Overcoming Java Reflection's Access Restrictions in 2023
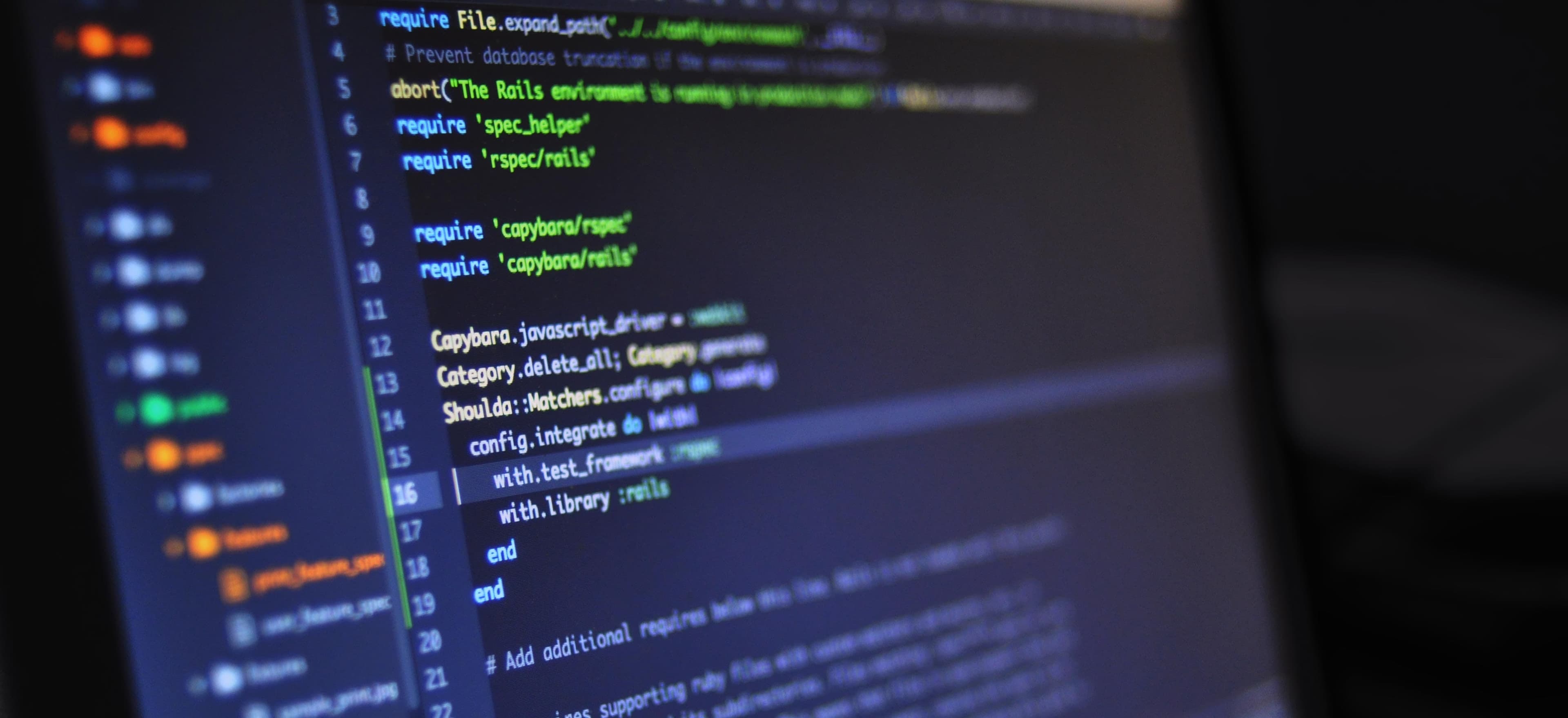
- Published on
Overcoming Java Reflection's Access Restrictions in 2023
Java Reflection is a powerful tool that allows developers to inspect and manipulate classes, methods, fields, and other components at runtime. While it provides significant flexibility, it also comes with access restrictions that can complicate its use. As of 2023, these restrictions have transformed, especially with the introduction of the Java Platform Module System (JPMS) and increased security measures across different Java versions.
In this blog post, we will explore Java Reflection, its access restrictions, and how to overcome these challenges. We'll also make use of relevant code snippets, provide insights into the 'why' behind each example, and offer best practices along the way.
Understanding Java Reflection
Before we dive into access restrictions, let’s clarify what Java Reflection is. With reflection, you can:
- Access Class Information: Inspect a class's methods, constructors, and fields.
- Dynamic Method Invocation: Invoke methods on objects at runtime.
- Manipulate Fields: Read and modify field values dynamically.
