Mastering Character Encoding: Avoiding Unicode Confusion in Java
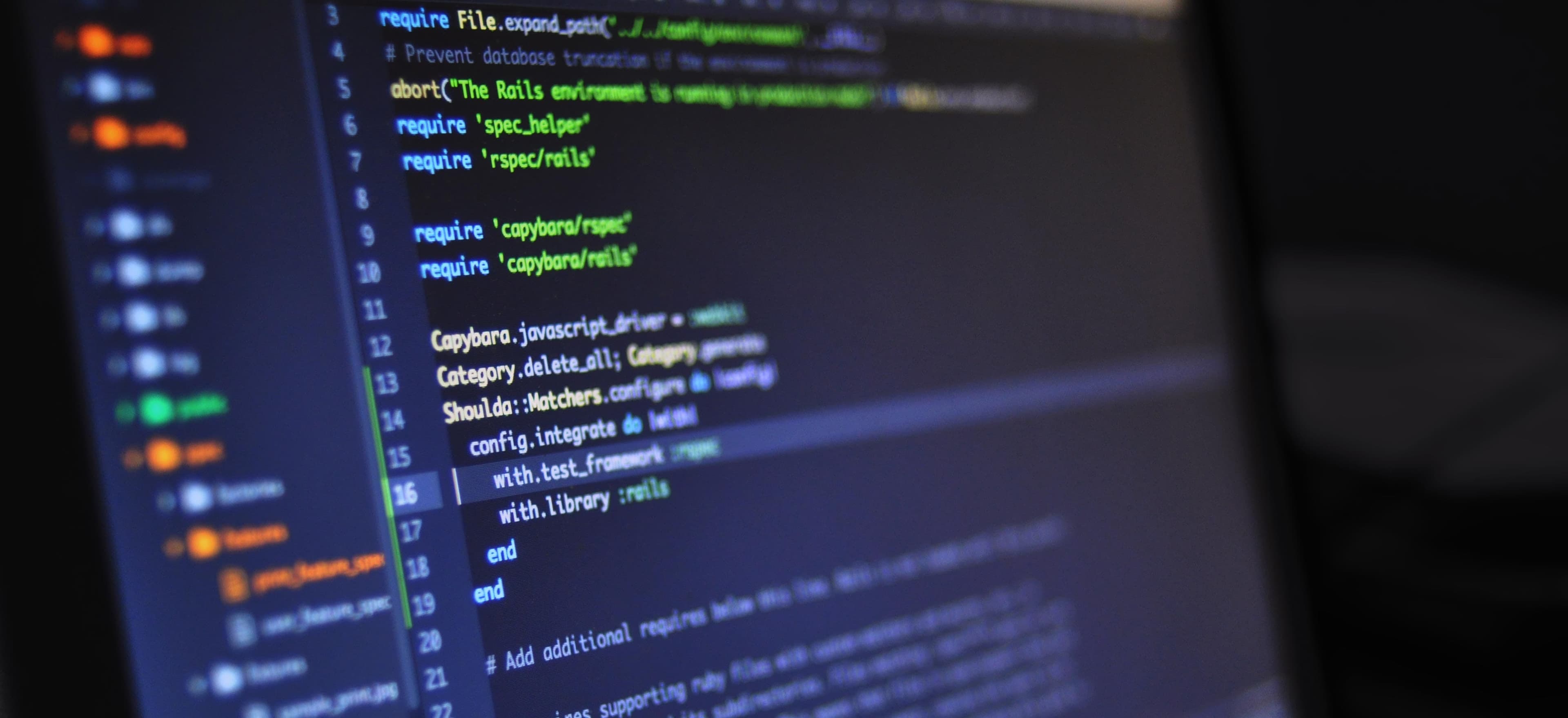
- Published on
Mastering Character Encoding: Avoiding Unicode Confusion in Java
Character encoding is a critical concept in programming, especially for developers working with internationalization or handling text data. This blog post will dive deep into the world of character encoding in Java, with a particular focus on Unicode. By the end, you will gain a clear understanding of how to avoid potential pitfalls associated with encoding.
