How to Fix "Could Not Reserve Enough Space for Object Heap"
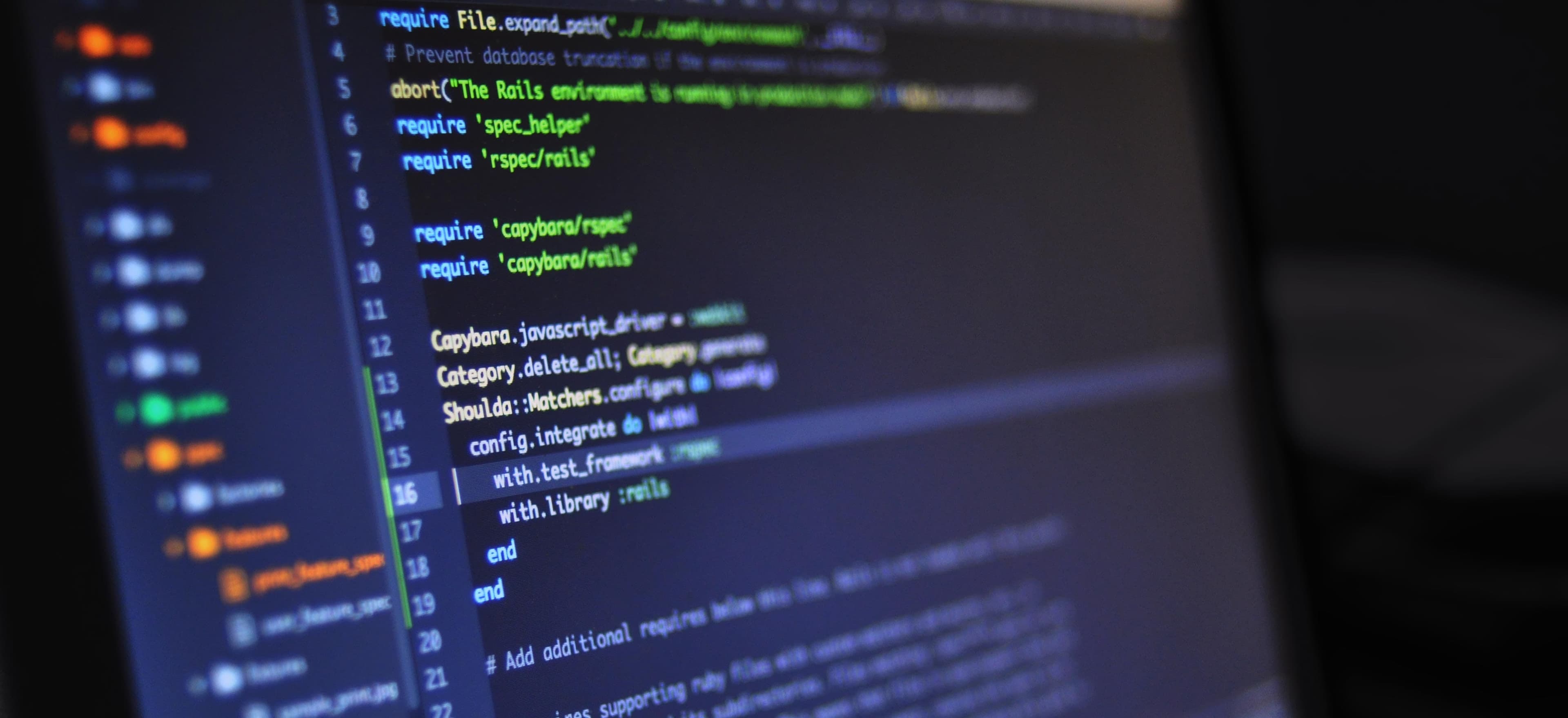
- Published on
How to Fix "Could Not Reserve Enough Space for Object Heap" in Java
If you are a Java developer, you might have encountered the error message "Could not reserve enough space for object heap." This warning can be quite frustrating and can hinder your application from running efficiently. In this post, we'll dive deep into understanding this error and explore various methods to resolve it.
