Troubleshooting Java NIO Non-Blocking IO Performance Pitfalls
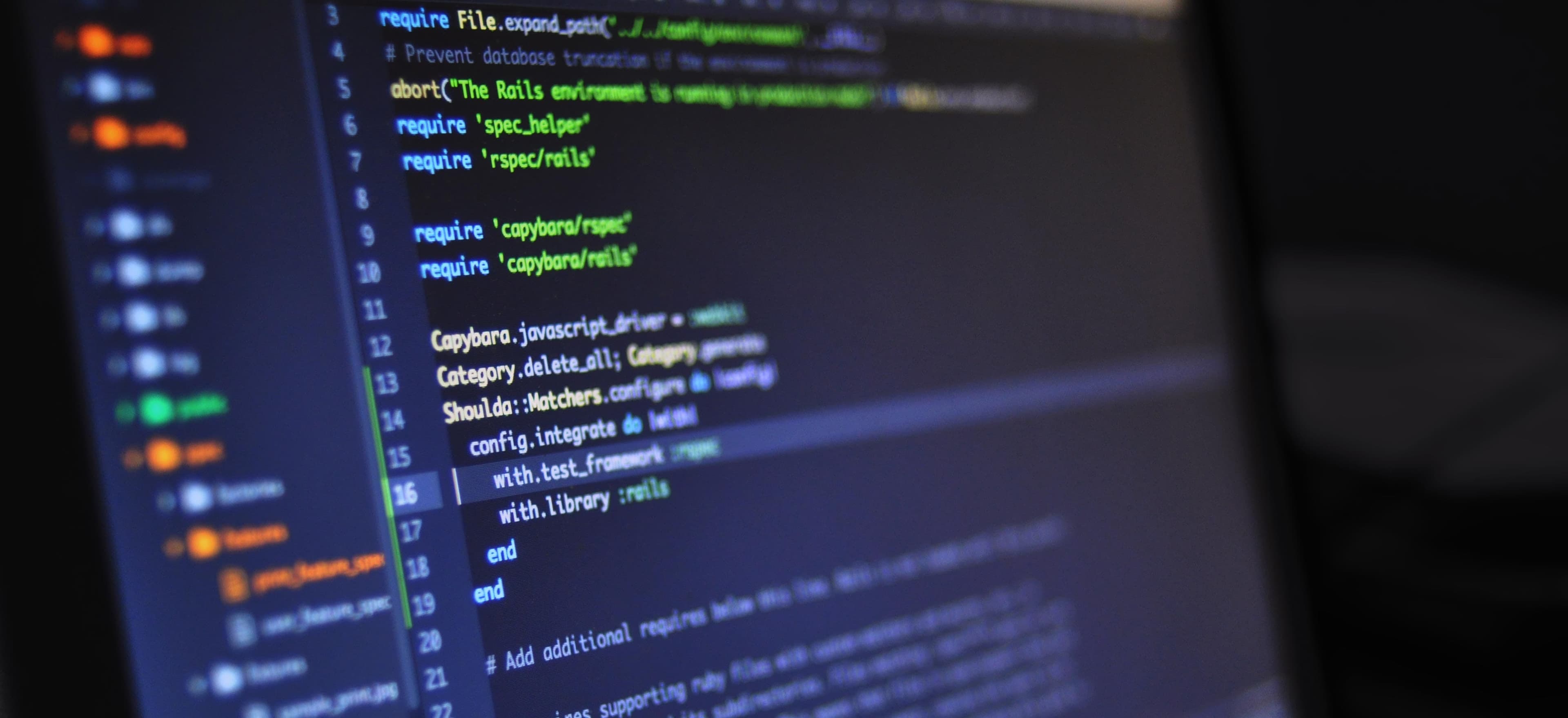
- Published on
Troubleshooting Java NIO Non-Blocking IO Performance Pitfalls
When it comes to high-performance Java applications, the Java New Input/Output (NIO) package often becomes a focal point. The NIO package equips developers with the ability to handle non-blocking IO, which can drastically improve the scalability of applications. However, leveraging NIO to its full potential is not always seamless. In this blog post, we will explore common performance pitfalls when using Java NIO and offer practical solutions for troubleshooting these issues.
Understanding Java NIO
Java NIO is designed primarily to work with buffers, channels, and selectors, making it a powerful addition to the traditional IO capabilities. Here are the core components:
- Buffers: Memory containers for data, capable of reading from and writing to various sources.
- Channels: Abstractions that facilitate the reading and writing of data to files and network sockets.
- Selectors: Enable non-blocking operations by monitoring multiple channels for events.