Understanding Character Encoding
Character encoding translates characters into a format that can be easily processed by computers. Unicode is a universal character encoding standard that encompasses a variety of characters from different languages in a single encoding scheme. Java, with its inherent support for Unicode, simplifies handling international text data.
Why Unicode?
Before diving deeper, let's address the significance of Unicode:
- Uniformity: It standardizes the representation of characters across various platforms and devices.
- Support for Internationalization: Handling multilingual data becomes a breeze; you can support characters from languages like Mandarin, Arabic, and more.
- Flexibility: Unicode can represent over a million characters, accommodating emojis, ancient scripts, and symbols.
Java's Character Encoding
In Java, the char
type is 16 bits, meaning it can directly work with Unicode characters. However, handling character encoding becomes critical when you’re reading from or writing to external sources such as files or network sockets. If you don’t manage encoding correctly, you can end up with garbled text or loss of data.
The Basics of Encoding in Java
To better understand encoding in Java, it's essential to focus on key classes:
- String: The main class for manipulating text.
- InputStreamReader: Bridges byte streams to character streams.
- OutputStreamWriter: Bridges character streams to byte streams.
Example: Reading a File with Correct Encoding
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.InputStreamReader;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
public class FileReadingExample {
public static void main(String[] args) {
String path = "example.txt";
try (BufferedReader reader = new BufferedReader(
new InputStreamReader(new FileReader(path, StandardCharsets.UTF_8)))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
In the above code:
- We use
BufferedReader
withInputStreamReader
to read data from the file. StandardCharsets.UTF_8
specifies that we want to read the file as UTF-8 encoded text.
Why use UTF-8? It’s the most widely used encoding on the web, supporting all characters in the Unicode Standard.
Common Pitfalls in Character Encoding
Knowing the potential issues can save you hours of debugging later. Here are common pitfalls developers encounter:
1. Mismatch Between File Encoding and Java
If a file is saved in a different encoding than UTF-8 but you assume it’s in UTF-8, you might see gibberish characters when reading it. It’s vital to know the encoding of your source data.
2. Databases and Character Encoding
When interacting with databases, ensure consistent character encoding. A mismatch can result in strange characters when you retrieve data.
Example: Connecting to a Database with UTF-8
Ensure your JDBC connection string specifies UTF-8:
String url = "jdbc:mysql://localhost:3306/mydb?useUnicode=true&characterEncoding=UTF-8";
Connection connection = DriverManager.getConnection(url, "user", "password");
3. Improper Use of String Conversion
Be cautious when converting between byte arrays and Strings. It’s important to specify the encoding explicitly to avoid confusion:
String original = "Hello, Unicode!";
byte[] bytes = original.getBytes(StandardCharsets.UTF_8);
String decoded = new String(bytes, StandardCharsets.UTF_8);
Commentary
The code above works correctly, ensuring we use UTF-8 throughout the encoding and decoding process. If we omit specifying the character set, it could lead to unpredictable results.
Best Practices for Character Encoding in Java
- Always Specify Encoding: Whether reading or writing files, always specify the charset.
- Standardize Encoding Across Applications: Ensure consistency in source files, database connections, and APIs.
- Use Unicode-Compatible Libraries: Ensure any libraries you use also adhere to Unicode standards.
- Perform Encoding Checks: Validate the encodings especially when reading data from external sources.
Additional Resources
For further reading on character encoding, you might explore:
The Bottom Line
Mastering character encoding is vital for any developer working with text data, especially in a globalized environment. With Java’s robust support for Unicode, avoiding encoding confusion is simplified. Implement best practices, understand potential pitfalls, and embrace the Unicode standard to enhance the quality of your Java applications.
Remember that embracing these practices not only prevents issues but also increases the user's trust in your software's ability to handle their needs—no matter where they come from.
By adhering to the principles discussed, you’ll be equipped to handle any encoding issues that may arise in your Java applications. Happy coding!