Understanding the Error
Before we jump into solutions, let's dissect the error itself. This message indicates that the Java Virtual Machine (JVM) was unable to allocate the memory it needs for the Object Heap. The Object Heap is used for dynamic memory allocation of Java objects. If the JVM cannot get enough memory, it simply won't run.
Why Does It Happen?
- Insufficient Memory: Your system may not have enough physical RAM to accommodate the requested heap size.
- Large Heap Size Configuration: The specified heap size might be set too high, attempting to use more memory than the system can provide.
- System Limits: Operating system constraints can also limit the maximum size that can be allocated, especially on 32-bit systems.
Now that we have a grasp of the problem, let's explore some solutions.
Solution 1: Adjust the Heap Size
One of the most straightforward ways to troubleshoot this issue is to adjust the heap size using the -Xms
and -Xmx
parameters.
Example:
java -Xms256m -Xmx1024m -jar YourApplication.jar
Explanation:
-Xms256m
: Sets the initial heap size to 256 MB.-Xmx1024m
: Sets the maximum heap size to 1024 MB.
Why Adjust These Parameters?
Having an initial heap size (-Xms
) that is too large can lead to allocation failures if the system doesn’t have sufficient resources. Conversely, setting the maximum heap size (-Xmx
) allows you to cap the memory used by your Java application, preventing it from consuming too much system memory.
Solution 2: Check System Memory
Sometimes, the issue is straightforward: your system may be low on RAM.
- Check Available Memory: Ensure that you have enough memory available on your machine.
- Close Unnecessary Applications: Free up memory by closing applications running in the background.
How to Check Available Memory on Linux:
free -m
This command will show you the free and used memory in megabytes.
For Windows Users
You can check memory availability via the Task Manager. Simply right-click on the taskbar, click on Task Manager, and check the Performance tab.
Solution 3: Use a 64-bit JVM
If you are currently using a 32-bit JVM, switching to a 64-bit JVM can provide a significant boost in available heap space.
Why A 64-bit JVM?
A 64-bit Java Virtual Machine allows your application to utilize more memory than a 32-bit JVM, which is typically limited to around 4 GB of heap size. By using a 64-bit JVM, you can bump this limit significantly, depending on your system's RAM.
How to Switch to a 64-bit JVM
To download a 64-bit JDK, visit the Oracle JDK Download page or use OpenJDK's Adoptium for a free alternative.
After installation, make sure your system's PATH variable points to the 64-bit version of the JDK.
Solution 4: Monitor for System Limits
On some operating systems, especially UNIX-based ones, there are limits on how much memory a single process can use, known as 'ulimits'. Use the following command to check current limits:
ulimit -a
Increasing Memory Limits
If your process reaches the maximum limit, you may need to increase it. This can usually be done in your shell profile (like .bashrc
or .bash_profile
) or for the current session:
ulimit -m unlimited
ulimit -v unlimited
Make sure to consult your system administrator, as certain limits may be enforced for a reason.
Solution 5: Clean Up Your Code
Memory leaks in your application can consume heap space unnecessarily. Use tools like VisualVM or Eclipse Memory Analyzer to identify and fix memory leaks.
Example: Using VisualVM
- Install VisualVM: Bundled with JDK or available separately.
- Connect: Attach VisualVM to your running Java application.
- Analyze Memory: Use the Heap Dump and Sampler features to identify the memory usage patterns.
This kind of monitoring helps in understanding not just the heap memory consumption but also optimizes your code for better performance.
Bringing It All Together
Errors like "Could not reserve enough space for object heap" can disrupt your development process, but with proper understanding and a systematic approach, they can be resolved effectively.
Summary of Solutions:
- Adjust JVM Heap Size with
-Xms
and-Xmx
. - Check available system memory.
- Switch to a 64-bit JVM for larger heap sizes.
- Monitor system limits with
ulimit
. - Clean up your code to avoid memory leaks.
By understanding these aspects and solutions, you can ensure a smoother performance for your Java applications. For further reading on Java memory management, consider checking out this Oracle tutorial.
With these strategies in place, your Java applications should run more seamlessly, free from the hurdles of memory allocation issues.
Checkout our other articles