization compatibility across different versions of the class.
Common Serialization Compatibility Issues
-
Changing Class Structure: Modifying fields (adding, removing, or renaming) in a serialized class without updating the
serialVersionUID
can lead toInvalidClassException
. -
Inheritance Changes: Inheritance can add complexity, especially when subclassing. Changes in the base class reflected in the subclass may lead to incompatibility.
-
Non-Serializable Fields: If a
Serializable
class includes fields that are not serializable, it will throw aNotSerializableException
. -
Default Serial Version UID: Avoid using the default
serialVersionUID
as it changes with each class modification, which can lead to differences in serialization between different versions of the class.
Strategies to Overcome Compatibility Issues
1. Use a Stable serialVersionUID
Always declare a serialVersionUID
to control the serialization process. Updating this ID whenever you change the class structure indicates to the Java Virtual Machine (JVM) to use a different serialization mechanism.
private static final long serialVersionUID = 2L; // Increment when class changes
2. Implement Read-Object and Write-Object Methods
You can handle complex serialization scenarios by implementing custom readObject
and writeObject
methods. This gives you control over how fields are serialized and deserialized.
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
out.defaultWriteObject(); // Serialize default fields
out.writeInt(extraField); // Manually serialize extra fields
}
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject(); // Deserialize default fields
extraField = in.readInt(); // Manually deserialize extra fields
}
3. Use transient
Fields
Fields marked as transient
are ignored during serialization. This is useful for non-serializable fields or fields whose state should not be serialized.
private transient String tempData; // This field will not be serialized
4. Versioned Classes
Create versioned classes to handle changes over time. This involves creating different serializable classes for different versions of your data.
public class UserV1 implements Serializable {
// Version 1 fields
}
public class UserV2 implements Serializable {
// New fields for version 2
}
5. Use Externalizable Interface
If you need full control over serialization, consider using the Externalizable
interface, allowing you to define your entire serialization mechanism.
public class User implements Externalizable {
public void writeExternal(ObjectOutput out) throws IOException {
out.writeUTF(name);
out.writeInt(age);
}
public void readExternal(ObjectInput in) throws IOException {
name = in.readUTF();
age = in.readInt();
}
}
6. Maintain a Serialization Compatibility Tribute
When modifying classes meant for serialization, maintain a comprehensive list of changes. This can be beneficial for new developers joining the project or during code reviews.
The Last Word
While Java serialization is a powerful feature, it is essential to handle it with care to avoid compatibility issues. Understanding the common pitfalls and implementing best practices such as declaring a stable serialVersionUID
, using transient
fields, and controlling serialization with custom methods can save developers from significant headaches.
For more in-depth readings on Java serialization, consider referencing the official Java Serialization documentation or exploring open-source libraries that handle serialization, such as Kryo.
Staying vigilant about backward compatibility when working with serialized data will not only improve your application’s resilience but also enhance the overall user experience. Implementing these strategies will empower you to navigate the complexities of Java serialization with confidence, making your applications robust and reliable.