To illustrate how NIO works, consider the following simple code snippet for reading data from a file using FileChannel:
import java.io.FileInputStream;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.io.IOException;
public class NioFileReader {
public static void main(String[] args) {
try (FileInputStream fis = new FileInputStream("example.txt");
FileChannel fileChannel = fis.getChannel()) {
ByteBuffer buffer = ByteBuffer.allocate(1024);
int bytesRead = fileChannel.read(buffer);
while (bytesRead != -1) {
buffer.flip(); // Prepare the buffer for reading
while (buffer.hasRemaining()) {
System.out.print((char) buffer.get());
}
buffer.clear(); // Clear the buffer for more data
bytesRead = fileChannel.read(buffer);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, we use a FileChannel
to read data from a file. The ByteBuffer
is manipulated using flip()
and clear()
, which are crucial for efficiently handling data flow between reading and writing.
Common Pitfalls in Java NIO Performance
Despite its advantages, using Java NIO can lead to some performance pitfalls. Below, we’ll cover a few common issues and how to troubleshoot them.
1. Improper Buffer Management
One of the biggest performance hits in NIO is from improperly managed buffers. Using a buffer size that is too small can lead to excessive read/write operations, while a buffer that is too large can consume unnecessary memory.
Solution:
Use dynamic buffer sizing based on your application’s workload. Implementing a strategy to determine the optimal buffer size based on benchmarks can make a significant difference.
Example of dynamic buffer size determination:
public class DynamicBufferReader {
private static final int DEFAULT_BUFFER_SIZE = 1024;
public static int determineBufferSize(int dataSize) {
return Math.min(DEFAULT_BUFFER_SIZE, dataSize);
}
}
2. Inefficient Use of Selectors
NIO's Selector
allows you to handle multiple channels using a single thread, but if used improperly, it can become a bottleneck for performance, especially under load.
Solution:
- Make sure to register only the necessary events to avoid unnecessary wake-ups.
- Use the
selectionKey
appropriately in order to minimize unnecessary operations.
Selector selector = Selector.open();
SocketChannel channel = SocketChannel.open();
channel.configureBlocking(false);
channel.register(selector, SelectionKey.OP_READ);
3. Inefficient Error Handling
Poorly handled exceptions or errors in IO operations can lead to dropped connections or resource leaks, severely affecting application performance.
Solution:
Ensure robust error handling and resource management using try-with-resources blocks for IO operations. Always close channels and buffers properly.
4. Excessive Context Switching
A frequent pitfall in multi-threaded Java NIO applications is excessive context switching, which might occur when using multiple threads for small, frequent tasks.
Solution:
Utilize a single-threaded event loop model, adopting approaches such as Akka or leveraging the Reactor pattern. This can reduce context switching, leading to better performance.
Here is an example of an asynchronous IO operation leveraging a single-threaded approach using CompletableFuture:
import java.nio.file.*;
import java.util.concurrent.CompletableFuture;
public class AsyncFileReader {
public CompletableFuture<String> readFileAsync(String path) {
return CompletableFuture.supplyAsync(() -> {
try {
return new String(Files.readAllBytes(Paths.get(path)));
} catch (IOException e) {
throw new RuntimeException(e);
}
});
}
}
5. Monitoring and Resource Management
Failing to monitor your application’s resource usage can lead to unforeseen performance issues down the line.
Solution:
Leverage Java's built-in tools like VisualVM or JConsole to monitor memory usage and CPU load. Pay attention to Garbage Collection (GC) logs, as excessive garbage collection can severely impact the performance of non-blocking IO operations.
Advanced NIO Strategies
For advanced NIO performance tuning, consider using techniques such as:
- Memory Mapping: Use memory-mapped files for high-performance file access. This allows you to treat file contents as part of the memory.
- Direct Byte Buffers: Use direct byte buffers that circumvent the usual garbage collection to reduce latency.
- Asynchronous Channels: From Java 7 onwards, asynchronous channels were introduced. They allow you to enhance concurrency without worrying about the traditional threading model.
Here’s an example using asynchronous file channel:
import java.nio.channels.AsynchronousFileChannel;
import java.nio.file.StandardOpenOption;
import java.nio.ByteBuffer;
import java.nio.file.Paths;
import java.util.concurrent.Future;
public class AsyncFileChannelExample {
public void readFile(String filePath) throws Exception {
AsynchronousFileChannel fileChannel = AsynchronousFileChannel.open(Paths.get(filePath), StandardOpenOption.READ);
ByteBuffer buffer = ByteBuffer.allocate(1024);
Future<Integer> result = fileChannel.read(buffer, 0);
while (!result.isDone()) {
// Do other processing if needed
}
System.out.println("Read " + result.get() + " bytes from file");
}
}
Lessons Learned
Java NIO offers significant performance advantages for high-throughput applications, but its complexity can lead to potential pitfalls. By properly managing buffers, selectors, and resources, as well as implementing efficient error handling, you can troubleshoot and overcome many performance challenges associated with Java NIO.
For a deeper understanding of Java NIO, consider visiting Oracle's Java NIO Overview. You may also explore more about asynchronous programming in Java through the Java 7 NIO.2.
By applying the troubleshooting strategies outlined in this post, you’ll be better equipped to maximize the performance of your non-blocking IO applications and develop more scalable and efficient Java solutions.
Checkout our other articles