Mastering Error Handling in CompletableFuture Explained
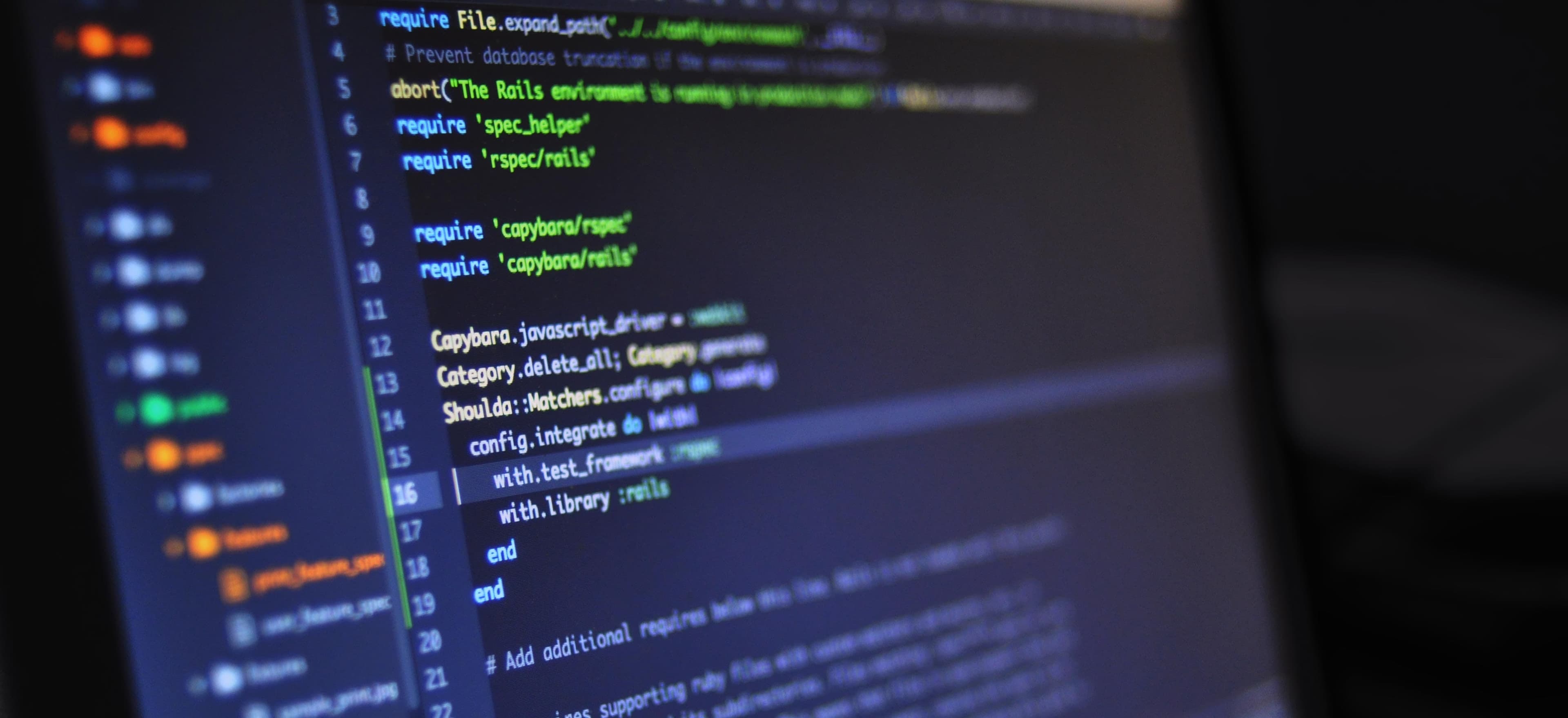
- Published on
Mastering Error Handling in CompletableFuture Explained
Java's CompletableFuture
was introduced in Java 8, providing a robust framework for handling asynchronous programming. One of its most compelling features is error handling, which allows developers to create applications that are not only efficient but also resilient to issues. In this blog post, we will dive deep into the ways you can master error handling when using CompletableFuture
.
Understanding CompletableFuture
CompletableFuture
is a class that represents a future result of an asynchronous computation. It implements the Future
interface and allows you to write non-blocking code. You can create and run multiple tasks in parallel without blocking the main thread, facilitating better resource management.