Reflection is particularly useful in frameworks (like Spring or Hibernate) where static binding is not feasible due to dynamic nature. However, it brings inherent challenges, especially regarding access to private members.
Access Restrictions in Reflection
Starting from Java 9, the introduction of the module system began to enforce stricter encapsulation. This means reflection is subject to access checks that prevent unauthorized manipulation of private members. Here are some common access control scenarios you may encounter:
- Private fields and methods: You can only access them through reflection if the security manager permits it.
- Module boundaries: Classes in one module cannot reflectively access classes, fields, or methods in another module unless explicitly allowed.
How to Overcome Access Restrictions
While challenging, you can use several methods to bypass these access restrictions when working with Java Reflection. Let’s dive into these solutions, outlining specific code snippets to demonstrate their use cases.
1. Setting Accessible to True
The most straightforward way to bypass access restrictions is to set the accessible
flag to true
using the Field.setAccessible(boolean)
method. This allows reflection to access private members.
Example:
import java.lang.reflect.Field;
public class ReflectionExample {
private String secret = "Hidden Value";
public static void main(String[] args) {
try {
ReflectionExample example = new ReflectionExample();
// Access the private field
Field field = ReflectionExample.class.getDeclaredField("secret");
// Set the field accessible
field.setAccessible(true);
// Get the value of the private field
String value = (String) field.get(example);
System.out.println("Private Field Value: " + value);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Works:
By calling setAccessible(true)
, we override the default access checks and can read or modify private members. However, be cautious as this can lead to security vulnerabilities. You should use this approach only when absolutely necessary.
2. Using AccessibleObject
Class
The AccessibleObject
class provides a way to set fields and methods as accessible. This approach is more generic and can be used when dealing with multiple objects.
Example:
import java.lang.reflect.Method;
public class ReflectionMethodExample {
private void displayMessage() {
System.out.println("Hello, This is a private method!");
}
public static void main(String[] args) {
try {
ReflectionMethodExample example = new ReflectionMethodExample();
Method method = ReflectionMethodExample.class.getDeclaredMethod("displayMessage");
// Setting the method accessible
method.setAccessible(true);
method.invoke(example); // Invoking the private method
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why This Works:
Similar to the previous example, we leverage setAccessible(true)
on the Method object. This allows invocation of methods that would normally be uninvokable due to their private visibility.
3. Bypassing Module Restrictions
In Java 9 and later, if you're working within modular applications, you might find additional barriers when trying to access classes across modules. You can override these restrictions using command-line flags when starting your Java application.
Example Command:
java --add-opens your.module.name/your.package.name=ALL-UNNAMED -cp your-class-path YourMainClass
Why This Works:
The --add-opens
command-line argument lets you specify that your module opens a package for reflection to other unnamed modules (the default behavior for classes not in a named module).
4. Using Unsafe Class (Caution!)
The Unsafe
class provides lower-level access to memory and the JVM, effectively allowing you to bypass more restrictions.
Example:
import sun.misc.Unsafe;
import java.lang.reflect.Field;
public class UnsafeExample {
private int unsafeField = 42;
public static void main(String[] args) throws Exception {
Unsafe unsafe = getUnsafeInstance();
UnsafeExample example = new UnsafeExample();
long offset = unsafe.objectFieldOffset(UnsafeExample.class.getDeclaredField("unsafeField"));
unsafe.putInt(example, offset, 100);
System.out.println("Modified Value: " + example.unsafeField);
}
private static Unsafe getUnsafeInstance() throws Exception {
Field field = Unsafe.class.getDeclaredField("theUnsafe");
field.setAccessible(true);
return (Unsafe) field.get(null);
}
}
Why This Works:
We are accessing the Unsafe
instance to operate directly on memory. However, this method comes with risks. The Unsafe
class is not part of the standard Java API and can lead to unstable applications. Use with extreme caution.
Best Practices to Consider
It's essential to remember that while reflection provides powerful capabilities, it should be used judiciously:
- Performance: Reflection is generally slower than direct access due to type checks and other overhead.
- Security: Excessively using reflection can expose your code to vulnerabilities and breaches.
- Maintainability: Code that heavily relies on reflection may become more challenging to read and maintain over time.
Wrapping Up
Java Reflection offers incredible flexibility for developers, but with power comes responsibility. As of 2023, access restrictions have evolved, particularly due to modular systems and security enhancements.
In this blog post, we examined various strategies for overcoming reflection access restrictions, from using setAccessible(true)
to leveraging command-line flags for module access. Each method comes with its own set of risks and best practices that developers should weigh carefully.
For additional insights on Java Reflection and best practices, consider checking out the official Java Reflection documentation and exploring community resources like Stack Overflow.
Happy coding, and may your reflection journeys be insightful!
Checkout our other articles