Why CompletableFuture?
- Non-blocking execution: It allows multiple threads to work efficiently.
- Composability: You can chain multiple asynchronous tasks.
- Error handling: It has built-in methods to handle errors gracefully.
The Basics
Before diving into error handling, let's take a look at a simple example of using CompletableFuture
:
import java.util.concurrent.CompletableFuture;
public class BasicCompletableFuture {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulate a long-running task
sleep(2000);
return "Hello, World!";
});
future.thenAccept(System.out::println);
// Keep the application running to see the result
sleep(3000);
}
private static void sleep(int millis) {
try {
Thread.sleep(millis);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
In this example, we create a CompletableFuture
that asynchronously executes a task. After two seconds, "Hello, World!" is printed.
Error Handling with CompletableFuture
One of the most significant advantages of CompletableFuture
is how it manages exceptions during asynchronous computations. Let’s explore some mechanisms it provides for error handling:
1. Handle with handle
The handle
method allows you to manage both the result and the exception in a single callback. If the computation succeeds, you get the result; if it fails, you receive the exception.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// This will throw an exception
if (true) throw new RuntimeException("Test Exception");
return "Success!";
}).handle((result, ex) -> {
if (ex != null) {
return "Error: " + ex.getMessage(); // Handle the error
}
return result; // Handle the success case
});
future.thenAccept(System.out::println);
Why use handle
? It’s versatile since it allows you to modify both success and error paths in one go.
2. Exception Handling with exceptionally
If you only want to handle exceptions, the exceptionally
method can be your go-to. It’s simpler as it only accepts a single argument—a function that processes the Throwable.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Test Exception");
}).exceptionally(ex -> {
return "Error handled: " + ex.getMessage();
});
future.thenAccept(System.out::println);
Why prefer exceptionally
? It makes your code cleaner when the focus is solely on handling exceptions.
3. Using whenComplete
The whenComplete
method gives you a chance to execute additional logic, regardless of whether the computation succeeded or failed. This is useful for logging or performing cleanup actions.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Simulating a task
if (true) throw new RuntimeException("Test Exception");
return "Success!";
}).whenComplete((result, ex) -> {
if (ex != null) {
System.out.println("Completed with exception: " + ex.getMessage());
} else {
System.out.println("Completed successfully with result: " + result);
}
});
future.join(); // Wait for the completion
Why take advantage of whenComplete
? It is great for non-blocking logging and other follow-up actions.
4. Combining Multiple Futures
When dealing with multiple asynchronous tasks, it’s crucial to handle errors that might arise in any of them effectively.
CompletableFuture<Integer> future1 = CompletableFuture.supplyAsync(() -> {
return 1 / 0; // This will throw an exception
});
CompletableFuture<Integer> future2 = CompletableFuture.supplyAsync(() -> {
return 2;
});
CompletableFuture<Integer> combinedFuture = future1
.thenCombine(future2, Integer::sum)
.exceptionally(ex -> {
System.out.println("An error occurred: " + ex.getMessage());
return 0; // Provide a default value
});
System.out.println(combinedFuture.join()); // Prints 0
Why Handle Errors in Combined Futures?
Handling errors effectively in combined futures ensures that one failing task does not propagate failures to others, maintaining the robustness of your application.
Best Practices for Error Handling in CompletableFuture
- Be Specific with Exceptions: Always log specific exceptions, so you can troubleshoot them in future investigations.
- Chain Wisely: When chaining multiple futures, consider where errors might occur and handle them appropriately at each stage.
- Fallback Actions: Use
exceptionally
orhandle
to provide fallback actions when a computation fails. - Testing: Make sure to write unit tests that simulate exceptions occurring in your asynchronous tasks. Testing ensures your error handling performs correctly under different scenarios.
Wrapping Up
Error handling is a critical aspect of programming, especially in asynchronous environments. Understanding the different methods available in CompletableFuture
allows you to build robust applications that can gracefully manage unexpected issues. Proper implementation ensures that your application remains user-friendly and resilient in production.
For further reading on this topic, check out the Java Documentation on CompletableFuture and explore the various methods and examples that can help cement your understanding of asynchronous programming in Java.
By mastering error handling in CompletableFuture
, you take one step closer to writing efficient, reliable Java applications. Happy coding!
Checkout our other articles